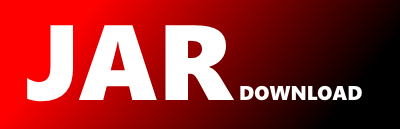
com.pulumi.aws.networkfirewall.FirewallArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.networkfirewall;
import com.pulumi.aws.networkfirewall.inputs.FirewallEncryptionConfigurationArgs;
import com.pulumi.aws.networkfirewall.inputs.FirewallSubnetMappingArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class FirewallArgs extends com.pulumi.resources.ResourceArgs {
public static final FirewallArgs Empty = new FirewallArgs();
/**
* A flag indicating whether the firewall is protected against deletion. Use this setting to protect against accidentally deleting a firewall that is in use. Defaults to `false`.
*
*/
@Import(name="deleteProtection")
private @Nullable Output deleteProtection;
/**
* @return A flag indicating whether the firewall is protected against deletion. Use this setting to protect against accidentally deleting a firewall that is in use. Defaults to `false`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy