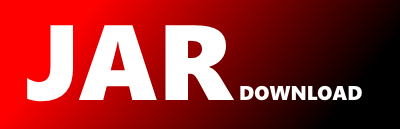
com.pulumi.aws.acmpca.inputs.CertificateAuthorityCertificateAuthorityConfigurationSubjectArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.acmpca.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class CertificateAuthorityCertificateAuthorityConfigurationSubjectArgs extends com.pulumi.resources.ResourceArgs {
public static final CertificateAuthorityCertificateAuthorityConfigurationSubjectArgs Empty = new CertificateAuthorityCertificateAuthorityConfigurationSubjectArgs();
/**
* Fully qualified domain name (FQDN) associated with the certificate subject. Must be less than or equal to 64 characters in length.
*
*/
@Import(name="commonName")
private @Nullable Output commonName;
/**
* @return Fully qualified domain name (FQDN) associated with the certificate subject. Must be less than or equal to 64 characters in length.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy