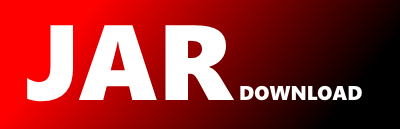
com.pulumi.aws.amplify.App Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.amplify;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.amplify.AppArgs;
import com.pulumi.aws.amplify.inputs.AppState;
import com.pulumi.aws.amplify.outputs.AppAutoBranchCreationConfig;
import com.pulumi.aws.amplify.outputs.AppCacheConfig;
import com.pulumi.aws.amplify.outputs.AppCustomRule;
import com.pulumi.aws.amplify.outputs.AppProductionBranch;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Amplify App resource, a fullstack serverless app hosted on the [AWS Amplify Console](https://docs.aws.amazon.com/amplify/latest/userguide/welcome.html).
*
* > **Note:** When you create/update an Amplify App from the provider, you may end up with the error "BadRequestException: You should at least provide one valid token" because of authentication issues. See the section "Repository with Tokens" below.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import com.pulumi.aws.amplify.inputs.AppCustomRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var example = new App("example", AppArgs.builder()
* .name("example")
* .repository("https://github.com/example/app")
* .buildSpec("""
* version: 0.1
* frontend:
* phases:
* preBuild:
* commands:
* - yarn install
* build:
* commands:
* - yarn run build
* artifacts:
* baseDirectory: build
* files:
* - '**}/{@code *'
* cache:
* paths:
* - node_modules/**}/{@code *
* """)
* .customRules(AppCustomRuleArgs.builder()
* .source("/<*>")
* .status("404")
* .target("/index.html")
* .build())
* .environmentVariables(Map.of("ENV", "test"))
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Repository with Tokens
*
* If you create a new Amplify App with the `repository` argument, you also need to set `oauth_token` or `access_token` for authentication. For GitHub, get a [personal access token](https://help.github.com/en/github/authenticating-to-github/creating-a-personal-access-token-for-the-command-line) and set `access_token` as follows:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new App("example", AppArgs.builder()
* .name("example")
* .repository("https://github.com/example/app")
* .accessToken("...")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* You can omit `access_token` if you import an existing Amplify App created by the Amplify Console (using OAuth for authentication).
*
* ### Auto Branch Creation
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import com.pulumi.aws.amplify.inputs.AppAutoBranchCreationConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* var example = new App("example", AppArgs.builder()
* .name("example")
* .enableAutoBranchCreation(true)
* .autoBranchCreationPatterns(
* "*",
* "*}/{@code **")
* .autoBranchCreationConfig(AppAutoBranchCreationConfigArgs.builder()
* .enableAutoBuild(true)
* .build())
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Basic Authorization
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new App("example", AppArgs.builder()
* .name("example")
* .enableBasicAuth(true)
* .basicAuthCredentials(StdFunctions.base64encode(Base64encodeArgs.builder()
* .input("username1:password1")
* .build()).result())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Rewrites and Redirects
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import com.pulumi.aws.amplify.inputs.AppCustomRuleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new App("example", AppArgs.builder()
* .name("example")
* .customRules(
* AppCustomRuleArgs.builder()
* .source("/api/<*>")
* .status("200")
* .target("https://api.example.com/api/<*>")
* .build(),
* AppCustomRuleArgs.builder()
* .source("^[^.]+$|\\.(?!(css|gif|ico|jpg|js|png|txt|svg|woff|ttf|map|json)$)([^.]+$)/>")
* .status("200")
* .target("/index.html")
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Custom Image
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new App("example", AppArgs.builder()
* .name("example")
* .environmentVariables(Map.of("_CUSTOM_IMAGE", "node:16"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Custom Headers
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.amplify.App;
* import com.pulumi.aws.amplify.AppArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new App("example", AppArgs.builder()
* .name("example")
* .customHeaders("""
* customHeaders:
* - pattern: '**'
* headers:
* - key: 'Strict-Transport-Security'
* value: 'max-age=31536000; includeSubDomains'
* - key: 'X-Frame-Options'
* value: 'SAMEORIGIN'
* - key: 'X-XSS-Protection'
* value: '1; mode=block'
* - key: 'X-Content-Type-Options'
* value: 'nosniff'
* - key: 'Content-Security-Policy'
* value: "default-src 'self'"
* """)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import Amplify App using Amplify App ID (appId). For example:
*
* ```sh
* $ pulumi import aws:amplify/app:App example d2ypk4k47z8u6
* ```
* App ID can be obtained from App ARN (e.g., `arn:aws:amplify:us-east-1:12345678:apps/d2ypk4k47z8u6`).
*
*/
@ResourceType(type="aws:amplify/app:App")
public class App extends com.pulumi.resources.CustomResource {
/**
* Personal access token for a third-party source control system for an Amplify app. This token must have write access to the relevant repo to create a webhook and a read-only deploy key for the Amplify project. The token is not stored, so after applying this attribute can be removed and the setup token deleted.
*
*/
@Export(name="accessToken", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> accessToken;
/**
* @return Personal access token for a third-party source control system for an Amplify app. This token must have write access to the relevant repo to create a webhook and a read-only deploy key for the Amplify project. The token is not stored, so after applying this attribute can be removed and the setup token deleted.
*
*/
public Output> accessToken() {
return Codegen.optional(this.accessToken);
}
/**
* ARN of the Amplify app.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the Amplify app.
*
*/
public Output arn() {
return this.arn;
}
/**
* Automated branch creation configuration for an Amplify app. See `auto_branch_creation_config` Block for details.
*
*/
@Export(name="autoBranchCreationConfig", refs={AppAutoBranchCreationConfig.class}, tree="[0]")
private Output autoBranchCreationConfig;
/**
* @return Automated branch creation configuration for an Amplify app. See `auto_branch_creation_config` Block for details.
*
*/
public Output autoBranchCreationConfig() {
return this.autoBranchCreationConfig;
}
/**
* Automated branch creation glob patterns for an Amplify app.
*
*/
@Export(name="autoBranchCreationPatterns", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> autoBranchCreationPatterns;
/**
* @return Automated branch creation glob patterns for an Amplify app.
*
*/
public Output>> autoBranchCreationPatterns() {
return Codegen.optional(this.autoBranchCreationPatterns);
}
/**
* Credentials for basic authorization for an Amplify app.
*
*/
@Export(name="basicAuthCredentials", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> basicAuthCredentials;
/**
* @return Credentials for basic authorization for an Amplify app.
*
*/
public Output> basicAuthCredentials() {
return Codegen.optional(this.basicAuthCredentials);
}
/**
* The [build specification](https://docs.aws.amazon.com/amplify/latest/userguide/build-settings.html) (build spec) for an Amplify app.
*
*/
@Export(name="buildSpec", refs={String.class}, tree="[0]")
private Output buildSpec;
/**
* @return The [build specification](https://docs.aws.amazon.com/amplify/latest/userguide/build-settings.html) (build spec) for an Amplify app.
*
*/
public Output buildSpec() {
return this.buildSpec;
}
/**
* Cache configuration for the Amplify app. See `cache_config` Block for details.
*
*/
@Export(name="cacheConfig", refs={AppCacheConfig.class}, tree="[0]")
private Output cacheConfig;
/**
* @return Cache configuration for the Amplify app. See `cache_config` Block for details.
*
*/
public Output cacheConfig() {
return this.cacheConfig;
}
/**
* The [custom HTTP headers](https://docs.aws.amazon.com/amplify/latest/userguide/custom-headers.html) for an Amplify app.
*
*/
@Export(name="customHeaders", refs={String.class}, tree="[0]")
private Output customHeaders;
/**
* @return The [custom HTTP headers](https://docs.aws.amazon.com/amplify/latest/userguide/custom-headers.html) for an Amplify app.
*
*/
public Output customHeaders() {
return this.customHeaders;
}
/**
* Custom rewrite and redirect rules for an Amplify app. See `custom_rule` Block for details.
*
*/
@Export(name="customRules", refs={List.class,AppCustomRule.class}, tree="[0,1]")
private Output* @Nullable */ List> customRules;
/**
* @return Custom rewrite and redirect rules for an Amplify app. See `custom_rule` Block for details.
*
*/
public Output>> customRules() {
return Codegen.optional(this.customRules);
}
/**
* Default domain for the Amplify app.
*
*/
@Export(name="defaultDomain", refs={String.class}, tree="[0]")
private Output defaultDomain;
/**
* @return Default domain for the Amplify app.
*
*/
public Output defaultDomain() {
return this.defaultDomain;
}
/**
* Description for an Amplify app.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Description for an Amplify app.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Enables automated branch creation for an Amplify app.
*
*/
@Export(name="enableAutoBranchCreation", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableAutoBranchCreation;
/**
* @return Enables automated branch creation for an Amplify app.
*
*/
public Output> enableAutoBranchCreation() {
return Codegen.optional(this.enableAutoBranchCreation);
}
/**
* Enables basic authorization for an Amplify app. This will apply to all branches that are part of this app.
*
*/
@Export(name="enableBasicAuth", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableBasicAuth;
/**
* @return Enables basic authorization for an Amplify app. This will apply to all branches that are part of this app.
*
*/
public Output> enableBasicAuth() {
return Codegen.optional(this.enableBasicAuth);
}
/**
* Enables auto-building of branches for the Amplify App.
*
*/
@Export(name="enableBranchAutoBuild", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableBranchAutoBuild;
/**
* @return Enables auto-building of branches for the Amplify App.
*
*/
public Output> enableBranchAutoBuild() {
return Codegen.optional(this.enableBranchAutoBuild);
}
/**
* Automatically disconnects a branch in the Amplify Console when you delete a branch from your Git repository.
*
*/
@Export(name="enableBranchAutoDeletion", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enableBranchAutoDeletion;
/**
* @return Automatically disconnects a branch in the Amplify Console when you delete a branch from your Git repository.
*
*/
public Output> enableBranchAutoDeletion() {
return Codegen.optional(this.enableBranchAutoDeletion);
}
/**
* Environment variables map for an Amplify app.
*
*/
@Export(name="environmentVariables", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> environmentVariables;
/**
* @return Environment variables map for an Amplify app.
*
*/
public Output>> environmentVariables() {
return Codegen.optional(this.environmentVariables);
}
/**
* AWS Identity and Access Management (IAM) service role for an Amplify app.
*
*/
@Export(name="iamServiceRoleArn", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> iamServiceRoleArn;
/**
* @return AWS Identity and Access Management (IAM) service role for an Amplify app.
*
*/
public Output> iamServiceRoleArn() {
return Codegen.optional(this.iamServiceRoleArn);
}
/**
* Name for an Amplify app.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name for an Amplify app.
*
*/
public Output name() {
return this.name;
}
/**
* OAuth token for a third-party source control system for an Amplify app. The OAuth token is used to create a webhook and a read-only deploy key. The OAuth token is not stored.
*
*/
@Export(name="oauthToken", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> oauthToken;
/**
* @return OAuth token for a third-party source control system for an Amplify app. The OAuth token is used to create a webhook and a read-only deploy key. The OAuth token is not stored.
*
*/
public Output> oauthToken() {
return Codegen.optional(this.oauthToken);
}
/**
* Platform or framework for an Amplify app. Valid values: `WEB`, `WEB_COMPUTE`. Default value: `WEB`.
*
*/
@Export(name="platform", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> platform;
/**
* @return Platform or framework for an Amplify app. Valid values: `WEB`, `WEB_COMPUTE`. Default value: `WEB`.
*
*/
public Output> platform() {
return Codegen.optional(this.platform);
}
/**
* Describes the information about a production branch for an Amplify app. A `production_branch` block is documented below.
*
*/
@Export(name="productionBranches", refs={List.class,AppProductionBranch.class}, tree="[0,1]")
private Output> productionBranches;
/**
* @return Describes the information about a production branch for an Amplify app. A `production_branch` block is documented below.
*
*/
public Output> productionBranches() {
return this.productionBranches;
}
/**
* Repository for an Amplify app.
*
*/
@Export(name="repository", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> repository;
/**
* @return Repository for an Amplify app.
*
*/
public Output> repository() {
return Codegen.optional(this.repository);
}
/**
* Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value mapping of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy