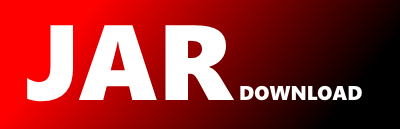
com.pulumi.aws.amplify.inputs.AppState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.amplify.inputs;
import com.pulumi.aws.amplify.inputs.AppAutoBranchCreationConfigArgs;
import com.pulumi.aws.amplify.inputs.AppCacheConfigArgs;
import com.pulumi.aws.amplify.inputs.AppCustomRuleArgs;
import com.pulumi.aws.amplify.inputs.AppProductionBranchArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AppState extends com.pulumi.resources.ResourceArgs {
public static final AppState Empty = new AppState();
/**
* Personal access token for a third-party source control system for an Amplify app. This token must have write access to the relevant repo to create a webhook and a read-only deploy key for the Amplify project. The token is not stored, so after applying this attribute can be removed and the setup token deleted.
*
*/
@Import(name="accessToken")
private @Nullable Output accessToken;
/**
* @return Personal access token for a third-party source control system for an Amplify app. This token must have write access to the relevant repo to create a webhook and a read-only deploy key for the Amplify project. The token is not stored, so after applying this attribute can be removed and the setup token deleted.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy