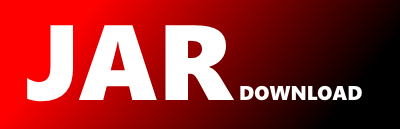
com.pulumi.aws.amplify.inputs.BranchState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.amplify.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class BranchState extends com.pulumi.resources.ResourceArgs {
public static final BranchState Empty = new BranchState();
/**
* Unique ID for an Amplify app.
*
*/
@Import(name="appId")
private @Nullable Output appId;
/**
* @return Unique ID for an Amplify app.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy