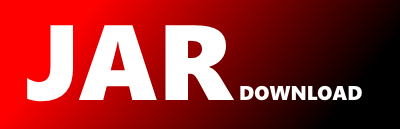
com.pulumi.aws.apigateway.inputs.DeploymentState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigateway.inputs;
import com.pulumi.aws.apigateway.inputs.DeploymentCanarySettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DeploymentState extends com.pulumi.resources.ResourceArgs {
public static final DeploymentState Empty = new DeploymentState();
/**
* Input configuration for the canary deployment when the deployment is a canary release deployment.
* See `canary_settings below.
* Has no effect when `stage_name` is not set.
*
* @deprecated
* The attribute "canary_settings" will be removed in a future major version. Use an explicit "aws.apigateway.Stage" instead.
*
*/
@Deprecated /* The attribute ""canary_settings"" will be removed in a future major version. Use an explicit ""aws.apigateway.Stage"" instead. */
@Import(name="canarySettings")
private @Nullable Output canarySettings;
/**
* @return Input configuration for the canary deployment when the deployment is a canary release deployment.
* See `canary_settings below.
* Has no effect when `stage_name` is not set.
*
* @deprecated
* The attribute "canary_settings" will be removed in a future major version. Use an explicit "aws.apigateway.Stage" instead.
*
*/
@Deprecated /* The attribute ""canary_settings"" will be removed in a future major version. Use an explicit ""aws.apigateway.Stage"" instead. */
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy