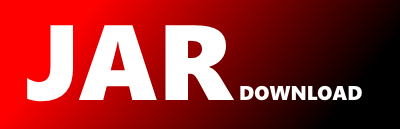
com.pulumi.aws.apigatewayv2.inputs.DomainNameDomainNameConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigatewayv2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DomainNameDomainNameConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final DomainNameDomainNameConfigurationArgs Empty = new DomainNameDomainNameConfigurationArgs();
/**
* ARN of an AWS-managed certificate that will be used by the endpoint for the domain name. AWS Certificate Manager is the only supported source. Use the `aws.acm.Certificate` resource to configure an ACM certificate.
*
*/
@Import(name="certificateArn", required=true)
private Output certificateArn;
/**
* @return ARN of an AWS-managed certificate that will be used by the endpoint for the domain name. AWS Certificate Manager is the only supported source. Use the `aws.acm.Certificate` resource to configure an ACM certificate.
*
*/
public Output certificateArn() {
return this.certificateArn;
}
/**
* Endpoint type. Valid values: `REGIONAL`.
*
*/
@Import(name="endpointType", required=true)
private Output endpointType;
/**
* @return Endpoint type. Valid values: `REGIONAL`.
*
*/
public Output endpointType() {
return this.endpointType;
}
/**
* Amazon Route 53 Hosted Zone ID of the endpoint.
*
*/
@Import(name="hostedZoneId")
private @Nullable Output hostedZoneId;
/**
* @return Amazon Route 53 Hosted Zone ID of the endpoint.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy