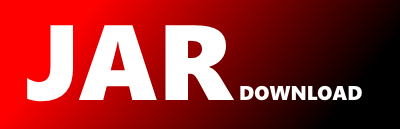
com.pulumi.aws.apigatewayv2.outputs.GetApiCorsConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apigatewayv2.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetApiCorsConfiguration {
/**
* @return Whether credentials are included in the CORS request.
*
*/
private Boolean allowCredentials;
/**
* @return Set of allowed HTTP headers.
*
*/
private List allowHeaders;
/**
* @return Set of allowed HTTP methods.
*
*/
private List allowMethods;
/**
* @return Set of allowed origins.
*
*/
private List allowOrigins;
/**
* @return Set of exposed HTTP headers.
*
*/
private List exposeHeaders;
/**
* @return Number of seconds that the browser should cache preflight request results.
*
*/
private Integer maxAge;
private GetApiCorsConfiguration() {}
/**
* @return Whether credentials are included in the CORS request.
*
*/
public Boolean allowCredentials() {
return this.allowCredentials;
}
/**
* @return Set of allowed HTTP headers.
*
*/
public List allowHeaders() {
return this.allowHeaders;
}
/**
* @return Set of allowed HTTP methods.
*
*/
public List allowMethods() {
return this.allowMethods;
}
/**
* @return Set of allowed origins.
*
*/
public List allowOrigins() {
return this.allowOrigins;
}
/**
* @return Set of exposed HTTP headers.
*
*/
public List exposeHeaders() {
return this.exposeHeaders;
}
/**
* @return Number of seconds that the browser should cache preflight request results.
*
*/
public Integer maxAge() {
return this.maxAge;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetApiCorsConfiguration defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean allowCredentials;
private List allowHeaders;
private List allowMethods;
private List allowOrigins;
private List exposeHeaders;
private Integer maxAge;
public Builder() {}
public Builder(GetApiCorsConfiguration defaults) {
Objects.requireNonNull(defaults);
this.allowCredentials = defaults.allowCredentials;
this.allowHeaders = defaults.allowHeaders;
this.allowMethods = defaults.allowMethods;
this.allowOrigins = defaults.allowOrigins;
this.exposeHeaders = defaults.exposeHeaders;
this.maxAge = defaults.maxAge;
}
@CustomType.Setter
public Builder allowCredentials(Boolean allowCredentials) {
if (allowCredentials == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "allowCredentials");
}
this.allowCredentials = allowCredentials;
return this;
}
@CustomType.Setter
public Builder allowHeaders(List allowHeaders) {
if (allowHeaders == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "allowHeaders");
}
this.allowHeaders = allowHeaders;
return this;
}
public Builder allowHeaders(String... allowHeaders) {
return allowHeaders(List.of(allowHeaders));
}
@CustomType.Setter
public Builder allowMethods(List allowMethods) {
if (allowMethods == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "allowMethods");
}
this.allowMethods = allowMethods;
return this;
}
public Builder allowMethods(String... allowMethods) {
return allowMethods(List.of(allowMethods));
}
@CustomType.Setter
public Builder allowOrigins(List allowOrigins) {
if (allowOrigins == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "allowOrigins");
}
this.allowOrigins = allowOrigins;
return this;
}
public Builder allowOrigins(String... allowOrigins) {
return allowOrigins(List.of(allowOrigins));
}
@CustomType.Setter
public Builder exposeHeaders(List exposeHeaders) {
if (exposeHeaders == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "exposeHeaders");
}
this.exposeHeaders = exposeHeaders;
return this;
}
public Builder exposeHeaders(String... exposeHeaders) {
return exposeHeaders(List.of(exposeHeaders));
}
@CustomType.Setter
public Builder maxAge(Integer maxAge) {
if (maxAge == null) {
throw new MissingRequiredPropertyException("GetApiCorsConfiguration", "maxAge");
}
this.maxAge = maxAge;
return this;
}
public GetApiCorsConfiguration build() {
final var _resultValue = new GetApiCorsConfiguration();
_resultValue.allowCredentials = allowCredentials;
_resultValue.allowHeaders = allowHeaders;
_resultValue.allowMethods = allowMethods;
_resultValue.allowOrigins = allowOrigins;
_resultValue.exposeHeaders = exposeHeaders;
_resultValue.maxAge = maxAge;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy