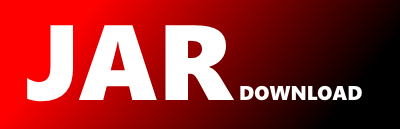
com.pulumi.aws.appautoscaling.ScheduledAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appautoscaling;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appautoscaling.ScheduledActionArgs;
import com.pulumi.aws.appautoscaling.inputs.ScheduledActionState;
import com.pulumi.aws.appautoscaling.outputs.ScheduledActionScalableTargetAction;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an Application AutoScaling ScheduledAction resource.
*
* ## Example Usage
*
* ### DynamoDB Table Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import com.pulumi.aws.appautoscaling.ScheduledAction;
* import com.pulumi.aws.appautoscaling.ScheduledActionArgs;
* import com.pulumi.aws.appautoscaling.inputs.ScheduledActionScalableTargetActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var dynamodb = new Target("dynamodb", TargetArgs.builder()
* .maxCapacity(100)
* .minCapacity(5)
* .resourceId("table/tableName")
* .scalableDimension("dynamodb:table:ReadCapacityUnits")
* .serviceNamespace("dynamodb")
* .build());
*
* var dynamodbScheduledAction = new ScheduledAction("dynamodbScheduledAction", ScheduledActionArgs.builder()
* .name("dynamodb")
* .serviceNamespace(dynamodb.serviceNamespace())
* .resourceId(dynamodb.resourceId())
* .scalableDimension(dynamodb.scalableDimension())
* .schedule("at(2006-01-02T15:04:05)")
* .scalableTargetAction(ScheduledActionScalableTargetActionArgs.builder()
* .minCapacity(1)
* .maxCapacity(200)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### ECS Service Autoscaling
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.appautoscaling.Target;
* import com.pulumi.aws.appautoscaling.TargetArgs;
* import com.pulumi.aws.appautoscaling.ScheduledAction;
* import com.pulumi.aws.appautoscaling.ScheduledActionArgs;
* import com.pulumi.aws.appautoscaling.inputs.ScheduledActionScalableTargetActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var ecs = new Target("ecs", TargetArgs.builder()
* .maxCapacity(4)
* .minCapacity(1)
* .resourceId("service/clusterName/serviceName")
* .scalableDimension("ecs:service:DesiredCount")
* .serviceNamespace("ecs")
* .build());
*
* var ecsScheduledAction = new ScheduledAction("ecsScheduledAction", ScheduledActionArgs.builder()
* .name("ecs")
* .serviceNamespace(ecs.serviceNamespace())
* .resourceId(ecs.resourceId())
* .scalableDimension(ecs.scalableDimension())
* .schedule("at(2006-01-02T15:04:05)")
* .scalableTargetAction(ScheduledActionScalableTargetActionArgs.builder()
* .minCapacity(1)
* .maxCapacity(10)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:appautoscaling/scheduledAction:ScheduledAction")
public class ScheduledAction extends com.pulumi.resources.CustomResource {
/**
* ARN of the scheduled action.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the scheduled action.
*
*/
public Output arn() {
return this.arn;
}
/**
* Date and time for the scheduled action to end in RFC 3339 format. The timezone is not affected by the setting of `timezone`.
*
*/
@Export(name="endTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> endTime;
/**
* @return Date and time for the scheduled action to end in RFC 3339 format. The timezone is not affected by the setting of `timezone`.
*
*/
public Output> endTime() {
return Codegen.optional(this.endTime);
}
/**
* Name of the scheduled action.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Name of the scheduled action.
*
*/
public Output name() {
return this.name;
}
/**
* Identifier of the resource associated with the scheduled action. Documentation can be found in the `ResourceId` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html)
*
*/
@Export(name="resourceId", refs={String.class}, tree="[0]")
private Output resourceId;
/**
* @return Identifier of the resource associated with the scheduled action. Documentation can be found in the `ResourceId` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html)
*
*/
public Output resourceId() {
return this.resourceId;
}
/**
* Scalable dimension. Documentation can be found in the `ScalableDimension` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html) Example: ecs:service:DesiredCount
*
*/
@Export(name="scalableDimension", refs={String.class}, tree="[0]")
private Output scalableDimension;
/**
* @return Scalable dimension. Documentation can be found in the `ScalableDimension` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html) Example: ecs:service:DesiredCount
*
*/
public Output scalableDimension() {
return this.scalableDimension;
}
/**
* New minimum and maximum capacity. You can set both values or just one. See below
*
*/
@Export(name="scalableTargetAction", refs={ScheduledActionScalableTargetAction.class}, tree="[0]")
private Output scalableTargetAction;
/**
* @return New minimum and maximum capacity. You can set both values or just one. See below
*
*/
public Output scalableTargetAction() {
return this.scalableTargetAction;
}
/**
* Schedule for this action. The following formats are supported: At expressions - at(yyyy-mm-ddThh:mm:ss), Rate expressions - rate(valueunit), Cron expressions - cron(fields). Times for at expressions and cron expressions are evaluated using the time zone configured in `timezone`. Documentation can be found in the `Timezone` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html)
*
*/
@Export(name="schedule", refs={String.class}, tree="[0]")
private Output schedule;
/**
* @return Schedule for this action. The following formats are supported: At expressions - at(yyyy-mm-ddThh:mm:ss), Rate expressions - rate(valueunit), Cron expressions - cron(fields). Times for at expressions and cron expressions are evaluated using the time zone configured in `timezone`. Documentation can be found in the `Timezone` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html)
*
*/
public Output schedule() {
return this.schedule;
}
/**
* Namespace of the AWS service. Documentation can be found in the `ServiceNamespace` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html) Example: ecs
*
*/
@Export(name="serviceNamespace", refs={String.class}, tree="[0]")
private Output serviceNamespace;
/**
* @return Namespace of the AWS service. Documentation can be found in the `ServiceNamespace` parameter at: [AWS Application Auto Scaling API Reference](https://docs.aws.amazon.com/autoscaling/application/APIReference/API_PutScheduledAction.html) Example: ecs
*
*/
public Output serviceNamespace() {
return this.serviceNamespace;
}
/**
* Date and time for the scheduled action to start in RFC 3339 format. The timezone is not affected by the setting of `timezone`.
*
*/
@Export(name="startTime", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> startTime;
/**
* @return Date and time for the scheduled action to start in RFC 3339 format. The timezone is not affected by the setting of `timezone`.
*
*/
public Output> startTime() {
return Codegen.optional(this.startTime);
}
/**
* Time zone used when setting a scheduled action by using an at or cron expression. Does not affect timezone for `start_time` and `end_time`. Valid values are the [canonical names of the IANA time zones supported by Joda-Time](https://www.joda.org/joda-time/timezones.html), such as `Etc/GMT+9` or `Pacific/Tahiti`. Default is `UTC`.
*
*/
@Export(name="timezone", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> timezone;
/**
* @return Time zone used when setting a scheduled action by using an at or cron expression. Does not affect timezone for `start_time` and `end_time`. Valid values are the [canonical names of the IANA time zones supported by Joda-Time](https://www.joda.org/joda-time/timezones.html), such as `Etc/GMT+9` or `Pacific/Tahiti`. Default is `UTC`.
*
*/
public Output> timezone() {
return Codegen.optional(this.timezone);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ScheduledAction(java.lang.String name) {
this(name, ScheduledActionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ScheduledAction(java.lang.String name, ScheduledActionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ScheduledAction(java.lang.String name, ScheduledActionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:appautoscaling/scheduledAction:ScheduledAction", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ScheduledAction(java.lang.String name, Output id, @Nullable ScheduledActionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:appautoscaling/scheduledAction:ScheduledAction", name, state, makeResourceOptions(options, id), false);
}
private static ScheduledActionArgs makeArgs(ScheduledActionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ScheduledActionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ScheduledAction get(java.lang.String name, Output id, @Nullable ScheduledActionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ScheduledAction(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy