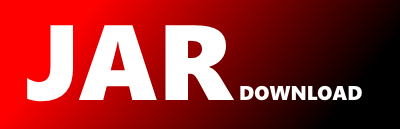
com.pulumi.aws.appflow.ConnectorProfile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appflow;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.appflow.ConnectorProfileArgs;
import com.pulumi.aws.appflow.inputs.ConnectorProfileState;
import com.pulumi.aws.appflow.outputs.ConnectorProfileConnectorProfileConfig;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an AppFlow connector profile resource.
*
* For information about AppFlow flows, see the [Amazon AppFlow API Reference](https://docs.aws.amazon.com/appflow/1.0/APIReference/Welcome.html).
* For specific information about creating an AppFlow connector profile, see the
* [CreateConnectorProfile](https://docs.aws.amazon.com/appflow/1.0/APIReference/API_CreateConnectorProfile.html) page in the Amazon AppFlow API Reference.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.iam.IamFunctions;
* import com.pulumi.aws.iam.inputs.GetPolicyArgs;
* import com.pulumi.aws.iam.Role;
* import com.pulumi.aws.iam.RoleArgs;
* import com.pulumi.aws.s3.BucketV2;
* import com.pulumi.aws.s3.BucketV2Args;
* import com.pulumi.aws.redshift.Cluster;
* import com.pulumi.aws.redshift.ClusterArgs;
* import com.pulumi.aws.appflow.ConnectorProfile;
* import com.pulumi.aws.appflow.ConnectorProfileArgs;
* import com.pulumi.aws.appflow.inputs.ConnectorProfileConnectorProfileConfigArgs;
* import com.pulumi.aws.appflow.inputs.ConnectorProfileConnectorProfileConfigConnectorProfileCredentialsArgs;
* import com.pulumi.aws.appflow.inputs.ConnectorProfileConnectorProfileConfigConnectorProfileCredentialsRedshiftArgs;
* import com.pulumi.aws.appflow.inputs.ConnectorProfileConnectorProfileConfigConnectorProfilePropertiesArgs;
* import com.pulumi.aws.appflow.inputs.ConnectorProfileConnectorProfileConfigConnectorProfilePropertiesRedshiftArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var example = IamFunctions.getPolicy(GetPolicyArgs.builder()
* .name("AmazonRedshiftAllCommandsFullAccess")
* .build());
*
* var exampleRole = new Role("exampleRole", RoleArgs.builder()
* .name("example_role")
* .managedPolicyArns(test.arn())
* .assumeRolePolicy(serializeJson(
* jsonObject(
* jsonProperty("Version", "2012-10-17"),
* jsonProperty("Statement", jsonArray(jsonObject(
* jsonProperty("Action", "sts:AssumeRole"),
* jsonProperty("Effect", "Allow"),
* jsonProperty("Sid", ""),
* jsonProperty("Principal", jsonObject(
* jsonProperty("Service", "ec2.amazonaws.com")
* ))
* )))
* )))
* .build());
*
* var exampleBucketV2 = new BucketV2("exampleBucketV2", BucketV2Args.builder()
* .bucket("example-bucket")
* .build());
*
* var exampleCluster = new Cluster("exampleCluster", ClusterArgs.builder()
* .clusterIdentifier("example_cluster")
* .databaseName("example_db")
* .masterUsername("exampleuser")
* .masterPassword("examplePassword123!")
* .nodeType("dc1.large")
* .clusterType("single-node")
* .build());
*
* var exampleConnectorProfile = new ConnectorProfile("exampleConnectorProfile", ConnectorProfileArgs.builder()
* .name("example_profile")
* .connectorType("Redshift")
* .connectionMode("Public")
* .connectorProfileConfig(ConnectorProfileConnectorProfileConfigArgs.builder()
* .connectorProfileCredentials(ConnectorProfileConnectorProfileConfigConnectorProfileCredentialsArgs.builder()
* .redshift(ConnectorProfileConnectorProfileConfigConnectorProfileCredentialsRedshiftArgs.builder()
* .password(exampleCluster.masterPassword())
* .username(exampleCluster.masterUsername())
* .build())
* .build())
* .connectorProfileProperties(ConnectorProfileConnectorProfileConfigConnectorProfilePropertiesArgs.builder()
* .redshift(ConnectorProfileConnectorProfileConfigConnectorProfilePropertiesRedshiftArgs.builder()
* .bucketName(exampleBucketV2.name())
* .databaseUrl(Output.tuple(exampleCluster.endpoint(), exampleCluster.databaseName()).applyValue(values -> {
* var endpoint = values.t1;
* var databaseName = values.t2;
* return String.format("jdbc:redshift://%s/%s", endpoint,databaseName);
* }))
* .roleArn(exampleRole.arn())
* .build())
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import AppFlow Connector Profile using the connector profile `arn`. For example:
*
* ```sh
* $ pulumi import aws:appflow/connectorProfile:ConnectorProfile profile arn:aws:appflow:us-west-2:123456789012:connectorprofile/example-profile
* ```
*
*/
@ResourceType(type="aws:appflow/connectorProfile:ConnectorProfile")
public class ConnectorProfile extends com.pulumi.resources.CustomResource {
/**
* ARN of the connector profile.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return ARN of the connector profile.
*
*/
public Output arn() {
return this.arn;
}
/**
* Indicates the connection mode and specifies whether it is public or private. Private flows use AWS PrivateLink to route data over AWS infrastructure without exposing it to the public internet. One of: `Public`, `Private`.
*
*/
@Export(name="connectionMode", refs={String.class}, tree="[0]")
private Output connectionMode;
/**
* @return Indicates the connection mode and specifies whether it is public or private. Private flows use AWS PrivateLink to route data over AWS infrastructure without exposing it to the public internet. One of: `Public`, `Private`.
*
*/
public Output connectionMode() {
return this.connectionMode;
}
/**
* The label of the connector. The label is unique for each ConnectorRegistration in your AWS account. Only needed if calling for `CustomConnector` connector type.
*
*/
@Export(name="connectorLabel", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> connectorLabel;
/**
* @return The label of the connector. The label is unique for each ConnectorRegistration in your AWS account. Only needed if calling for `CustomConnector` connector type.
*
*/
public Output> connectorLabel() {
return Codegen.optional(this.connectorLabel);
}
/**
* Defines the connector-specific configuration and credentials. See Connector Profile Config for more details.
*
*/
@Export(name="connectorProfileConfig", refs={ConnectorProfileConnectorProfileConfig.class}, tree="[0]")
private Output connectorProfileConfig;
/**
* @return Defines the connector-specific configuration and credentials. See Connector Profile Config for more details.
*
*/
public Output connectorProfileConfig() {
return this.connectorProfileConfig;
}
/**
* The type of connector. One of: `Amplitude`, `CustomConnector`, `CustomerProfiles`, `Datadog`, `Dynatrace`, `EventBridge`, `Googleanalytics`, `Honeycode`, `Infornexus`, `LookoutMetrics`, `Marketo`, `Redshift`, `S3`, `Salesforce`, `SAPOData`, `Servicenow`, `Singular`, `Slack`, `Snowflake`, `Trendmicro`, `Upsolver`, `Veeva`, `Zendesk`.
*
*/
@Export(name="connectorType", refs={String.class}, tree="[0]")
private Output connectorType;
/**
* @return The type of connector. One of: `Amplitude`, `CustomConnector`, `CustomerProfiles`, `Datadog`, `Dynatrace`, `EventBridge`, `Googleanalytics`, `Honeycode`, `Infornexus`, `LookoutMetrics`, `Marketo`, `Redshift`, `S3`, `Salesforce`, `SAPOData`, `Servicenow`, `Singular`, `Slack`, `Snowflake`, `Trendmicro`, `Upsolver`, `Veeva`, `Zendesk`.
*
*/
public Output connectorType() {
return this.connectorType;
}
/**
* ARN of the connector profile credentials.
*
*/
@Export(name="credentialsArn", refs={String.class}, tree="[0]")
private Output credentialsArn;
/**
* @return ARN of the connector profile credentials.
*
*/
public Output credentialsArn() {
return this.credentialsArn;
}
/**
* ARN (Amazon Resource Name) of the Key Management Service (KMS) key you provide for encryption. This is required if you do not want to use the Amazon AppFlow-managed KMS key. If you don't provide anything here, Amazon AppFlow uses the Amazon AppFlow-managed KMS key.
*
*/
@Export(name="kmsArn", refs={String.class}, tree="[0]")
private Output kmsArn;
/**
* @return ARN (Amazon Resource Name) of the Key Management Service (KMS) key you provide for encryption. This is required if you do not want to use the Amazon AppFlow-managed KMS key. If you don't provide anything here, Amazon AppFlow uses the Amazon AppFlow-managed KMS key.
*
*/
public Output kmsArn() {
return this.kmsArn;
}
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
public Output name() {
return this.name;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ConnectorProfile(java.lang.String name) {
this(name, ConnectorProfileArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ConnectorProfile(java.lang.String name, ConnectorProfileArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ConnectorProfile(java.lang.String name, ConnectorProfileArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:appflow/connectorProfile:ConnectorProfile", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ConnectorProfile(java.lang.String name, Output id, @Nullable ConnectorProfileState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:appflow/connectorProfile:ConnectorProfile", name, state, makeResourceOptions(options, id), false);
}
private static ConnectorProfileArgs makeArgs(ConnectorProfileArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ConnectorProfileArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ConnectorProfile get(java.lang.String name, Output id, @Nullable ConnectorProfileState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ConnectorProfile(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy