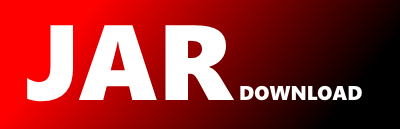
com.pulumi.aws.apprunner.VpcIngressConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.apprunner;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.apprunner.VpcIngressConnectionArgs;
import com.pulumi.aws.apprunner.inputs.VpcIngressConnectionState;
import com.pulumi.aws.apprunner.outputs.VpcIngressConnectionIngressVpcConfiguration;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an App Runner VPC Ingress Connection.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.apprunner.VpcIngressConnection;
* import com.pulumi.aws.apprunner.VpcIngressConnectionArgs;
* import com.pulumi.aws.apprunner.inputs.VpcIngressConnectionIngressVpcConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new VpcIngressConnection("example", VpcIngressConnectionArgs.builder()
* .name("example")
* .serviceArn(exampleAwsApprunnerService.arn())
* .ingressVpcConfiguration(VpcIngressConnectionIngressVpcConfigurationArgs.builder()
* .vpcId(default_.id())
* .vpcEndpointId(apprunner.id())
* .build())
* .tags(Map.of("foo", "bar"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import App Runner VPC Ingress Connection using the `arn`. For example:
*
* ```sh
* $ pulumi import aws:apprunner/vpcIngressConnection:VpcIngressConnection example "arn:aws:apprunner:us-west-2:837424938642:vpcingressconnection/example/b379f86381d74825832c2e82080342fa"
* ```
*
*/
@ResourceType(type="aws:apprunner/vpcIngressConnection:VpcIngressConnection")
public class VpcIngressConnection extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) of the VPC Ingress Connection.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) of the VPC Ingress Connection.
*
*/
public Output arn() {
return this.arn;
}
/**
* The domain name associated with the VPC Ingress Connection resource.
*
*/
@Export(name="domainName", refs={String.class}, tree="[0]")
private Output domainName;
/**
* @return The domain name associated with the VPC Ingress Connection resource.
*
*/
public Output domainName() {
return this.domainName;
}
/**
* Specifications for the customer’s Amazon VPC and the related AWS PrivateLink VPC endpoint that are used to create the VPC Ingress Connection resource. See Ingress VPC Configuration below for more details.
*
*/
@Export(name="ingressVpcConfiguration", refs={VpcIngressConnectionIngressVpcConfiguration.class}, tree="[0]")
private Output ingressVpcConfiguration;
/**
* @return Specifications for the customer’s Amazon VPC and the related AWS PrivateLink VPC endpoint that are used to create the VPC Ingress Connection resource. See Ingress VPC Configuration below for more details.
*
*/
public Output ingressVpcConfiguration() {
return this.ingressVpcConfiguration;
}
/**
* A name for the VPC Ingress Connection resource. It must be unique across all the active VPC Ingress Connections in your AWS account in the AWS Region.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return A name for the VPC Ingress Connection resource. It must be unique across all the active VPC Ingress Connections in your AWS account in the AWS Region.
*
*/
public Output name() {
return this.name;
}
/**
* The Amazon Resource Name (ARN) for this App Runner service that is used to create the VPC Ingress Connection resource.
*
*/
@Export(name="serviceArn", refs={String.class}, tree="[0]")
private Output serviceArn;
/**
* @return The Amazon Resource Name (ARN) for this App Runner service that is used to create the VPC Ingress Connection resource.
*
*/
public Output serviceArn() {
return this.serviceArn;
}
/**
* The current status of the VPC Ingress Connection.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The current status of the VPC Ingress Connection.
*
*/
public Output status() {
return this.status;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* Map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy