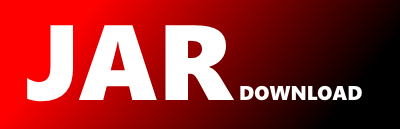
com.pulumi.aws.appstream.StackArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.appstream;
import com.pulumi.aws.appstream.inputs.StackAccessEndpointArgs;
import com.pulumi.aws.appstream.inputs.StackApplicationSettingsArgs;
import com.pulumi.aws.appstream.inputs.StackStorageConnectorArgs;
import com.pulumi.aws.appstream.inputs.StackStreamingExperienceSettingsArgs;
import com.pulumi.aws.appstream.inputs.StackUserSettingArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class StackArgs extends com.pulumi.resources.ResourceArgs {
public static final StackArgs Empty = new StackArgs();
/**
* Set of configuration blocks defining the interface VPC endpoints. Users of the stack can connect to AppStream 2.0 only through the specified endpoints.
* See `access_endpoints` below.
*
*/
@Import(name="accessEndpoints")
private @Nullable Output> accessEndpoints;
/**
* @return Set of configuration blocks defining the interface VPC endpoints. Users of the stack can connect to AppStream 2.0 only through the specified endpoints.
* See `access_endpoints` below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy