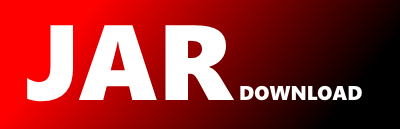
com.pulumi.aws.autoscaling.inputs.GroupWarmPoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.autoscaling.inputs;
import com.pulumi.aws.autoscaling.inputs.GroupWarmPoolInstanceReusePolicyArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GroupWarmPoolArgs extends com.pulumi.resources.ResourceArgs {
public static final GroupWarmPoolArgs Empty = new GroupWarmPoolArgs();
/**
* Whether instances in the Auto Scaling group can be returned to the warm pool on scale in. The default is to terminate instances in the Auto Scaling group when the group scales in.
*
*/
@Import(name="instanceReusePolicy")
private @Nullable Output instanceReusePolicy;
/**
* @return Whether instances in the Auto Scaling group can be returned to the warm pool on scale in. The default is to terminate instances in the Auto Scaling group when the group scales in.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy