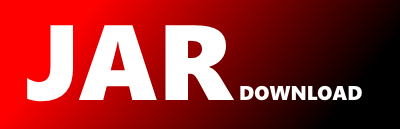
com.pulumi.aws.batch.outputs.GetJobQueueResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch.outputs;
import com.pulumi.aws.batch.outputs.GetJobQueueComputeEnvironmentOrder;
import com.pulumi.aws.batch.outputs.GetJobQueueJobStateTimeLimitAction;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetJobQueueResult {
/**
* @return ARN of the job queue.
*
*/
private String arn;
/**
* @return The compute environments that are attached to the job queue and the order in
* which job placement is preferred. Compute environments are selected for job placement in ascending order.
* * `compute_environment_order.#.order` - The order of the compute environment.
* * `compute_environment_order.#.compute_environment` - The ARN of the compute environment.
*
*/
private List computeEnvironmentOrders;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Specifies an action that AWS Batch will take after the job has remained at the head of the queue in the specified state for longer than the specified time.
* * `job_state_time_limit_action.#.action` - The action to take when a job is at the head of the job queue in the specified state for the specified period of time.
* * `job_state_time_limit_action.#.max_time_seconds` - The approximate amount of time, in seconds, that must pass with the job in the specified state before the action is taken.
* * `job_state_time_limit_action.#.reason` - The reason to log for the action being taken.
* * `job_state_time_limit_action.#.state` - The state of the job needed to trigger the action.
*
*/
private List jobStateTimeLimitActions;
private String name;
/**
* @return Priority of the job queue. Job queues with a higher priority are evaluated first when
* associated with the same compute environment.
*
*/
private Integer priority;
/**
* @return The ARN of the fair share scheduling policy. If this attribute has a value, the job queue uses a fair share scheduling policy. If this attribute does not have a value, the job queue uses a first in, first out (FIFO) scheduling policy.
*
*/
private String schedulingPolicyArn;
/**
* @return Describes the ability of the queue to accept new jobs (for example, `ENABLED` or `DISABLED`).
*
*/
private String state;
/**
* @return Current status of the job queue (for example, `CREATING` or `VALID`).
*
*/
private String status;
/**
* @return Short, human-readable string to provide additional details about the current status
* of the job queue.
*
*/
private String statusReason;
/**
* @return Key-value map of resource tags
*
*/
private Map tags;
private GetJobQueueResult() {}
/**
* @return ARN of the job queue.
*
*/
public String arn() {
return this.arn;
}
/**
* @return The compute environments that are attached to the job queue and the order in
* which job placement is preferred. Compute environments are selected for job placement in ascending order.
* * `compute_environment_order.#.order` - The order of the compute environment.
* * `compute_environment_order.#.compute_environment` - The ARN of the compute environment.
*
*/
public List computeEnvironmentOrders() {
return this.computeEnvironmentOrders;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Specifies an action that AWS Batch will take after the job has remained at the head of the queue in the specified state for longer than the specified time.
* * `job_state_time_limit_action.#.action` - The action to take when a job is at the head of the job queue in the specified state for the specified period of time.
* * `job_state_time_limit_action.#.max_time_seconds` - The approximate amount of time, in seconds, that must pass with the job in the specified state before the action is taken.
* * `job_state_time_limit_action.#.reason` - The reason to log for the action being taken.
* * `job_state_time_limit_action.#.state` - The state of the job needed to trigger the action.
*
*/
public List jobStateTimeLimitActions() {
return this.jobStateTimeLimitActions;
}
public String name() {
return this.name;
}
/**
* @return Priority of the job queue. Job queues with a higher priority are evaluated first when
* associated with the same compute environment.
*
*/
public Integer priority() {
return this.priority;
}
/**
* @return The ARN of the fair share scheduling policy. If this attribute has a value, the job queue uses a fair share scheduling policy. If this attribute does not have a value, the job queue uses a first in, first out (FIFO) scheduling policy.
*
*/
public String schedulingPolicyArn() {
return this.schedulingPolicyArn;
}
/**
* @return Describes the ability of the queue to accept new jobs (for example, `ENABLED` or `DISABLED`).
*
*/
public String state() {
return this.state;
}
/**
* @return Current status of the job queue (for example, `CREATING` or `VALID`).
*
*/
public String status() {
return this.status;
}
/**
* @return Short, human-readable string to provide additional details about the current status
* of the job queue.
*
*/
public String statusReason() {
return this.statusReason;
}
/**
* @return Key-value map of resource tags
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetJobQueueResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List computeEnvironmentOrders;
private String id;
private List jobStateTimeLimitActions;
private String name;
private Integer priority;
private String schedulingPolicyArn;
private String state;
private String status;
private String statusReason;
private Map tags;
public Builder() {}
public Builder(GetJobQueueResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.computeEnvironmentOrders = defaults.computeEnvironmentOrders;
this.id = defaults.id;
this.jobStateTimeLimitActions = defaults.jobStateTimeLimitActions;
this.name = defaults.name;
this.priority = defaults.priority;
this.schedulingPolicyArn = defaults.schedulingPolicyArn;
this.state = defaults.state;
this.status = defaults.status;
this.statusReason = defaults.statusReason;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder computeEnvironmentOrders(List computeEnvironmentOrders) {
if (computeEnvironmentOrders == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "computeEnvironmentOrders");
}
this.computeEnvironmentOrders = computeEnvironmentOrders;
return this;
}
public Builder computeEnvironmentOrders(GetJobQueueComputeEnvironmentOrder... computeEnvironmentOrders) {
return computeEnvironmentOrders(List.of(computeEnvironmentOrders));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder jobStateTimeLimitActions(List jobStateTimeLimitActions) {
if (jobStateTimeLimitActions == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "jobStateTimeLimitActions");
}
this.jobStateTimeLimitActions = jobStateTimeLimitActions;
return this;
}
public Builder jobStateTimeLimitActions(GetJobQueueJobStateTimeLimitAction... jobStateTimeLimitActions) {
return jobStateTimeLimitActions(List.of(jobStateTimeLimitActions));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder priority(Integer priority) {
if (priority == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "priority");
}
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder schedulingPolicyArn(String schedulingPolicyArn) {
if (schedulingPolicyArn == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "schedulingPolicyArn");
}
this.schedulingPolicyArn = schedulingPolicyArn;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder statusReason(String statusReason) {
if (statusReason == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "statusReason");
}
this.statusReason = statusReason;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetJobQueueResult", "tags");
}
this.tags = tags;
return this;
}
public GetJobQueueResult build() {
final var _resultValue = new GetJobQueueResult();
_resultValue.arn = arn;
_resultValue.computeEnvironmentOrders = computeEnvironmentOrders;
_resultValue.id = id;
_resultValue.jobStateTimeLimitActions = jobStateTimeLimitActions;
_resultValue.name = name;
_resultValue.priority = priority;
_resultValue.schedulingPolicyArn = schedulingPolicyArn;
_resultValue.state = state;
_resultValue.status = status;
_resultValue.statusReason = statusReason;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy