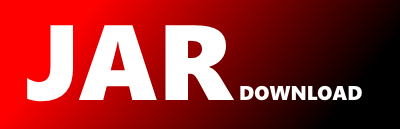
com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.batch.outputs;
import com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodPropertiesContainers;
import com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodPropertiesImagePullSecret;
import com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodPropertiesInitContainer;
import com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodPropertiesMetadata;
import com.pulumi.aws.batch.outputs.JobDefinitionEksPropertiesPodPropertiesVolume;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class JobDefinitionEksPropertiesPodProperties {
/**
* @return Properties of the container that's used on the Amazon EKS pod. See containers below.
*
*/
private JobDefinitionEksPropertiesPodPropertiesContainers containers;
/**
* @return DNS policy for the pod. The default value is `ClusterFirst`. If the `host_network` argument is not specified, the default is `ClusterFirstWithHostNet`. `ClusterFirst` indicates that any DNS query that does not match the configured cluster domain suffix is forwarded to the upstream nameserver inherited from the node. For more information, see Pod's DNS policy in the Kubernetes documentation.
*
*/
private @Nullable String dnsPolicy;
/**
* @return Whether the pod uses the hosts' network IP address. The default value is `true`. Setting this to `false` enables the Kubernetes pod networking model. Most AWS Batch workloads are egress-only and don't require the overhead of IP allocation for each pod for incoming connections.
*
*/
private @Nullable Boolean hostNetwork;
/**
* @return List of Kubernetes secret resources. See `image_pull_secret` below.
*
*/
private @Nullable List imagePullSecrets;
/**
* @return Containers which run before application containers, always runs to completion, and must complete successfully before the next container starts. These containers are registered with the Amazon EKS Connector agent and persists the registration information in the Kubernetes backend data store. See containers below.
*
*/
private @Nullable List initContainers;
/**
* @return Metadata about the Kubernetes pod.
*
*/
private @Nullable JobDefinitionEksPropertiesPodPropertiesMetadata metadata;
/**
* @return Name of the service account that's used to run the pod.
*
*/
private @Nullable String serviceAccountName;
/**
* @return Indicates if the processes in a container are shared, or visible, to other containers in the same pod.
*
*/
private @Nullable Boolean shareProcessNamespace;
/**
* @return Volumes for a job definition that uses Amazon EKS resources. AWS Batch supports emptyDir, hostPath, and secret volume types.
*
*/
private @Nullable List volumes;
private JobDefinitionEksPropertiesPodProperties() {}
/**
* @return Properties of the container that's used on the Amazon EKS pod. See containers below.
*
*/
public JobDefinitionEksPropertiesPodPropertiesContainers containers() {
return this.containers;
}
/**
* @return DNS policy for the pod. The default value is `ClusterFirst`. If the `host_network` argument is not specified, the default is `ClusterFirstWithHostNet`. `ClusterFirst` indicates that any DNS query that does not match the configured cluster domain suffix is forwarded to the upstream nameserver inherited from the node. For more information, see Pod's DNS policy in the Kubernetes documentation.
*
*/
public Optional dnsPolicy() {
return Optional.ofNullable(this.dnsPolicy);
}
/**
* @return Whether the pod uses the hosts' network IP address. The default value is `true`. Setting this to `false` enables the Kubernetes pod networking model. Most AWS Batch workloads are egress-only and don't require the overhead of IP allocation for each pod for incoming connections.
*
*/
public Optional hostNetwork() {
return Optional.ofNullable(this.hostNetwork);
}
/**
* @return List of Kubernetes secret resources. See `image_pull_secret` below.
*
*/
public List imagePullSecrets() {
return this.imagePullSecrets == null ? List.of() : this.imagePullSecrets;
}
/**
* @return Containers which run before application containers, always runs to completion, and must complete successfully before the next container starts. These containers are registered with the Amazon EKS Connector agent and persists the registration information in the Kubernetes backend data store. See containers below.
*
*/
public List initContainers() {
return this.initContainers == null ? List.of() : this.initContainers;
}
/**
* @return Metadata about the Kubernetes pod.
*
*/
public Optional metadata() {
return Optional.ofNullable(this.metadata);
}
/**
* @return Name of the service account that's used to run the pod.
*
*/
public Optional serviceAccountName() {
return Optional.ofNullable(this.serviceAccountName);
}
/**
* @return Indicates if the processes in a container are shared, or visible, to other containers in the same pod.
*
*/
public Optional shareProcessNamespace() {
return Optional.ofNullable(this.shareProcessNamespace);
}
/**
* @return Volumes for a job definition that uses Amazon EKS resources. AWS Batch supports emptyDir, hostPath, and secret volume types.
*
*/
public List volumes() {
return this.volumes == null ? List.of() : this.volumes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(JobDefinitionEksPropertiesPodProperties defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private JobDefinitionEksPropertiesPodPropertiesContainers containers;
private @Nullable String dnsPolicy;
private @Nullable Boolean hostNetwork;
private @Nullable List imagePullSecrets;
private @Nullable List initContainers;
private @Nullable JobDefinitionEksPropertiesPodPropertiesMetadata metadata;
private @Nullable String serviceAccountName;
private @Nullable Boolean shareProcessNamespace;
private @Nullable List volumes;
public Builder() {}
public Builder(JobDefinitionEksPropertiesPodProperties defaults) {
Objects.requireNonNull(defaults);
this.containers = defaults.containers;
this.dnsPolicy = defaults.dnsPolicy;
this.hostNetwork = defaults.hostNetwork;
this.imagePullSecrets = defaults.imagePullSecrets;
this.initContainers = defaults.initContainers;
this.metadata = defaults.metadata;
this.serviceAccountName = defaults.serviceAccountName;
this.shareProcessNamespace = defaults.shareProcessNamespace;
this.volumes = defaults.volumes;
}
@CustomType.Setter
public Builder containers(JobDefinitionEksPropertiesPodPropertiesContainers containers) {
if (containers == null) {
throw new MissingRequiredPropertyException("JobDefinitionEksPropertiesPodProperties", "containers");
}
this.containers = containers;
return this;
}
@CustomType.Setter
public Builder dnsPolicy(@Nullable String dnsPolicy) {
this.dnsPolicy = dnsPolicy;
return this;
}
@CustomType.Setter
public Builder hostNetwork(@Nullable Boolean hostNetwork) {
this.hostNetwork = hostNetwork;
return this;
}
@CustomType.Setter
public Builder imagePullSecrets(@Nullable List imagePullSecrets) {
this.imagePullSecrets = imagePullSecrets;
return this;
}
public Builder imagePullSecrets(JobDefinitionEksPropertiesPodPropertiesImagePullSecret... imagePullSecrets) {
return imagePullSecrets(List.of(imagePullSecrets));
}
@CustomType.Setter
public Builder initContainers(@Nullable List initContainers) {
this.initContainers = initContainers;
return this;
}
public Builder initContainers(JobDefinitionEksPropertiesPodPropertiesInitContainer... initContainers) {
return initContainers(List.of(initContainers));
}
@CustomType.Setter
public Builder metadata(@Nullable JobDefinitionEksPropertiesPodPropertiesMetadata metadata) {
this.metadata = metadata;
return this;
}
@CustomType.Setter
public Builder serviceAccountName(@Nullable String serviceAccountName) {
this.serviceAccountName = serviceAccountName;
return this;
}
@CustomType.Setter
public Builder shareProcessNamespace(@Nullable Boolean shareProcessNamespace) {
this.shareProcessNamespace = shareProcessNamespace;
return this;
}
@CustomType.Setter
public Builder volumes(@Nullable List volumes) {
this.volumes = volumes;
return this;
}
public Builder volumes(JobDefinitionEksPropertiesPodPropertiesVolume... volumes) {
return volumes(List.of(volumes));
}
public JobDefinitionEksPropertiesPodProperties build() {
final var _resultValue = new JobDefinitionEksPropertiesPodProperties();
_resultValue.containers = containers;
_resultValue.dnsPolicy = dnsPolicy;
_resultValue.hostNetwork = hostNetwork;
_resultValue.imagePullSecrets = imagePullSecrets;
_resultValue.initContainers = initContainers;
_resultValue.metadata = metadata;
_resultValue.serviceAccountName = serviceAccountName;
_resultValue.shareProcessNamespace = shareProcessNamespace;
_resultValue.volumes = volumes;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy