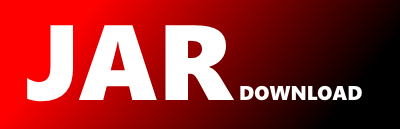
com.pulumi.aws.bedrock.ProvisionedModelThroughputArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.bedrock;
import com.pulumi.aws.bedrock.inputs.ProvisionedModelThroughputTimeoutsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProvisionedModelThroughputArgs extends com.pulumi.resources.ResourceArgs {
public static final ProvisionedModelThroughputArgs Empty = new ProvisionedModelThroughputArgs();
/**
* Commitment duration requested for the Provisioned Throughput. For custom models, you can purchase on-demand Provisioned Throughput by omitting this argument. Valid values: `OneMonth`, `SixMonths`.
*
*/
@Import(name="commitmentDuration")
private @Nullable Output commitmentDuration;
/**
* @return Commitment duration requested for the Provisioned Throughput. For custom models, you can purchase on-demand Provisioned Throughput by omitting this argument. Valid values: `OneMonth`, `SixMonths`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy