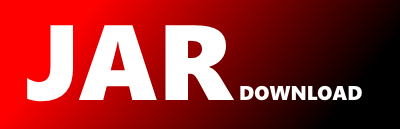
com.pulumi.aws.cfg.RemediationConfigurationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cfg;
import com.pulumi.aws.cfg.inputs.RemediationConfigurationExecutionControlsArgs;
import com.pulumi.aws.cfg.inputs.RemediationConfigurationParameterArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class RemediationConfigurationArgs extends com.pulumi.resources.ResourceArgs {
public static final RemediationConfigurationArgs Empty = new RemediationConfigurationArgs();
/**
* Remediation is triggered automatically if `true`.
*
*/
@Import(name="automatic")
private @Nullable Output automatic;
/**
* @return Remediation is triggered automatically if `true`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy