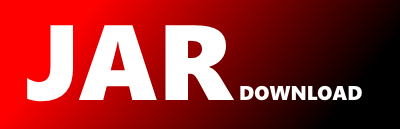
com.pulumi.aws.codebuild.outputs.ProjectFileSystemLocation Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.codebuild.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ProjectFileSystemLocation {
/**
* @return The name used to access a file system created by Amazon EFS. CodeBuild creates an environment variable by appending the identifier in all capital letters to CODEBUILD\_. For example, if you specify my-efs for identifier, a new environment variable is create named CODEBUILD_MY-EFS.
*
*/
private @Nullable String identifier;
/**
* @return A string that specifies the location of the file system created by Amazon EFS. Its format is `efs-dns-name:/directory-path`.
*
*/
private @Nullable String location;
/**
* @return The mount options for a file system created by AWS EFS.
*
*/
private @Nullable String mountOptions;
/**
* @return The location in the container where you mount the file system.
*
*/
private @Nullable String mountPoint;
/**
* @return The type of the file system. The one supported type is `EFS`.
*
*/
private @Nullable String type;
private ProjectFileSystemLocation() {}
/**
* @return The name used to access a file system created by Amazon EFS. CodeBuild creates an environment variable by appending the identifier in all capital letters to CODEBUILD\_. For example, if you specify my-efs for identifier, a new environment variable is create named CODEBUILD_MY-EFS.
*
*/
public Optional identifier() {
return Optional.ofNullable(this.identifier);
}
/**
* @return A string that specifies the location of the file system created by Amazon EFS. Its format is `efs-dns-name:/directory-path`.
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return The mount options for a file system created by AWS EFS.
*
*/
public Optional mountOptions() {
return Optional.ofNullable(this.mountOptions);
}
/**
* @return The location in the container where you mount the file system.
*
*/
public Optional mountPoint() {
return Optional.ofNullable(this.mountPoint);
}
/**
* @return The type of the file system. The one supported type is `EFS`.
*
*/
public Optional type() {
return Optional.ofNullable(this.type);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ProjectFileSystemLocation defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String identifier;
private @Nullable String location;
private @Nullable String mountOptions;
private @Nullable String mountPoint;
private @Nullable String type;
public Builder() {}
public Builder(ProjectFileSystemLocation defaults) {
Objects.requireNonNull(defaults);
this.identifier = defaults.identifier;
this.location = defaults.location;
this.mountOptions = defaults.mountOptions;
this.mountPoint = defaults.mountPoint;
this.type = defaults.type;
}
@CustomType.Setter
public Builder identifier(@Nullable String identifier) {
this.identifier = identifier;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder mountOptions(@Nullable String mountOptions) {
this.mountOptions = mountOptions;
return this;
}
@CustomType.Setter
public Builder mountPoint(@Nullable String mountPoint) {
this.mountPoint = mountPoint;
return this;
}
@CustomType.Setter
public Builder type(@Nullable String type) {
this.type = type;
return this;
}
public ProjectFileSystemLocation build() {
final var _resultValue = new ProjectFileSystemLocation();
_resultValue.identifier = identifier;
_resultValue.location = location;
_resultValue.mountOptions = mountOptions;
_resultValue.mountPoint = mountPoint;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy