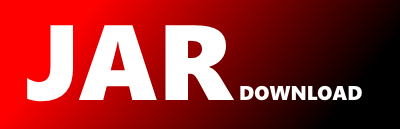
com.pulumi.aws.connect.outputs.GetRoutingProfileResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.connect.outputs;
import com.pulumi.aws.connect.outputs.GetRoutingProfileMediaConcurrency;
import com.pulumi.aws.connect.outputs.GetRoutingProfileQueueConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetRoutingProfileResult {
/**
* @return ARN of the Routing Profile.
*
*/
private String arn;
/**
* @return Specifies the default outbound queue for the Routing Profile.
*
*/
private String defaultOutboundQueueId;
/**
* @return Description of the Routing Profile.
*
*/
private String description;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String instanceId;
/**
* @return One or more `media_concurrencies` blocks that specify the channels that agents can handle in the Contact Control Panel (CCP) for this Routing Profile. The `media_concurrencies` block is documented below.
*
*/
private List mediaConcurrencies;
private String name;
/**
* @return One or more `queue_configs` blocks that specify the inbound queues associated with the routing profile. If no queue is added, the agent only can make outbound calls. The `queue_configs` block is documented below.
*
*/
private List queueConfigs;
private String routingProfileId;
/**
* @return Map of tags to assign to the Routing Profile.
*
*/
private Map tags;
private GetRoutingProfileResult() {}
/**
* @return ARN of the Routing Profile.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Specifies the default outbound queue for the Routing Profile.
*
*/
public String defaultOutboundQueueId() {
return this.defaultOutboundQueueId;
}
/**
* @return Description of the Routing Profile.
*
*/
public String description() {
return this.description;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String instanceId() {
return this.instanceId;
}
/**
* @return One or more `media_concurrencies` blocks that specify the channels that agents can handle in the Contact Control Panel (CCP) for this Routing Profile. The `media_concurrencies` block is documented below.
*
*/
public List mediaConcurrencies() {
return this.mediaConcurrencies;
}
public String name() {
return this.name;
}
/**
* @return One or more `queue_configs` blocks that specify the inbound queues associated with the routing profile. If no queue is added, the agent only can make outbound calls. The `queue_configs` block is documented below.
*
*/
public List queueConfigs() {
return this.queueConfigs;
}
public String routingProfileId() {
return this.routingProfileId;
}
/**
* @return Map of tags to assign to the Routing Profile.
*
*/
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetRoutingProfileResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private String defaultOutboundQueueId;
private String description;
private String id;
private String instanceId;
private List mediaConcurrencies;
private String name;
private List queueConfigs;
private String routingProfileId;
private Map tags;
public Builder() {}
public Builder(GetRoutingProfileResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.defaultOutboundQueueId = defaults.defaultOutboundQueueId;
this.description = defaults.description;
this.id = defaults.id;
this.instanceId = defaults.instanceId;
this.mediaConcurrencies = defaults.mediaConcurrencies;
this.name = defaults.name;
this.queueConfigs = defaults.queueConfigs;
this.routingProfileId = defaults.routingProfileId;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder defaultOutboundQueueId(String defaultOutboundQueueId) {
if (defaultOutboundQueueId == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "defaultOutboundQueueId");
}
this.defaultOutboundQueueId = defaultOutboundQueueId;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceId(String instanceId) {
if (instanceId == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "instanceId");
}
this.instanceId = instanceId;
return this;
}
@CustomType.Setter
public Builder mediaConcurrencies(List mediaConcurrencies) {
if (mediaConcurrencies == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "mediaConcurrencies");
}
this.mediaConcurrencies = mediaConcurrencies;
return this;
}
public Builder mediaConcurrencies(GetRoutingProfileMediaConcurrency... mediaConcurrencies) {
return mediaConcurrencies(List.of(mediaConcurrencies));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder queueConfigs(List queueConfigs) {
if (queueConfigs == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "queueConfigs");
}
this.queueConfigs = queueConfigs;
return this;
}
public Builder queueConfigs(GetRoutingProfileQueueConfig... queueConfigs) {
return queueConfigs(List.of(queueConfigs));
}
@CustomType.Setter
public Builder routingProfileId(String routingProfileId) {
if (routingProfileId == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "routingProfileId");
}
this.routingProfileId = routingProfileId;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetRoutingProfileResult", "tags");
}
this.tags = tags;
return this;
}
public GetRoutingProfileResult build() {
final var _resultValue = new GetRoutingProfileResult();
_resultValue.arn = arn;
_resultValue.defaultOutboundQueueId = defaultOutboundQueueId;
_resultValue.description = description;
_resultValue.id = id;
_resultValue.instanceId = instanceId;
_resultValue.mediaConcurrencies = mediaConcurrencies;
_resultValue.name = name;
_resultValue.queueConfigs = queueConfigs;
_resultValue.routingProfileId = routingProfileId;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy