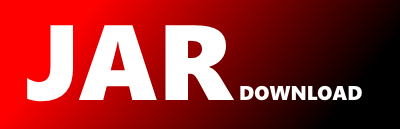
com.pulumi.aws.cur.outputs.GetReportDefinitionResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.cur.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetReportDefinitionResult {
/**
* @return A list of additional artifacts.
*
*/
private List additionalArtifacts;
/**
* @return A list of schema elements.
*
*/
private List additionalSchemaElements;
/**
* @return Preferred format for report.
*
*/
private String compression;
/**
* @return Preferred compression format for report.
*
*/
private String format;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return If true reports are updated after they have been finalized.
*
*/
private Boolean refreshClosedReports;
private String reportName;
/**
* @return Overwrite the previous version of each report or to deliver the report in addition to the previous versions.
*
*/
private String reportVersioning;
/**
* @return Name of customer S3 bucket.
*
*/
private String s3Bucket;
/**
* @return Preferred report path prefix.
*
*/
private String s3Prefix;
/**
* @return Region of customer S3 bucket.
*
*/
private String s3Region;
/**
* @return Map of key-value pairs assigned to the resource.
*
*/
private Map tags;
/**
* @return Frequency on which report data are measured and displayed.
*
*/
private String timeUnit;
private GetReportDefinitionResult() {}
/**
* @return A list of additional artifacts.
*
*/
public List additionalArtifacts() {
return this.additionalArtifacts;
}
/**
* @return A list of schema elements.
*
*/
public List additionalSchemaElements() {
return this.additionalSchemaElements;
}
/**
* @return Preferred format for report.
*
*/
public String compression() {
return this.compression;
}
/**
* @return Preferred compression format for report.
*
*/
public String format() {
return this.format;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return If true reports are updated after they have been finalized.
*
*/
public Boolean refreshClosedReports() {
return this.refreshClosedReports;
}
public String reportName() {
return this.reportName;
}
/**
* @return Overwrite the previous version of each report or to deliver the report in addition to the previous versions.
*
*/
public String reportVersioning() {
return this.reportVersioning;
}
/**
* @return Name of customer S3 bucket.
*
*/
public String s3Bucket() {
return this.s3Bucket;
}
/**
* @return Preferred report path prefix.
*
*/
public String s3Prefix() {
return this.s3Prefix;
}
/**
* @return Region of customer S3 bucket.
*
*/
public String s3Region() {
return this.s3Region;
}
/**
* @return Map of key-value pairs assigned to the resource.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Frequency on which report data are measured and displayed.
*
*/
public String timeUnit() {
return this.timeUnit;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReportDefinitionResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List additionalArtifacts;
private List additionalSchemaElements;
private String compression;
private String format;
private String id;
private Boolean refreshClosedReports;
private String reportName;
private String reportVersioning;
private String s3Bucket;
private String s3Prefix;
private String s3Region;
private Map tags;
private String timeUnit;
public Builder() {}
public Builder(GetReportDefinitionResult defaults) {
Objects.requireNonNull(defaults);
this.additionalArtifacts = defaults.additionalArtifacts;
this.additionalSchemaElements = defaults.additionalSchemaElements;
this.compression = defaults.compression;
this.format = defaults.format;
this.id = defaults.id;
this.refreshClosedReports = defaults.refreshClosedReports;
this.reportName = defaults.reportName;
this.reportVersioning = defaults.reportVersioning;
this.s3Bucket = defaults.s3Bucket;
this.s3Prefix = defaults.s3Prefix;
this.s3Region = defaults.s3Region;
this.tags = defaults.tags;
this.timeUnit = defaults.timeUnit;
}
@CustomType.Setter
public Builder additionalArtifacts(List additionalArtifacts) {
if (additionalArtifacts == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "additionalArtifacts");
}
this.additionalArtifacts = additionalArtifacts;
return this;
}
public Builder additionalArtifacts(String... additionalArtifacts) {
return additionalArtifacts(List.of(additionalArtifacts));
}
@CustomType.Setter
public Builder additionalSchemaElements(List additionalSchemaElements) {
if (additionalSchemaElements == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "additionalSchemaElements");
}
this.additionalSchemaElements = additionalSchemaElements;
return this;
}
public Builder additionalSchemaElements(String... additionalSchemaElements) {
return additionalSchemaElements(List.of(additionalSchemaElements));
}
@CustomType.Setter
public Builder compression(String compression) {
if (compression == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "compression");
}
this.compression = compression;
return this;
}
@CustomType.Setter
public Builder format(String format) {
if (format == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "format");
}
this.format = format;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder refreshClosedReports(Boolean refreshClosedReports) {
if (refreshClosedReports == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "refreshClosedReports");
}
this.refreshClosedReports = refreshClosedReports;
return this;
}
@CustomType.Setter
public Builder reportName(String reportName) {
if (reportName == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "reportName");
}
this.reportName = reportName;
return this;
}
@CustomType.Setter
public Builder reportVersioning(String reportVersioning) {
if (reportVersioning == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "reportVersioning");
}
this.reportVersioning = reportVersioning;
return this;
}
@CustomType.Setter
public Builder s3Bucket(String s3Bucket) {
if (s3Bucket == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "s3Bucket");
}
this.s3Bucket = s3Bucket;
return this;
}
@CustomType.Setter
public Builder s3Prefix(String s3Prefix) {
if (s3Prefix == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "s3Prefix");
}
this.s3Prefix = s3Prefix;
return this;
}
@CustomType.Setter
public Builder s3Region(String s3Region) {
if (s3Region == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "s3Region");
}
this.s3Region = s3Region;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder timeUnit(String timeUnit) {
if (timeUnit == null) {
throw new MissingRequiredPropertyException("GetReportDefinitionResult", "timeUnit");
}
this.timeUnit = timeUnit;
return this;
}
public GetReportDefinitionResult build() {
final var _resultValue = new GetReportDefinitionResult();
_resultValue.additionalArtifacts = additionalArtifacts;
_resultValue.additionalSchemaElements = additionalSchemaElements;
_resultValue.compression = compression;
_resultValue.format = format;
_resultValue.id = id;
_resultValue.refreshClosedReports = refreshClosedReports;
_resultValue.reportName = reportName;
_resultValue.reportVersioning = reportVersioning;
_resultValue.s3Bucket = s3Bucket;
_resultValue.s3Prefix = s3Prefix;
_resultValue.s3Region = s3Region;
_resultValue.tags = tags;
_resultValue.timeUnit = timeUnit;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy