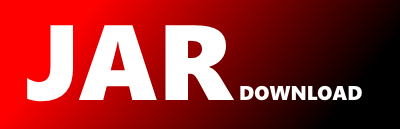
com.pulumi.aws.datasync.LocationFsxOntapFileSystemArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.datasync;
import com.pulumi.aws.datasync.inputs.LocationFsxOntapFileSystemProtocolArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class LocationFsxOntapFileSystemArgs extends com.pulumi.resources.ResourceArgs {
public static final LocationFsxOntapFileSystemArgs Empty = new LocationFsxOntapFileSystemArgs();
/**
* The data transfer protocol that DataSync uses to access your Amazon FSx file system. See Protocol below.
*
*/
@Import(name="protocol", required=true)
private Output protocol;
/**
* @return The data transfer protocol that DataSync uses to access your Amazon FSx file system. See Protocol below.
*
*/
public Output protocol() {
return this.protocol;
}
/**
* The security groups that provide access to your file system's preferred subnet. The security groups must allow outbbound traffic on the following ports (depending on the protocol you use):
* * Network File System (NFS): TCP ports 111, 635, and 2049
* * Server Message Block (SMB): TCP port 445
*
*/
@Import(name="securityGroupArns", required=true)
private Output> securityGroupArns;
/**
* @return The security groups that provide access to your file system's preferred subnet. The security groups must allow outbbound traffic on the following ports (depending on the protocol you use):
* * Network File System (NFS): TCP ports 111, 635, and 2049
* * Server Message Block (SMB): TCP port 445
*
*/
public Output> securityGroupArns() {
return this.securityGroupArns;
}
/**
* The ARN of the SVM in your file system where you want to copy data to of from.
*
* The following arguments are optional:
*
*/
@Import(name="storageVirtualMachineArn", required=true)
private Output storageVirtualMachineArn;
/**
* @return The ARN of the SVM in your file system where you want to copy data to of from.
*
* The following arguments are optional:
*
*/
public Output storageVirtualMachineArn() {
return this.storageVirtualMachineArn;
}
/**
* Path to the file share in the SVM where you'll copy your data. You can specify a junction path (also known as a mount point), qtree path (for NFS file shares), or share name (for SMB file shares) (e.g. `/vol1`, `/vol1/tree1`, `share1`).
*
*/
@Import(name="subdirectory")
private @Nullable Output subdirectory;
/**
* @return Path to the file share in the SVM where you'll copy your data. You can specify a junction path (also known as a mount point), qtree path (for NFS file shares), or share name (for SMB file shares) (e.g. `/vol1`, `/vol1/tree1`, `share1`).
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy