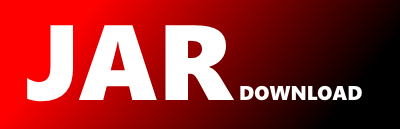
com.pulumi.aws.datasync.S3LocationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.datasync;
import com.pulumi.aws.datasync.inputs.S3LocationS3ConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class S3LocationArgs extends com.pulumi.resources.ResourceArgs {
public static final S3LocationArgs Empty = new S3LocationArgs();
/**
* A list of DataSync Agent ARNs with which this location will be associated.
*
*/
@Import(name="agentArns")
private @Nullable Output> agentArns;
/**
* @return A list of DataSync Agent ARNs with which this location will be associated.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy