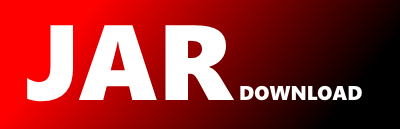
com.pulumi.aws.datasync.inputs.TaskTaskReportConfigReportOverridesArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.datasync.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TaskTaskReportConfigReportOverridesArgs extends com.pulumi.resources.ResourceArgs {
public static final TaskTaskReportConfigReportOverridesArgs Empty = new TaskTaskReportConfigReportOverridesArgs();
/**
* Specifies the level of reporting for the files, objects, and directories that DataSync attempted to delete in your destination location. This only applies if you configure your task to delete data in the destination that isn't in the source. Valid values: `ERRORS_ONLY` and `SUCCESSES_AND_ERRORS`.
*
*/
@Import(name="deletedOverride")
private @Nullable Output deletedOverride;
/**
* @return Specifies the level of reporting for the files, objects, and directories that DataSync attempted to delete in your destination location. This only applies if you configure your task to delete data in the destination that isn't in the source. Valid values: `ERRORS_ONLY` and `SUCCESSES_AND_ERRORS`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy