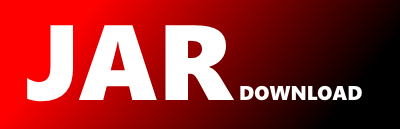
com.pulumi.aws.dax.ClusterArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dax;
import com.pulumi.aws.dax.inputs.ClusterServerSideEncryptionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterArgs extends com.pulumi.resources.ResourceArgs {
public static final ClusterArgs Empty = new ClusterArgs();
/**
* List of Availability Zones in which the
* nodes will be created
*
*/
@Import(name="availabilityZones")
private @Nullable Output> availabilityZones;
/**
* @return List of Availability Zones in which the
* nodes will be created
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy