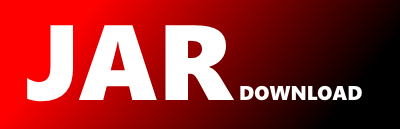
com.pulumi.aws.directconnect.HostedConnection Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directconnect;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.directconnect.HostedConnectionArgs;
import com.pulumi.aws.directconnect.inputs.HostedConnectionState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import javax.annotation.Nullable;
/**
* Provides a hosted connection on the specified interconnect or a link aggregation group (LAG) of interconnects. Intended for use by AWS Direct Connect Partners only.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.directconnect.HostedConnection;
* import com.pulumi.aws.directconnect.HostedConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var hosted = new HostedConnection("hosted", HostedConnectionArgs.builder()
* .connectionId("dxcon-ffabc123")
* .bandwidth("100Mbps")
* .name("tf-dx-hosted-connection")
* .ownerAccountId("123456789012")
* .vlan(1)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
*/
@ResourceType(type="aws:directconnect/hostedConnection:HostedConnection")
public class HostedConnection extends com.pulumi.resources.CustomResource {
/**
* The Direct Connect endpoint on which the physical connection terminates.
*
*/
@Export(name="awsDevice", refs={String.class}, tree="[0]")
private Output awsDevice;
/**
* @return The Direct Connect endpoint on which the physical connection terminates.
*
*/
public Output awsDevice() {
return this.awsDevice;
}
/**
* The bandwidth of the connection. Valid values for dedicated connections: 1Gbps, 10Gbps. Valid values for hosted connections: 50Mbps, 100Mbps, 200Mbps, 300Mbps, 400Mbps, 500Mbps, 1Gbps, 2Gbps, 5Gbps and 10Gbps. Case sensitive.
*
*/
@Export(name="bandwidth", refs={String.class}, tree="[0]")
private Output bandwidth;
/**
* @return The bandwidth of the connection. Valid values for dedicated connections: 1Gbps, 10Gbps. Valid values for hosted connections: 50Mbps, 100Mbps, 200Mbps, 300Mbps, 400Mbps, 500Mbps, 1Gbps, 2Gbps, 5Gbps and 10Gbps. Case sensitive.
*
*/
public Output bandwidth() {
return this.bandwidth;
}
/**
* The ID of the interconnect or LAG.
*
*/
@Export(name="connectionId", refs={String.class}, tree="[0]")
private Output connectionId;
/**
* @return The ID of the interconnect or LAG.
*
*/
public Output connectionId() {
return this.connectionId;
}
/**
* Indicates whether the connection supports a secondary BGP peer in the same address family (IPv4/IPv6).
*
*/
@Export(name="hasLogicalRedundancy", refs={String.class}, tree="[0]")
private Output hasLogicalRedundancy;
/**
* @return Indicates whether the connection supports a secondary BGP peer in the same address family (IPv4/IPv6).
*
*/
public Output hasLogicalRedundancy() {
return this.hasLogicalRedundancy;
}
/**
* Boolean value representing if jumbo frames have been enabled for this connection.
*
*/
@Export(name="jumboFrameCapable", refs={Boolean.class}, tree="[0]")
private Output jumboFrameCapable;
/**
* @return Boolean value representing if jumbo frames have been enabled for this connection.
*
*/
public Output jumboFrameCapable() {
return this.jumboFrameCapable;
}
/**
* The ID of the LAG.
*
*/
@Export(name="lagId", refs={String.class}, tree="[0]")
private Output lagId;
/**
* @return The ID of the LAG.
*
*/
public Output lagId() {
return this.lagId;
}
/**
* The time of the most recent call to [DescribeLoa](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_DescribeLoa.html) for this connection.
*
*/
@Export(name="loaIssueTime", refs={String.class}, tree="[0]")
private Output loaIssueTime;
/**
* @return The time of the most recent call to [DescribeLoa](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_DescribeLoa.html) for this connection.
*
*/
public Output loaIssueTime() {
return this.loaIssueTime;
}
/**
* The location of the connection.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The location of the connection.
*
*/
public Output location() {
return this.location;
}
/**
* The name of the connection.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the connection.
*
*/
public Output name() {
return this.name;
}
/**
* The ID of the AWS account of the customer for the connection.
*
*/
@Export(name="ownerAccountId", refs={String.class}, tree="[0]")
private Output ownerAccountId;
/**
* @return The ID of the AWS account of the customer for the connection.
*
*/
public Output ownerAccountId() {
return this.ownerAccountId;
}
/**
* The name of the AWS Direct Connect service provider associated with the connection.
*
*/
@Export(name="partnerName", refs={String.class}, tree="[0]")
private Output partnerName;
/**
* @return The name of the AWS Direct Connect service provider associated with the connection.
*
*/
public Output partnerName() {
return this.partnerName;
}
/**
* The name of the service provider associated with the connection.
*
*/
@Export(name="providerName", refs={String.class}, tree="[0]")
private Output providerName;
/**
* @return The name of the service provider associated with the connection.
*
*/
public Output providerName() {
return this.providerName;
}
/**
* The AWS Region where the connection is located.
*
*/
@Export(name="region", refs={String.class}, tree="[0]")
private Output region;
/**
* @return The AWS Region where the connection is located.
*
*/
public Output region() {
return this.region;
}
/**
* The state of the connection. Possible values include: ordering, requested, pending, available, down, deleting, deleted, rejected, unknown. See [AllocateHostedConnection](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_AllocateHostedConnection.html) for a description of each connection state.
*
*/
@Export(name="state", refs={String.class}, tree="[0]")
private Output state;
/**
* @return The state of the connection. Possible values include: ordering, requested, pending, available, down, deleting, deleted, rejected, unknown. See [AllocateHostedConnection](https://docs.aws.amazon.com/directconnect/latest/APIReference/API_AllocateHostedConnection.html) for a description of each connection state.
*
*/
public Output state() {
return this.state;
}
/**
* The dedicated VLAN provisioned to the hosted connection.
*
*/
@Export(name="vlan", refs={Integer.class}, tree="[0]")
private Output vlan;
/**
* @return The dedicated VLAN provisioned to the hosted connection.
*
*/
public Output vlan() {
return this.vlan;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public HostedConnection(java.lang.String name) {
this(name, HostedConnectionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public HostedConnection(java.lang.String name, HostedConnectionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public HostedConnection(java.lang.String name, HostedConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:directconnect/hostedConnection:HostedConnection", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private HostedConnection(java.lang.String name, Output id, @Nullable HostedConnectionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:directconnect/hostedConnection:HostedConnection", name, state, makeResourceOptions(options, id), false);
}
private static HostedConnectionArgs makeArgs(HostedConnectionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? HostedConnectionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static HostedConnection get(java.lang.String name, Output id, @Nullable HostedConnectionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new HostedConnection(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy