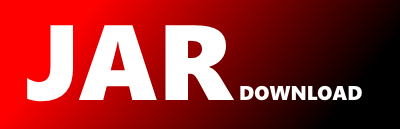
com.pulumi.aws.directoryservice.inputs.DirectoryState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.directoryservice.inputs;
import com.pulumi.aws.directoryservice.inputs.DirectoryConnectSettingsArgs;
import com.pulumi.aws.directoryservice.inputs.DirectoryVpcSettingsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DirectoryState extends com.pulumi.resources.ResourceArgs {
public static final DirectoryState Empty = new DirectoryState();
/**
* The access URL for the directory, such as `http://alias.awsapps.com`.
*
*/
@Import(name="accessUrl")
private @Nullable Output accessUrl;
/**
* @return The access URL for the directory, such as `http://alias.awsapps.com`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy