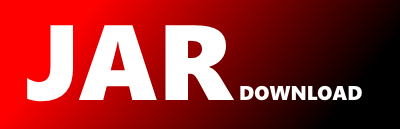
com.pulumi.aws.dms.outputs.GetEndpointResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dms.outputs;
import com.pulumi.aws.dms.outputs.GetEndpointElasticsearchSetting;
import com.pulumi.aws.dms.outputs.GetEndpointKafkaSetting;
import com.pulumi.aws.dms.outputs.GetEndpointKinesisSetting;
import com.pulumi.aws.dms.outputs.GetEndpointMongodbSetting;
import com.pulumi.aws.dms.outputs.GetEndpointPostgresSetting;
import com.pulumi.aws.dms.outputs.GetEndpointRedisSetting;
import com.pulumi.aws.dms.outputs.GetEndpointRedshiftSetting;
import com.pulumi.aws.dms.outputs.GetEndpointS3Setting;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetEndpointResult {
private String certificateArn;
private String databaseName;
private List elasticsearchSettings;
private String endpointArn;
private String endpointId;
private String endpointType;
private String engineName;
private String extraConnectionAttributes;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private List kafkaSettings;
private List kinesisSettings;
private String kmsKeyArn;
private List mongodbSettings;
private String password;
private Integer port;
private List postgresSettings;
private List redisSettings;
private List redshiftSettings;
private List s3Settings;
private String secretsManagerAccessRoleArn;
private String secretsManagerArn;
private String serverName;
private String serviceAccessRole;
private String sslMode;
private Map tags;
private String username;
private GetEndpointResult() {}
public String certificateArn() {
return this.certificateArn;
}
public String databaseName() {
return this.databaseName;
}
public List elasticsearchSettings() {
return this.elasticsearchSettings;
}
public String endpointArn() {
return this.endpointArn;
}
public String endpointId() {
return this.endpointId;
}
public String endpointType() {
return this.endpointType;
}
public String engineName() {
return this.engineName;
}
public String extraConnectionAttributes() {
return this.extraConnectionAttributes;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public List kafkaSettings() {
return this.kafkaSettings;
}
public List kinesisSettings() {
return this.kinesisSettings;
}
public String kmsKeyArn() {
return this.kmsKeyArn;
}
public List mongodbSettings() {
return this.mongodbSettings;
}
public String password() {
return this.password;
}
public Integer port() {
return this.port;
}
public List postgresSettings() {
return this.postgresSettings;
}
public List redisSettings() {
return this.redisSettings;
}
public List redshiftSettings() {
return this.redshiftSettings;
}
public List s3Settings() {
return this.s3Settings;
}
public String secretsManagerAccessRoleArn() {
return this.secretsManagerAccessRoleArn;
}
public String secretsManagerArn() {
return this.secretsManagerArn;
}
public String serverName() {
return this.serverName;
}
public String serviceAccessRole() {
return this.serviceAccessRole;
}
public String sslMode() {
return this.sslMode;
}
public Map tags() {
return this.tags;
}
public String username() {
return this.username;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEndpointResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String certificateArn;
private String databaseName;
private List elasticsearchSettings;
private String endpointArn;
private String endpointId;
private String endpointType;
private String engineName;
private String extraConnectionAttributes;
private String id;
private List kafkaSettings;
private List kinesisSettings;
private String kmsKeyArn;
private List mongodbSettings;
private String password;
private Integer port;
private List postgresSettings;
private List redisSettings;
private List redshiftSettings;
private List s3Settings;
private String secretsManagerAccessRoleArn;
private String secretsManagerArn;
private String serverName;
private String serviceAccessRole;
private String sslMode;
private Map tags;
private String username;
public Builder() {}
public Builder(GetEndpointResult defaults) {
Objects.requireNonNull(defaults);
this.certificateArn = defaults.certificateArn;
this.databaseName = defaults.databaseName;
this.elasticsearchSettings = defaults.elasticsearchSettings;
this.endpointArn = defaults.endpointArn;
this.endpointId = defaults.endpointId;
this.endpointType = defaults.endpointType;
this.engineName = defaults.engineName;
this.extraConnectionAttributes = defaults.extraConnectionAttributes;
this.id = defaults.id;
this.kafkaSettings = defaults.kafkaSettings;
this.kinesisSettings = defaults.kinesisSettings;
this.kmsKeyArn = defaults.kmsKeyArn;
this.mongodbSettings = defaults.mongodbSettings;
this.password = defaults.password;
this.port = defaults.port;
this.postgresSettings = defaults.postgresSettings;
this.redisSettings = defaults.redisSettings;
this.redshiftSettings = defaults.redshiftSettings;
this.s3Settings = defaults.s3Settings;
this.secretsManagerAccessRoleArn = defaults.secretsManagerAccessRoleArn;
this.secretsManagerArn = defaults.secretsManagerArn;
this.serverName = defaults.serverName;
this.serviceAccessRole = defaults.serviceAccessRole;
this.sslMode = defaults.sslMode;
this.tags = defaults.tags;
this.username = defaults.username;
}
@CustomType.Setter
public Builder certificateArn(String certificateArn) {
if (certificateArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "certificateArn");
}
this.certificateArn = certificateArn;
return this;
}
@CustomType.Setter
public Builder databaseName(String databaseName) {
if (databaseName == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "databaseName");
}
this.databaseName = databaseName;
return this;
}
@CustomType.Setter
public Builder elasticsearchSettings(List elasticsearchSettings) {
if (elasticsearchSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "elasticsearchSettings");
}
this.elasticsearchSettings = elasticsearchSettings;
return this;
}
public Builder elasticsearchSettings(GetEndpointElasticsearchSetting... elasticsearchSettings) {
return elasticsearchSettings(List.of(elasticsearchSettings));
}
@CustomType.Setter
public Builder endpointArn(String endpointArn) {
if (endpointArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "endpointArn");
}
this.endpointArn = endpointArn;
return this;
}
@CustomType.Setter
public Builder endpointId(String endpointId) {
if (endpointId == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "endpointId");
}
this.endpointId = endpointId;
return this;
}
@CustomType.Setter
public Builder endpointType(String endpointType) {
if (endpointType == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "endpointType");
}
this.endpointType = endpointType;
return this;
}
@CustomType.Setter
public Builder engineName(String engineName) {
if (engineName == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "engineName");
}
this.engineName = engineName;
return this;
}
@CustomType.Setter
public Builder extraConnectionAttributes(String extraConnectionAttributes) {
if (extraConnectionAttributes == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "extraConnectionAttributes");
}
this.extraConnectionAttributes = extraConnectionAttributes;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kafkaSettings(List kafkaSettings) {
if (kafkaSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "kafkaSettings");
}
this.kafkaSettings = kafkaSettings;
return this;
}
public Builder kafkaSettings(GetEndpointKafkaSetting... kafkaSettings) {
return kafkaSettings(List.of(kafkaSettings));
}
@CustomType.Setter
public Builder kinesisSettings(List kinesisSettings) {
if (kinesisSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "kinesisSettings");
}
this.kinesisSettings = kinesisSettings;
return this;
}
public Builder kinesisSettings(GetEndpointKinesisSetting... kinesisSettings) {
return kinesisSettings(List.of(kinesisSettings));
}
@CustomType.Setter
public Builder kmsKeyArn(String kmsKeyArn) {
if (kmsKeyArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "kmsKeyArn");
}
this.kmsKeyArn = kmsKeyArn;
return this;
}
@CustomType.Setter
public Builder mongodbSettings(List mongodbSettings) {
if (mongodbSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "mongodbSettings");
}
this.mongodbSettings = mongodbSettings;
return this;
}
public Builder mongodbSettings(GetEndpointMongodbSetting... mongodbSettings) {
return mongodbSettings(List.of(mongodbSettings));
}
@CustomType.Setter
public Builder password(String password) {
if (password == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "password");
}
this.password = password;
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder postgresSettings(List postgresSettings) {
if (postgresSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "postgresSettings");
}
this.postgresSettings = postgresSettings;
return this;
}
public Builder postgresSettings(GetEndpointPostgresSetting... postgresSettings) {
return postgresSettings(List.of(postgresSettings));
}
@CustomType.Setter
public Builder redisSettings(List redisSettings) {
if (redisSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "redisSettings");
}
this.redisSettings = redisSettings;
return this;
}
public Builder redisSettings(GetEndpointRedisSetting... redisSettings) {
return redisSettings(List.of(redisSettings));
}
@CustomType.Setter
public Builder redshiftSettings(List redshiftSettings) {
if (redshiftSettings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "redshiftSettings");
}
this.redshiftSettings = redshiftSettings;
return this;
}
public Builder redshiftSettings(GetEndpointRedshiftSetting... redshiftSettings) {
return redshiftSettings(List.of(redshiftSettings));
}
@CustomType.Setter
public Builder s3Settings(List s3Settings) {
if (s3Settings == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "s3Settings");
}
this.s3Settings = s3Settings;
return this;
}
public Builder s3Settings(GetEndpointS3Setting... s3Settings) {
return s3Settings(List.of(s3Settings));
}
@CustomType.Setter
public Builder secretsManagerAccessRoleArn(String secretsManagerAccessRoleArn) {
if (secretsManagerAccessRoleArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "secretsManagerAccessRoleArn");
}
this.secretsManagerAccessRoleArn = secretsManagerAccessRoleArn;
return this;
}
@CustomType.Setter
public Builder secretsManagerArn(String secretsManagerArn) {
if (secretsManagerArn == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "secretsManagerArn");
}
this.secretsManagerArn = secretsManagerArn;
return this;
}
@CustomType.Setter
public Builder serverName(String serverName) {
if (serverName == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "serverName");
}
this.serverName = serverName;
return this;
}
@CustomType.Setter
public Builder serviceAccessRole(String serviceAccessRole) {
if (serviceAccessRole == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "serviceAccessRole");
}
this.serviceAccessRole = serviceAccessRole;
return this;
}
@CustomType.Setter
public Builder sslMode(String sslMode) {
if (sslMode == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "sslMode");
}
this.sslMode = sslMode;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder username(String username) {
if (username == null) {
throw new MissingRequiredPropertyException("GetEndpointResult", "username");
}
this.username = username;
return this;
}
public GetEndpointResult build() {
final var _resultValue = new GetEndpointResult();
_resultValue.certificateArn = certificateArn;
_resultValue.databaseName = databaseName;
_resultValue.elasticsearchSettings = elasticsearchSettings;
_resultValue.endpointArn = endpointArn;
_resultValue.endpointId = endpointId;
_resultValue.endpointType = endpointType;
_resultValue.engineName = engineName;
_resultValue.extraConnectionAttributes = extraConnectionAttributes;
_resultValue.id = id;
_resultValue.kafkaSettings = kafkaSettings;
_resultValue.kinesisSettings = kinesisSettings;
_resultValue.kmsKeyArn = kmsKeyArn;
_resultValue.mongodbSettings = mongodbSettings;
_resultValue.password = password;
_resultValue.port = port;
_resultValue.postgresSettings = postgresSettings;
_resultValue.redisSettings = redisSettings;
_resultValue.redshiftSettings = redshiftSettings;
_resultValue.s3Settings = s3Settings;
_resultValue.secretsManagerAccessRoleArn = secretsManagerAccessRoleArn;
_resultValue.secretsManagerArn = secretsManagerArn;
_resultValue.serverName = serverName;
_resultValue.serviceAccessRole = serviceAccessRole;
_resultValue.sslMode = sslMode;
_resultValue.tags = tags;
_resultValue.username = username;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy