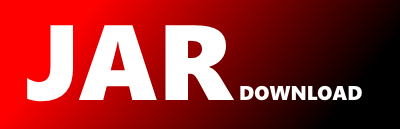
com.pulumi.aws.dms.outputs.GetReplicationInstanceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.dms.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetReplicationInstanceResult {
/**
* @return The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*/
private Integer allocatedStorage;
/**
* @return Indicates that minor engine upgrades will be applied automatically to the replication instance during the maintenance window.
*
*/
private Boolean autoMinorVersionUpgrade;
/**
* @return The EC2 Availability Zone that the replication instance will be created in.
*
*/
private String availabilityZone;
/**
* @return The engine version number of the replication instance.
*
*/
private String engineVersion;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return The Amazon Resource Name (ARN) for the KMS key used to encrypt the connection parameters.
*
*/
private String kmsKeyArn;
/**
* @return Specifies if the replication instance is a multi-az deployment.
*
*/
private Boolean multiAz;
/**
* @return The type of IP address protocol used by the replication instance.
*
*/
private String networkType;
/**
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*/
private String preferredMaintenanceWindow;
/**
* @return Specifies the accessibility options for the replication instance. A value of true represents an instance with a public IP address. A value of false represents an instance with a private IP address.
*
*/
private Boolean publiclyAccessible;
/**
* @return The Amazon Resource Name (ARN) of the replication instance.
*
*/
private String replicationInstanceArn;
/**
* @return The compute and memory capacity of the replication instance as specified by the replication instance class. See [AWS DMS User Guide](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.Types.html) for information on instance classes.
*
*/
private String replicationInstanceClass;
private String replicationInstanceId;
/**
* @return A list of the private IP addresses of the replication instance.
*
*/
private List replicationInstancePrivateIps;
/**
* @return A list of the public IP addresses of the replication instance.
*
*/
private List replicationInstancePublicIps;
/**
* @return A subnet group to associate with the replication instance.
*
*/
private String replicationSubnetGroupId;
private Map tags;
/**
* @return A set of VPC security group IDs that are used with the replication instance.
*
*/
private List vpcSecurityGroupIds;
private GetReplicationInstanceResult() {}
/**
* @return The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*/
public Integer allocatedStorage() {
return this.allocatedStorage;
}
/**
* @return Indicates that minor engine upgrades will be applied automatically to the replication instance during the maintenance window.
*
*/
public Boolean autoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
* @return The EC2 Availability Zone that the replication instance will be created in.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return The engine version number of the replication instance.
*
*/
public String engineVersion() {
return this.engineVersion;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return The Amazon Resource Name (ARN) for the KMS key used to encrypt the connection parameters.
*
*/
public String kmsKeyArn() {
return this.kmsKeyArn;
}
/**
* @return Specifies if the replication instance is a multi-az deployment.
*
*/
public Boolean multiAz() {
return this.multiAz;
}
/**
* @return The type of IP address protocol used by the replication instance.
*
*/
public String networkType() {
return this.networkType;
}
/**
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*/
public String preferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
* @return Specifies the accessibility options for the replication instance. A value of true represents an instance with a public IP address. A value of false represents an instance with a private IP address.
*
*/
public Boolean publiclyAccessible() {
return this.publiclyAccessible;
}
/**
* @return The Amazon Resource Name (ARN) of the replication instance.
*
*/
public String replicationInstanceArn() {
return this.replicationInstanceArn;
}
/**
* @return The compute and memory capacity of the replication instance as specified by the replication instance class. See [AWS DMS User Guide](https://docs.aws.amazon.com/dms/latest/userguide/CHAP_ReplicationInstance.Types.html) for information on instance classes.
*
*/
public String replicationInstanceClass() {
return this.replicationInstanceClass;
}
public String replicationInstanceId() {
return this.replicationInstanceId;
}
/**
* @return A list of the private IP addresses of the replication instance.
*
*/
public List replicationInstancePrivateIps() {
return this.replicationInstancePrivateIps;
}
/**
* @return A list of the public IP addresses of the replication instance.
*
*/
public List replicationInstancePublicIps() {
return this.replicationInstancePublicIps;
}
/**
* @return A subnet group to associate with the replication instance.
*
*/
public String replicationSubnetGroupId() {
return this.replicationSubnetGroupId;
}
public Map tags() {
return this.tags;
}
/**
* @return A set of VPC security group IDs that are used with the replication instance.
*
*/
public List vpcSecurityGroupIds() {
return this.vpcSecurityGroupIds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetReplicationInstanceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer allocatedStorage;
private Boolean autoMinorVersionUpgrade;
private String availabilityZone;
private String engineVersion;
private String id;
private String kmsKeyArn;
private Boolean multiAz;
private String networkType;
private String preferredMaintenanceWindow;
private Boolean publiclyAccessible;
private String replicationInstanceArn;
private String replicationInstanceClass;
private String replicationInstanceId;
private List replicationInstancePrivateIps;
private List replicationInstancePublicIps;
private String replicationSubnetGroupId;
private Map tags;
private List vpcSecurityGroupIds;
public Builder() {}
public Builder(GetReplicationInstanceResult defaults) {
Objects.requireNonNull(defaults);
this.allocatedStorage = defaults.allocatedStorage;
this.autoMinorVersionUpgrade = defaults.autoMinorVersionUpgrade;
this.availabilityZone = defaults.availabilityZone;
this.engineVersion = defaults.engineVersion;
this.id = defaults.id;
this.kmsKeyArn = defaults.kmsKeyArn;
this.multiAz = defaults.multiAz;
this.networkType = defaults.networkType;
this.preferredMaintenanceWindow = defaults.preferredMaintenanceWindow;
this.publiclyAccessible = defaults.publiclyAccessible;
this.replicationInstanceArn = defaults.replicationInstanceArn;
this.replicationInstanceClass = defaults.replicationInstanceClass;
this.replicationInstanceId = defaults.replicationInstanceId;
this.replicationInstancePrivateIps = defaults.replicationInstancePrivateIps;
this.replicationInstancePublicIps = defaults.replicationInstancePublicIps;
this.replicationSubnetGroupId = defaults.replicationSubnetGroupId;
this.tags = defaults.tags;
this.vpcSecurityGroupIds = defaults.vpcSecurityGroupIds;
}
@CustomType.Setter
public Builder allocatedStorage(Integer allocatedStorage) {
if (allocatedStorage == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "allocatedStorage");
}
this.allocatedStorage = allocatedStorage;
return this;
}
@CustomType.Setter
public Builder autoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
if (autoMinorVersionUpgrade == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "autoMinorVersionUpgrade");
}
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
return this;
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder engineVersion(String engineVersion) {
if (engineVersion == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "engineVersion");
}
this.engineVersion = engineVersion;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kmsKeyArn(String kmsKeyArn) {
if (kmsKeyArn == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "kmsKeyArn");
}
this.kmsKeyArn = kmsKeyArn;
return this;
}
@CustomType.Setter
public Builder multiAz(Boolean multiAz) {
if (multiAz == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "multiAz");
}
this.multiAz = multiAz;
return this;
}
@CustomType.Setter
public Builder networkType(String networkType) {
if (networkType == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "networkType");
}
this.networkType = networkType;
return this;
}
@CustomType.Setter
public Builder preferredMaintenanceWindow(String preferredMaintenanceWindow) {
if (preferredMaintenanceWindow == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "preferredMaintenanceWindow");
}
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
return this;
}
@CustomType.Setter
public Builder publiclyAccessible(Boolean publiclyAccessible) {
if (publiclyAccessible == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "publiclyAccessible");
}
this.publiclyAccessible = publiclyAccessible;
return this;
}
@CustomType.Setter
public Builder replicationInstanceArn(String replicationInstanceArn) {
if (replicationInstanceArn == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationInstanceArn");
}
this.replicationInstanceArn = replicationInstanceArn;
return this;
}
@CustomType.Setter
public Builder replicationInstanceClass(String replicationInstanceClass) {
if (replicationInstanceClass == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationInstanceClass");
}
this.replicationInstanceClass = replicationInstanceClass;
return this;
}
@CustomType.Setter
public Builder replicationInstanceId(String replicationInstanceId) {
if (replicationInstanceId == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationInstanceId");
}
this.replicationInstanceId = replicationInstanceId;
return this;
}
@CustomType.Setter
public Builder replicationInstancePrivateIps(List replicationInstancePrivateIps) {
if (replicationInstancePrivateIps == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationInstancePrivateIps");
}
this.replicationInstancePrivateIps = replicationInstancePrivateIps;
return this;
}
public Builder replicationInstancePrivateIps(String... replicationInstancePrivateIps) {
return replicationInstancePrivateIps(List.of(replicationInstancePrivateIps));
}
@CustomType.Setter
public Builder replicationInstancePublicIps(List replicationInstancePublicIps) {
if (replicationInstancePublicIps == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationInstancePublicIps");
}
this.replicationInstancePublicIps = replicationInstancePublicIps;
return this;
}
public Builder replicationInstancePublicIps(String... replicationInstancePublicIps) {
return replicationInstancePublicIps(List.of(replicationInstancePublicIps));
}
@CustomType.Setter
public Builder replicationSubnetGroupId(String replicationSubnetGroupId) {
if (replicationSubnetGroupId == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "replicationSubnetGroupId");
}
this.replicationSubnetGroupId = replicationSubnetGroupId;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder vpcSecurityGroupIds(List vpcSecurityGroupIds) {
if (vpcSecurityGroupIds == null) {
throw new MissingRequiredPropertyException("GetReplicationInstanceResult", "vpcSecurityGroupIds");
}
this.vpcSecurityGroupIds = vpcSecurityGroupIds;
return this;
}
public Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds) {
return vpcSecurityGroupIds(List.of(vpcSecurityGroupIds));
}
public GetReplicationInstanceResult build() {
final var _resultValue = new GetReplicationInstanceResult();
_resultValue.allocatedStorage = allocatedStorage;
_resultValue.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
_resultValue.availabilityZone = availabilityZone;
_resultValue.engineVersion = engineVersion;
_resultValue.id = id;
_resultValue.kmsKeyArn = kmsKeyArn;
_resultValue.multiAz = multiAz;
_resultValue.networkType = networkType;
_resultValue.preferredMaintenanceWindow = preferredMaintenanceWindow;
_resultValue.publiclyAccessible = publiclyAccessible;
_resultValue.replicationInstanceArn = replicationInstanceArn;
_resultValue.replicationInstanceClass = replicationInstanceClass;
_resultValue.replicationInstanceId = replicationInstanceId;
_resultValue.replicationInstancePrivateIps = replicationInstancePrivateIps;
_resultValue.replicationInstancePublicIps = replicationInstancePublicIps;
_resultValue.replicationSubnetGroupId = replicationSubnetGroupId;
_resultValue.tags = tags;
_resultValue.vpcSecurityGroupIds = vpcSecurityGroupIds;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy