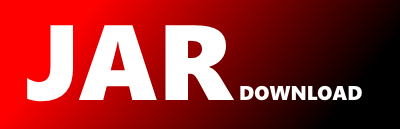
com.pulumi.aws.ec2.TrafficMirrorSessionArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class TrafficMirrorSessionArgs extends com.pulumi.resources.ResourceArgs {
public static final TrafficMirrorSessionArgs Empty = new TrafficMirrorSessionArgs();
/**
* A description of the traffic mirror session.
*
*/
@Import(name="description")
private @Nullable Output description;
/**
* @return A description of the traffic mirror session.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy