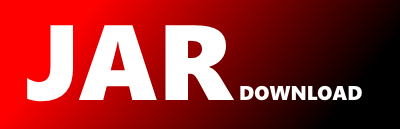
com.pulumi.aws.ec2.VpcIpv6CidrBlockAssociation Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.VpcIpv6CidrBlockAssociationArgs;
import com.pulumi.aws.ec2.inputs.VpcIpv6CidrBlockAssociationState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource to associate additional IPv6 CIDR blocks with a VPC.
*
* The `aws.ec2.VpcIpv6CidrBlockAssociation` resource allows IPv6 CIDR blocks to be added to the VPC.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.VpcIpv6CidrBlockAssociation;
* import com.pulumi.aws.ec2.VpcIpv6CidrBlockAssociationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new Vpc("test", VpcArgs.builder()
* .cidrBlock("10.0.0.0/16")
* .build());
*
* var testVpcIpv6CidrBlockAssociation = new VpcIpv6CidrBlockAssociation("testVpcIpv6CidrBlockAssociation", VpcIpv6CidrBlockAssociationArgs.builder()
* .ipv6IpamPoolId(testAwsVpcIpamPool.id())
* .vpcId(test.id())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import `aws_vpc_ipv6_cidr_block_association` using the VPC CIDR Association ID. For example:
*
* ```sh
* $ pulumi import aws:ec2/vpcIpv6CidrBlockAssociation:VpcIpv6CidrBlockAssociation example vpc-cidr-assoc-xxxxxxxx
* ```
*
*/
@ResourceType(type="aws:ec2/vpcIpv6CidrBlockAssociation:VpcIpv6CidrBlockAssociation")
public class VpcIpv6CidrBlockAssociation extends com.pulumi.resources.CustomResource {
/**
* Requests an Amazon-provided IPv6 CIDR block with a /56 prefix length for the VPC. You cannot specify the range of IPv6 addresses, or the size of the CIDR block. Default is `false`. Conflicts with `ipv6_pam_pool_id`, `ipv6_pool`, `ipv6_cidr_block` and `ipv6_netmask_length`.
*
*/
@Export(name="assignGeneratedIpv6CidrBlock", refs={Boolean.class}, tree="[0]")
private Output assignGeneratedIpv6CidrBlock;
/**
* @return Requests an Amazon-provided IPv6 CIDR block with a /56 prefix length for the VPC. You cannot specify the range of IPv6 addresses, or the size of the CIDR block. Default is `false`. Conflicts with `ipv6_pam_pool_id`, `ipv6_pool`, `ipv6_cidr_block` and `ipv6_netmask_length`.
*
*/
public Output assignGeneratedIpv6CidrBlock() {
return this.assignGeneratedIpv6CidrBlock;
}
/**
* The source that allocated the IP address space. Values: `amazon`, `byoip`, `none`.
*
*/
@Export(name="ipSource", refs={String.class}, tree="[0]")
private Output ipSource;
/**
* @return The source that allocated the IP address space. Values: `amazon`, `byoip`, `none`.
*
*/
public Output ipSource() {
return this.ipSource;
}
/**
* Public IPv6 addresses are those advertised on the internet from AWS. Private IP addresses are not and cannot be advertised on the internet from AWS. Values: `public`, `private`.
*
*/
@Export(name="ipv6AddressAttribute", refs={String.class}, tree="[0]")
private Output ipv6AddressAttribute;
/**
* @return Public IPv6 addresses are those advertised on the internet from AWS. Private IP addresses are not and cannot be advertised on the internet from AWS. Values: `public`, `private`.
*
*/
public Output ipv6AddressAttribute() {
return this.ipv6AddressAttribute;
}
/**
* The IPv6 CIDR block for the VPC. CIDR can be explicitly set or it can be derived from IPAM using `ipv6_netmask_length`. This parameter is required if `ipv6_netmask_length` is not set and the IPAM pool does not have `allocation_default_netmask` set. Conflicts with `assign_generated_ipv6_cidr_block`.
*
*/
@Export(name="ipv6CidrBlock", refs={String.class}, tree="[0]")
private Output ipv6CidrBlock;
/**
* @return The IPv6 CIDR block for the VPC. CIDR can be explicitly set or it can be derived from IPAM using `ipv6_netmask_length`. This parameter is required if `ipv6_netmask_length` is not set and the IPAM pool does not have `allocation_default_netmask` set. Conflicts with `assign_generated_ipv6_cidr_block`.
*
*/
public Output ipv6CidrBlock() {
return this.ipv6CidrBlock;
}
/**
* - (Optional) The ID of an IPv6 IPAM pool you want to use for allocating this VPC's CIDR. IPAM is a VPC feature that you can use to automate your IP address management workflows including assigning, tracking, troubleshooting, and auditing IP addresses across AWS Regions and accounts. Conflict with `assign_generated_ipv6_cidr_block` and `ipv6_ipam_pool_id`.
*
*/
@Export(name="ipv6IpamPoolId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> ipv6IpamPoolId;
/**
* @return - (Optional) The ID of an IPv6 IPAM pool you want to use for allocating this VPC's CIDR. IPAM is a VPC feature that you can use to automate your IP address management workflows including assigning, tracking, troubleshooting, and auditing IP addresses across AWS Regions and accounts. Conflict with `assign_generated_ipv6_cidr_block` and `ipv6_ipam_pool_id`.
*
*/
public Output> ipv6IpamPoolId() {
return Codegen.optional(this.ipv6IpamPoolId);
}
/**
* The netmask length of the IPv6 CIDR you want to allocate to this VPC. Requires specifying a `ipv6_ipam_pool_id`. This parameter is optional if the IPAM pool has `allocation_default_netmask` set, otherwise it or `ipv6_cidr_block` are required. Conflicts with `assign_generated_ipv6_cidr_block` and `ipv6_ipam_pool_id`.
*
*/
@Export(name="ipv6NetmaskLength", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> ipv6NetmaskLength;
/**
* @return The netmask length of the IPv6 CIDR you want to allocate to this VPC. Requires specifying a `ipv6_ipam_pool_id`. This parameter is optional if the IPAM pool has `allocation_default_netmask` set, otherwise it or `ipv6_cidr_block` are required. Conflicts with `assign_generated_ipv6_cidr_block` and `ipv6_ipam_pool_id`.
*
*/
public Output> ipv6NetmaskLength() {
return Codegen.optional(this.ipv6NetmaskLength);
}
/**
* The ID of an IPv6 address pool from which to allocate the IPv6 CIDR block. Conflicts with `ipv6_pam_pool_id`, `ipv6_pool`.
*
*/
@Export(name="ipv6Pool", refs={String.class}, tree="[0]")
private Output ipv6Pool;
/**
* @return The ID of an IPv6 address pool from which to allocate the IPv6 CIDR block. Conflicts with `ipv6_pam_pool_id`, `ipv6_pool`.
*
*/
public Output ipv6Pool() {
return this.ipv6Pool;
}
/**
* The ID of the VPC to make the association with.
*
*/
@Export(name="vpcId", refs={String.class}, tree="[0]")
private Output vpcId;
/**
* @return The ID of the VPC to make the association with.
*
*/
public Output vpcId() {
return this.vpcId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public VpcIpv6CidrBlockAssociation(java.lang.String name) {
this(name, VpcIpv6CidrBlockAssociationArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public VpcIpv6CidrBlockAssociation(java.lang.String name, VpcIpv6CidrBlockAssociationArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public VpcIpv6CidrBlockAssociation(java.lang.String name, VpcIpv6CidrBlockAssociationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2/vpcIpv6CidrBlockAssociation:VpcIpv6CidrBlockAssociation", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private VpcIpv6CidrBlockAssociation(java.lang.String name, Output id, @Nullable VpcIpv6CidrBlockAssociationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("aws:ec2/vpcIpv6CidrBlockAssociation:VpcIpv6CidrBlockAssociation", name, state, makeResourceOptions(options, id), false);
}
private static VpcIpv6CidrBlockAssociationArgs makeArgs(VpcIpv6CidrBlockAssociationArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? VpcIpv6CidrBlockAssociationArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static VpcIpv6CidrBlockAssociation get(java.lang.String name, Output id, @Nullable VpcIpv6CidrBlockAssociationState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new VpcIpv6CidrBlockAssociation(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy