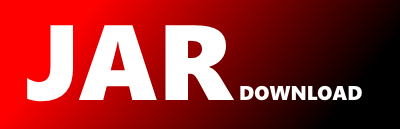
com.pulumi.aws.ec2.VpcPeeringConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.ec2.VpcPeeringConnectionArgs;
import com.pulumi.aws.ec2.inputs.VpcPeeringConnectionState;
import com.pulumi.aws.ec2.outputs.VpcPeeringConnectionAccepter;
import com.pulumi.aws.ec2.outputs.VpcPeeringConnectionRequester;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides a resource to manage a VPC peering connection.
*
* > **NOTE on VPC Peering Connections and VPC Peering Connection Options:** This provider provides
* both a standalone VPC Peering Connection Options and a VPC Peering Connection
* resource with `accepter` and `requester` attributes. Do not manage options for the same VPC peering
* connection in both a VPC Peering Connection resource and a VPC Peering Connection Options resource.
* Doing so will cause a conflict of options and will overwrite the options.
* Using a VPC Peering Connection Options resource decouples management of the connection options from
* management of the VPC Peering Connection and allows options to be set correctly in cross-account scenarios.
*
* > **Note:** For cross-account (requester's AWS account differs from the accepter's AWS account) or inter-region
* VPC Peering Connections use the `aws.ec2.VpcPeeringConnection` resource to manage the requester's side of the
* connection and use the `aws.ec2.VpcPeeringConnectionAccepter` resource to manage the accepter's side of the connection.
*
* > **Note:** Creating multiple `aws.ec2.VpcPeeringConnection` resources with the same `peer_vpc_id` and `vpc_id` will not produce an error. Instead, AWS will return the connection `id` that already exists, resulting in multiple `aws.ec2.VpcPeeringConnection` resources with the same `id`.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.VpcPeeringConnection;
* import com.pulumi.aws.ec2.VpcPeeringConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foo = new VpcPeeringConnection("foo", VpcPeeringConnectionArgs.builder()
* .peerOwnerId(peerOwnerId)
* .peerVpcId(bar.id())
* .vpcId(fooAwsVpc.id())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Basic usage with connection options:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.VpcPeeringConnection;
* import com.pulumi.aws.ec2.VpcPeeringConnectionArgs;
* import com.pulumi.aws.ec2.inputs.VpcPeeringConnectionAccepterArgs;
* import com.pulumi.aws.ec2.inputs.VpcPeeringConnectionRequesterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var foo = new VpcPeeringConnection("foo", VpcPeeringConnectionArgs.builder()
* .peerOwnerId(peerOwnerId)
* .peerVpcId(bar.id())
* .vpcId(fooAwsVpc.id())
* .accepter(VpcPeeringConnectionAccepterArgs.builder()
* .allowRemoteVpcDnsResolution(true)
* .build())
* .requester(VpcPeeringConnectionRequesterArgs.builder()
* .allowRemoteVpcDnsResolution(true)
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Basic usage with tags:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.VpcPeeringConnection;
* import com.pulumi.aws.ec2.VpcPeeringConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fooVpc = new Vpc("fooVpc", VpcArgs.builder()
* .cidrBlock("10.1.0.0/16")
* .build());
*
* var bar = new Vpc("bar", VpcArgs.builder()
* .cidrBlock("10.2.0.0/16")
* .build());
*
* var foo = new VpcPeeringConnection("foo", VpcPeeringConnectionArgs.builder()
* .peerOwnerId(peerOwnerId)
* .peerVpcId(bar.id())
* .vpcId(fooVpc.id())
* .autoAccept(true)
* .tags(Map.of("Name", "VPC Peering between foo and bar"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* Basic usage with region:
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.ec2.Vpc;
* import com.pulumi.aws.ec2.VpcArgs;
* import com.pulumi.aws.ec2.VpcPeeringConnection;
* import com.pulumi.aws.ec2.VpcPeeringConnectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fooVpc = new Vpc("fooVpc", VpcArgs.builder()
* .cidrBlock("10.1.0.0/16")
* .build());
*
* var bar = new Vpc("bar", VpcArgs.builder()
* .cidrBlock("10.2.0.0/16")
* .build());
*
* var foo = new VpcPeeringConnection("foo", VpcPeeringConnectionArgs.builder()
* .peerOwnerId(peerOwnerId)
* .peerVpcId(bar.id())
* .vpcId(fooVpc.id())
* .peerRegion("us-east-1")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Notes
*
* If both VPCs are not in the same AWS account and region do not enable the `auto_accept` attribute.
* The accepter can manage its side of the connection using the `aws.ec2.VpcPeeringConnectionAccepter` resource
* or accept the connection manually using the AWS Management Console, AWS CLI, through SDKs, etc.
*
* ## Import
*
* Using `pulumi import`, import VPC Peering resources using the VPC peering `id`. For example:
*
* ```sh
* $ pulumi import aws:ec2/vpcPeeringConnection:VpcPeeringConnection test_connection pcx-111aaa111
* ```
*
*/
@ResourceType(type="aws:ec2/vpcPeeringConnection:VpcPeeringConnection")
public class VpcPeeringConnection extends com.pulumi.resources.CustomResource {
/**
* The status of the VPC Peering Connection request.
*
*/
@Export(name="acceptStatus", refs={String.class}, tree="[0]")
private Output acceptStatus;
/**
* @return The status of the VPC Peering Connection request.
*
*/
public Output acceptStatus() {
return this.acceptStatus;
}
/**
* An optional configuration block that allows for [VPC Peering Connection](https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options to be set for the VPC that accepts
* the peering connection (a maximum of one).
*
*/
@Export(name="accepter", refs={VpcPeeringConnectionAccepter.class}, tree="[0]")
private Output accepter;
/**
* @return An optional configuration block that allows for [VPC Peering Connection](https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options to be set for the VPC that accepts
* the peering connection (a maximum of one).
*
*/
public Output accepter() {
return this.accepter;
}
/**
* Accept the peering (both VPCs need to be in the same AWS account and region).
*
*/
@Export(name="autoAccept", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> autoAccept;
/**
* @return Accept the peering (both VPCs need to be in the same AWS account and region).
*
*/
public Output> autoAccept() {
return Codegen.optional(this.autoAccept);
}
/**
* The AWS account ID of the target peer VPC.
* Defaults to the account ID the [AWS provider][1] is currently connected to, so must be managed if connecting cross-account.
*
*/
@Export(name="peerOwnerId", refs={String.class}, tree="[0]")
private Output peerOwnerId;
/**
* @return The AWS account ID of the target peer VPC.
* Defaults to the account ID the [AWS provider][1] is currently connected to, so must be managed if connecting cross-account.
*
*/
public Output peerOwnerId() {
return this.peerOwnerId;
}
/**
* The region of the accepter VPC of the VPC Peering Connection. `auto_accept` must be `false`,
* and use the `aws.ec2.VpcPeeringConnectionAccepter` to manage the accepter side.
*
*/
@Export(name="peerRegion", refs={String.class}, tree="[0]")
private Output peerRegion;
/**
* @return The region of the accepter VPC of the VPC Peering Connection. `auto_accept` must be `false`,
* and use the `aws.ec2.VpcPeeringConnectionAccepter` to manage the accepter side.
*
*/
public Output peerRegion() {
return this.peerRegion;
}
/**
* The ID of the target VPC with which you are creating the VPC Peering Connection.
*
*/
@Export(name="peerVpcId", refs={String.class}, tree="[0]")
private Output peerVpcId;
/**
* @return The ID of the target VPC with which you are creating the VPC Peering Connection.
*
*/
public Output peerVpcId() {
return this.peerVpcId;
}
/**
* A optional configuration block that allows for [VPC Peering Connection](https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options to be set for the VPC that requests
* the peering connection (a maximum of one).
*
*/
@Export(name="requester", refs={VpcPeeringConnectionRequester.class}, tree="[0]")
private Output requester;
/**
* @return A optional configuration block that allows for [VPC Peering Connection](https://docs.aws.amazon.com/vpc/latest/peering/what-is-vpc-peering.html) options to be set for the VPC that requests
* the peering connection (a maximum of one).
*
*/
public Output requester() {
return this.requester;
}
/**
* A map of tags to assign to the resource. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the resource. .If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy