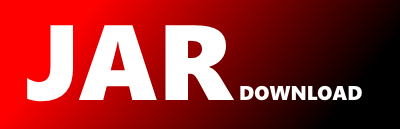
com.pulumi.aws.ec2.inputs.EipState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class EipState extends com.pulumi.resources.ResourceArgs {
public static final EipState Empty = new EipState();
/**
* IP address from an EC2 BYOIP pool. This option is only available for VPC EIPs.
*
*/
@Import(name="address")
private @Nullable Output address;
/**
* @return IP address from an EC2 BYOIP pool. This option is only available for VPC EIPs.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy