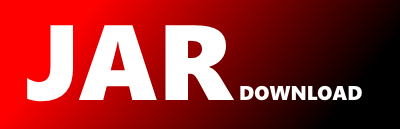
com.pulumi.aws.ec2.inputs.VpcIpamResourceDiscoveryState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.inputs;
import com.pulumi.aws.ec2.inputs.VpcIpamResourceDiscoveryOperatingRegionArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class VpcIpamResourceDiscoveryState extends com.pulumi.resources.ResourceArgs {
public static final VpcIpamResourceDiscoveryState Empty = new VpcIpamResourceDiscoveryState();
/**
* Amazon Resource Name (ARN) of IPAM Resource Discovery
*
*/
@Import(name="arn")
private @Nullable Output arn;
/**
* @return Amazon Resource Name (ARN) of IPAM Resource Discovery
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy