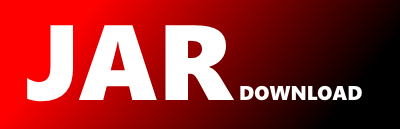
com.pulumi.aws.ec2.outputs.GetInstanceResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ec2.outputs;
import com.pulumi.aws.ec2.outputs.GetInstanceCreditSpecification;
import com.pulumi.aws.ec2.outputs.GetInstanceEbsBlockDevice;
import com.pulumi.aws.ec2.outputs.GetInstanceEnclaveOption;
import com.pulumi.aws.ec2.outputs.GetInstanceEphemeralBlockDevice;
import com.pulumi.aws.ec2.outputs.GetInstanceFilter;
import com.pulumi.aws.ec2.outputs.GetInstanceMaintenanceOption;
import com.pulumi.aws.ec2.outputs.GetInstanceMetadataOption;
import com.pulumi.aws.ec2.outputs.GetInstancePrivateDnsNameOption;
import com.pulumi.aws.ec2.outputs.GetInstanceRootBlockDevice;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetInstanceResult {
/**
* @return ID of the AMI used to launch the instance.
*
*/
private String ami;
/**
* @return ARN of the instance.
*
*/
private String arn;
/**
* @return Whether or not the Instance is associated with a public IP address or not (Boolean).
*
*/
private Boolean associatePublicIpAddress;
/**
* @return Availability zone of the Instance.
*
*/
private String availabilityZone;
/**
* @return Credit specification of the Instance.
*
*/
private List creditSpecifications;
/**
* @return Whether or not EC2 Instance Stop Protection](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/Stop_Start.html#Using_StopProtection) is enabled (Boolean).
*
*/
private Boolean disableApiStop;
/**
* @return Whether or not [EC2 Instance Termination Protection](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/terminating-instances.html#Using_ChangingDisableAPITermination) is enabled (Boolean).
*
*/
private Boolean disableApiTermination;
/**
* @return EBS block device mappings of the Instance.
*
*/
private List ebsBlockDevices;
/**
* @return Whether the Instance is EBS optimized or not (Boolean).
*
*/
private Boolean ebsOptimized;
/**
* @return Enclave options of the instance.
*
*/
private List enclaveOptions;
/**
* @return Ephemeral block device mappings of the Instance.
*
*/
private List ephemeralBlockDevices;
private @Nullable List filters;
private @Nullable Boolean getPasswordData;
private @Nullable Boolean getUserData;
/**
* @return ID of the dedicated host the instance will be assigned to.
*
*/
private String hostId;
/**
* @return ARN of the host resource group the instance is associated with.
*
*/
private String hostResourceGroupArn;
/**
* @return Name of the instance profile associated with the Instance.
*
*/
private String iamInstanceProfile;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable String instanceId;
/**
* @return State of the instance. One of: `pending`, `running`, `shutting-down`, `terminated`, `stopping`, `stopped`. See [Instance Lifecycle](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/ec2-instance-lifecycle.html) for more information.
*
*/
private String instanceState;
private Map instanceTags;
/**
* @return Type of the Instance.
*
*/
private String instanceType;
/**
* @return IPv6 addresses associated to the Instance, if applicable. **NOTE**: Unlike the IPv4 address, this doesn't change if you attach an EIP to the instance.
*
*/
private List ipv6Addresses;
/**
* @return Key name of the Instance.
*
*/
private String keyName;
/**
* @return Time the instance was launched.
*
*/
private String launchTime;
/**
* @return Maintenance and recovery options for the instance.
*
*/
private List maintenanceOptions;
/**
* @return Metadata options of the Instance.
*
*/
private List metadataOptions;
/**
* @return Whether detailed monitoring is enabled or disabled for the Instance (Boolean).
*
*/
private Boolean monitoring;
/**
* @return ID of the network interface that was created with the Instance.
*
*/
private String networkInterfaceId;
/**
* @return ARN of the Outpost.
*
*/
private String outpostArn;
/**
* @return Base-64 encoded encrypted password data for the instance. Useful for getting the administrator password for instances running Microsoft Windows. This attribute is only exported if `get_password_data` is true. See [GetPasswordData](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_GetPasswordData.html) for more information.
*
*/
private String passwordData;
/**
* @return Placement group of the Instance.
*
*/
private String placementGroup;
/**
* @return Number of the partition the instance is in.
*
*/
private Integer placementPartitionNumber;
/**
* @return Private DNS name assigned to the Instance. Can only be used inside the Amazon EC2, and only available if you've enabled DNS hostnames for your VPC.
*
*/
private String privateDns;
/**
* @return Options for the instance hostname.
*
*/
private List privateDnsNameOptions;
/**
* @return Private IP address assigned to the Instance.
*
*/
private String privateIp;
/**
* @return Public DNS name assigned to the Instance. For EC2-VPC, this is only available if you've enabled DNS hostnames for your VPC.
*
*/
private String publicDns;
/**
* @return Public IP address assigned to the Instance, if applicable. **NOTE**: If you are using an `aws.ec2.Eip` with your instance, you should refer to the EIP's address directly and not use `public_ip`, as this field will change after the EIP is attached.
*
*/
private String publicIp;
/**
* @return Root block device mappings of the Instance
*
*/
private List rootBlockDevices;
/**
* @return Secondary private IPv4 addresses assigned to the instance's primary network interface (eth0) in a VPC.
*
*/
private List secondaryPrivateIps;
/**
* @return Associated security groups.
*
*/
private List securityGroups;
/**
* @return Whether the network interface performs source/destination checking (Boolean).
*
*/
private Boolean sourceDestCheck;
/**
* @return VPC subnet ID.
*
*/
private String subnetId;
/**
* @return Map of tags assigned to the Instance.
*
*/
private Map tags;
/**
* @return Tenancy of the instance: `dedicated`, `default`, `host`.
*
*/
private String tenancy;
/**
* @return SHA-1 hash of User Data supplied to the Instance.
*
*/
private String userData;
/**
* @return Base64 encoded contents of User Data supplied to the Instance. This attribute is only exported if `get_user_data` is true.
*
*/
private String userDataBase64;
/**
* @return Associated security groups in a non-default VPC.
*
*/
private List vpcSecurityGroupIds;
private GetInstanceResult() {}
/**
* @return ID of the AMI used to launch the instance.
*
*/
public String ami() {
return this.ami;
}
/**
* @return ARN of the instance.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Whether or not the Instance is associated with a public IP address or not (Boolean).
*
*/
public Boolean associatePublicIpAddress() {
return this.associatePublicIpAddress;
}
/**
* @return Availability zone of the Instance.
*
*/
public String availabilityZone() {
return this.availabilityZone;
}
/**
* @return Credit specification of the Instance.
*
*/
public List creditSpecifications() {
return this.creditSpecifications;
}
/**
* @return Whether or not EC2 Instance Stop Protection](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/Stop_Start.html#Using_StopProtection) is enabled (Boolean).
*
*/
public Boolean disableApiStop() {
return this.disableApiStop;
}
/**
* @return Whether or not [EC2 Instance Termination Protection](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/terminating-instances.html#Using_ChangingDisableAPITermination) is enabled (Boolean).
*
*/
public Boolean disableApiTermination() {
return this.disableApiTermination;
}
/**
* @return EBS block device mappings of the Instance.
*
*/
public List ebsBlockDevices() {
return this.ebsBlockDevices;
}
/**
* @return Whether the Instance is EBS optimized or not (Boolean).
*
*/
public Boolean ebsOptimized() {
return this.ebsOptimized;
}
/**
* @return Enclave options of the instance.
*
*/
public List enclaveOptions() {
return this.enclaveOptions;
}
/**
* @return Ephemeral block device mappings of the Instance.
*
*/
public List ephemeralBlockDevices() {
return this.ephemeralBlockDevices;
}
public List filters() {
return this.filters == null ? List.of() : this.filters;
}
public Optional getPasswordData() {
return Optional.ofNullable(this.getPasswordData);
}
public Optional getUserData() {
return Optional.ofNullable(this.getUserData);
}
/**
* @return ID of the dedicated host the instance will be assigned to.
*
*/
public String hostId() {
return this.hostId;
}
/**
* @return ARN of the host resource group the instance is associated with.
*
*/
public String hostResourceGroupArn() {
return this.hostResourceGroupArn;
}
/**
* @return Name of the instance profile associated with the Instance.
*
*/
public String iamInstanceProfile() {
return this.iamInstanceProfile;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional instanceId() {
return Optional.ofNullable(this.instanceId);
}
/**
* @return State of the instance. One of: `pending`, `running`, `shutting-down`, `terminated`, `stopping`, `stopped`. See [Instance Lifecycle](https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/ec2-instance-lifecycle.html) for more information.
*
*/
public String instanceState() {
return this.instanceState;
}
public Map instanceTags() {
return this.instanceTags;
}
/**
* @return Type of the Instance.
*
*/
public String instanceType() {
return this.instanceType;
}
/**
* @return IPv6 addresses associated to the Instance, if applicable. **NOTE**: Unlike the IPv4 address, this doesn't change if you attach an EIP to the instance.
*
*/
public List ipv6Addresses() {
return this.ipv6Addresses;
}
/**
* @return Key name of the Instance.
*
*/
public String keyName() {
return this.keyName;
}
/**
* @return Time the instance was launched.
*
*/
public String launchTime() {
return this.launchTime;
}
/**
* @return Maintenance and recovery options for the instance.
*
*/
public List maintenanceOptions() {
return this.maintenanceOptions;
}
/**
* @return Metadata options of the Instance.
*
*/
public List metadataOptions() {
return this.metadataOptions;
}
/**
* @return Whether detailed monitoring is enabled or disabled for the Instance (Boolean).
*
*/
public Boolean monitoring() {
return this.monitoring;
}
/**
* @return ID of the network interface that was created with the Instance.
*
*/
public String networkInterfaceId() {
return this.networkInterfaceId;
}
/**
* @return ARN of the Outpost.
*
*/
public String outpostArn() {
return this.outpostArn;
}
/**
* @return Base-64 encoded encrypted password data for the instance. Useful for getting the administrator password for instances running Microsoft Windows. This attribute is only exported if `get_password_data` is true. See [GetPasswordData](https://docs.aws.amazon.com/AWSEC2/latest/APIReference/API_GetPasswordData.html) for more information.
*
*/
public String passwordData() {
return this.passwordData;
}
/**
* @return Placement group of the Instance.
*
*/
public String placementGroup() {
return this.placementGroup;
}
/**
* @return Number of the partition the instance is in.
*
*/
public Integer placementPartitionNumber() {
return this.placementPartitionNumber;
}
/**
* @return Private DNS name assigned to the Instance. Can only be used inside the Amazon EC2, and only available if you've enabled DNS hostnames for your VPC.
*
*/
public String privateDns() {
return this.privateDns;
}
/**
* @return Options for the instance hostname.
*
*/
public List privateDnsNameOptions() {
return this.privateDnsNameOptions;
}
/**
* @return Private IP address assigned to the Instance.
*
*/
public String privateIp() {
return this.privateIp;
}
/**
* @return Public DNS name assigned to the Instance. For EC2-VPC, this is only available if you've enabled DNS hostnames for your VPC.
*
*/
public String publicDns() {
return this.publicDns;
}
/**
* @return Public IP address assigned to the Instance, if applicable. **NOTE**: If you are using an `aws.ec2.Eip` with your instance, you should refer to the EIP's address directly and not use `public_ip`, as this field will change after the EIP is attached.
*
*/
public String publicIp() {
return this.publicIp;
}
/**
* @return Root block device mappings of the Instance
*
*/
public List rootBlockDevices() {
return this.rootBlockDevices;
}
/**
* @return Secondary private IPv4 addresses assigned to the instance's primary network interface (eth0) in a VPC.
*
*/
public List secondaryPrivateIps() {
return this.secondaryPrivateIps;
}
/**
* @return Associated security groups.
*
*/
public List securityGroups() {
return this.securityGroups;
}
/**
* @return Whether the network interface performs source/destination checking (Boolean).
*
*/
public Boolean sourceDestCheck() {
return this.sourceDestCheck;
}
/**
* @return VPC subnet ID.
*
*/
public String subnetId() {
return this.subnetId;
}
/**
* @return Map of tags assigned to the Instance.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return Tenancy of the instance: `dedicated`, `default`, `host`.
*
*/
public String tenancy() {
return this.tenancy;
}
/**
* @return SHA-1 hash of User Data supplied to the Instance.
*
*/
public String userData() {
return this.userData;
}
/**
* @return Base64 encoded contents of User Data supplied to the Instance. This attribute is only exported if `get_user_data` is true.
*
*/
public String userDataBase64() {
return this.userDataBase64;
}
/**
* @return Associated security groups in a non-default VPC.
*
*/
public List vpcSecurityGroupIds() {
return this.vpcSecurityGroupIds;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetInstanceResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String ami;
private String arn;
private Boolean associatePublicIpAddress;
private String availabilityZone;
private List creditSpecifications;
private Boolean disableApiStop;
private Boolean disableApiTermination;
private List ebsBlockDevices;
private Boolean ebsOptimized;
private List enclaveOptions;
private List ephemeralBlockDevices;
private @Nullable List filters;
private @Nullable Boolean getPasswordData;
private @Nullable Boolean getUserData;
private String hostId;
private String hostResourceGroupArn;
private String iamInstanceProfile;
private String id;
private @Nullable String instanceId;
private String instanceState;
private Map instanceTags;
private String instanceType;
private List ipv6Addresses;
private String keyName;
private String launchTime;
private List maintenanceOptions;
private List metadataOptions;
private Boolean monitoring;
private String networkInterfaceId;
private String outpostArn;
private String passwordData;
private String placementGroup;
private Integer placementPartitionNumber;
private String privateDns;
private List privateDnsNameOptions;
private String privateIp;
private String publicDns;
private String publicIp;
private List rootBlockDevices;
private List secondaryPrivateIps;
private List securityGroups;
private Boolean sourceDestCheck;
private String subnetId;
private Map tags;
private String tenancy;
private String userData;
private String userDataBase64;
private List vpcSecurityGroupIds;
public Builder() {}
public Builder(GetInstanceResult defaults) {
Objects.requireNonNull(defaults);
this.ami = defaults.ami;
this.arn = defaults.arn;
this.associatePublicIpAddress = defaults.associatePublicIpAddress;
this.availabilityZone = defaults.availabilityZone;
this.creditSpecifications = defaults.creditSpecifications;
this.disableApiStop = defaults.disableApiStop;
this.disableApiTermination = defaults.disableApiTermination;
this.ebsBlockDevices = defaults.ebsBlockDevices;
this.ebsOptimized = defaults.ebsOptimized;
this.enclaveOptions = defaults.enclaveOptions;
this.ephemeralBlockDevices = defaults.ephemeralBlockDevices;
this.filters = defaults.filters;
this.getPasswordData = defaults.getPasswordData;
this.getUserData = defaults.getUserData;
this.hostId = defaults.hostId;
this.hostResourceGroupArn = defaults.hostResourceGroupArn;
this.iamInstanceProfile = defaults.iamInstanceProfile;
this.id = defaults.id;
this.instanceId = defaults.instanceId;
this.instanceState = defaults.instanceState;
this.instanceTags = defaults.instanceTags;
this.instanceType = defaults.instanceType;
this.ipv6Addresses = defaults.ipv6Addresses;
this.keyName = defaults.keyName;
this.launchTime = defaults.launchTime;
this.maintenanceOptions = defaults.maintenanceOptions;
this.metadataOptions = defaults.metadataOptions;
this.monitoring = defaults.monitoring;
this.networkInterfaceId = defaults.networkInterfaceId;
this.outpostArn = defaults.outpostArn;
this.passwordData = defaults.passwordData;
this.placementGroup = defaults.placementGroup;
this.placementPartitionNumber = defaults.placementPartitionNumber;
this.privateDns = defaults.privateDns;
this.privateDnsNameOptions = defaults.privateDnsNameOptions;
this.privateIp = defaults.privateIp;
this.publicDns = defaults.publicDns;
this.publicIp = defaults.publicIp;
this.rootBlockDevices = defaults.rootBlockDevices;
this.secondaryPrivateIps = defaults.secondaryPrivateIps;
this.securityGroups = defaults.securityGroups;
this.sourceDestCheck = defaults.sourceDestCheck;
this.subnetId = defaults.subnetId;
this.tags = defaults.tags;
this.tenancy = defaults.tenancy;
this.userData = defaults.userData;
this.userDataBase64 = defaults.userDataBase64;
this.vpcSecurityGroupIds = defaults.vpcSecurityGroupIds;
}
@CustomType.Setter
public Builder ami(String ami) {
if (ami == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "ami");
}
this.ami = ami;
return this;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder associatePublicIpAddress(Boolean associatePublicIpAddress) {
if (associatePublicIpAddress == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "associatePublicIpAddress");
}
this.associatePublicIpAddress = associatePublicIpAddress;
return this;
}
@CustomType.Setter
public Builder availabilityZone(String availabilityZone) {
if (availabilityZone == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "availabilityZone");
}
this.availabilityZone = availabilityZone;
return this;
}
@CustomType.Setter
public Builder creditSpecifications(List creditSpecifications) {
if (creditSpecifications == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "creditSpecifications");
}
this.creditSpecifications = creditSpecifications;
return this;
}
public Builder creditSpecifications(GetInstanceCreditSpecification... creditSpecifications) {
return creditSpecifications(List.of(creditSpecifications));
}
@CustomType.Setter
public Builder disableApiStop(Boolean disableApiStop) {
if (disableApiStop == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "disableApiStop");
}
this.disableApiStop = disableApiStop;
return this;
}
@CustomType.Setter
public Builder disableApiTermination(Boolean disableApiTermination) {
if (disableApiTermination == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "disableApiTermination");
}
this.disableApiTermination = disableApiTermination;
return this;
}
@CustomType.Setter
public Builder ebsBlockDevices(List ebsBlockDevices) {
if (ebsBlockDevices == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "ebsBlockDevices");
}
this.ebsBlockDevices = ebsBlockDevices;
return this;
}
public Builder ebsBlockDevices(GetInstanceEbsBlockDevice... ebsBlockDevices) {
return ebsBlockDevices(List.of(ebsBlockDevices));
}
@CustomType.Setter
public Builder ebsOptimized(Boolean ebsOptimized) {
if (ebsOptimized == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "ebsOptimized");
}
this.ebsOptimized = ebsOptimized;
return this;
}
@CustomType.Setter
public Builder enclaveOptions(List enclaveOptions) {
if (enclaveOptions == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "enclaveOptions");
}
this.enclaveOptions = enclaveOptions;
return this;
}
public Builder enclaveOptions(GetInstanceEnclaveOption... enclaveOptions) {
return enclaveOptions(List.of(enclaveOptions));
}
@CustomType.Setter
public Builder ephemeralBlockDevices(List ephemeralBlockDevices) {
if (ephemeralBlockDevices == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "ephemeralBlockDevices");
}
this.ephemeralBlockDevices = ephemeralBlockDevices;
return this;
}
public Builder ephemeralBlockDevices(GetInstanceEphemeralBlockDevice... ephemeralBlockDevices) {
return ephemeralBlockDevices(List.of(ephemeralBlockDevices));
}
@CustomType.Setter
public Builder filters(@Nullable List filters) {
this.filters = filters;
return this;
}
public Builder filters(GetInstanceFilter... filters) {
return filters(List.of(filters));
}
@CustomType.Setter
public Builder getPasswordData(@Nullable Boolean getPasswordData) {
this.getPasswordData = getPasswordData;
return this;
}
@CustomType.Setter
public Builder getUserData(@Nullable Boolean getUserData) {
this.getUserData = getUserData;
return this;
}
@CustomType.Setter
public Builder hostId(String hostId) {
if (hostId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "hostId");
}
this.hostId = hostId;
return this;
}
@CustomType.Setter
public Builder hostResourceGroupArn(String hostResourceGroupArn) {
if (hostResourceGroupArn == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "hostResourceGroupArn");
}
this.hostResourceGroupArn = hostResourceGroupArn;
return this;
}
@CustomType.Setter
public Builder iamInstanceProfile(String iamInstanceProfile) {
if (iamInstanceProfile == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "iamInstanceProfile");
}
this.iamInstanceProfile = iamInstanceProfile;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder instanceId(@Nullable String instanceId) {
this.instanceId = instanceId;
return this;
}
@CustomType.Setter
public Builder instanceState(String instanceState) {
if (instanceState == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "instanceState");
}
this.instanceState = instanceState;
return this;
}
@CustomType.Setter
public Builder instanceTags(Map instanceTags) {
if (instanceTags == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "instanceTags");
}
this.instanceTags = instanceTags;
return this;
}
@CustomType.Setter
public Builder instanceType(String instanceType) {
if (instanceType == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "instanceType");
}
this.instanceType = instanceType;
return this;
}
@CustomType.Setter
public Builder ipv6Addresses(List ipv6Addresses) {
if (ipv6Addresses == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "ipv6Addresses");
}
this.ipv6Addresses = ipv6Addresses;
return this;
}
public Builder ipv6Addresses(String... ipv6Addresses) {
return ipv6Addresses(List.of(ipv6Addresses));
}
@CustomType.Setter
public Builder keyName(String keyName) {
if (keyName == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "keyName");
}
this.keyName = keyName;
return this;
}
@CustomType.Setter
public Builder launchTime(String launchTime) {
if (launchTime == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "launchTime");
}
this.launchTime = launchTime;
return this;
}
@CustomType.Setter
public Builder maintenanceOptions(List maintenanceOptions) {
if (maintenanceOptions == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "maintenanceOptions");
}
this.maintenanceOptions = maintenanceOptions;
return this;
}
public Builder maintenanceOptions(GetInstanceMaintenanceOption... maintenanceOptions) {
return maintenanceOptions(List.of(maintenanceOptions));
}
@CustomType.Setter
public Builder metadataOptions(List metadataOptions) {
if (metadataOptions == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "metadataOptions");
}
this.metadataOptions = metadataOptions;
return this;
}
public Builder metadataOptions(GetInstanceMetadataOption... metadataOptions) {
return metadataOptions(List.of(metadataOptions));
}
@CustomType.Setter
public Builder monitoring(Boolean monitoring) {
if (monitoring == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "monitoring");
}
this.monitoring = monitoring;
return this;
}
@CustomType.Setter
public Builder networkInterfaceId(String networkInterfaceId) {
if (networkInterfaceId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "networkInterfaceId");
}
this.networkInterfaceId = networkInterfaceId;
return this;
}
@CustomType.Setter
public Builder outpostArn(String outpostArn) {
if (outpostArn == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "outpostArn");
}
this.outpostArn = outpostArn;
return this;
}
@CustomType.Setter
public Builder passwordData(String passwordData) {
if (passwordData == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "passwordData");
}
this.passwordData = passwordData;
return this;
}
@CustomType.Setter
public Builder placementGroup(String placementGroup) {
if (placementGroup == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "placementGroup");
}
this.placementGroup = placementGroup;
return this;
}
@CustomType.Setter
public Builder placementPartitionNumber(Integer placementPartitionNumber) {
if (placementPartitionNumber == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "placementPartitionNumber");
}
this.placementPartitionNumber = placementPartitionNumber;
return this;
}
@CustomType.Setter
public Builder privateDns(String privateDns) {
if (privateDns == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "privateDns");
}
this.privateDns = privateDns;
return this;
}
@CustomType.Setter
public Builder privateDnsNameOptions(List privateDnsNameOptions) {
if (privateDnsNameOptions == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "privateDnsNameOptions");
}
this.privateDnsNameOptions = privateDnsNameOptions;
return this;
}
public Builder privateDnsNameOptions(GetInstancePrivateDnsNameOption... privateDnsNameOptions) {
return privateDnsNameOptions(List.of(privateDnsNameOptions));
}
@CustomType.Setter
public Builder privateIp(String privateIp) {
if (privateIp == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "privateIp");
}
this.privateIp = privateIp;
return this;
}
@CustomType.Setter
public Builder publicDns(String publicDns) {
if (publicDns == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "publicDns");
}
this.publicDns = publicDns;
return this;
}
@CustomType.Setter
public Builder publicIp(String publicIp) {
if (publicIp == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "publicIp");
}
this.publicIp = publicIp;
return this;
}
@CustomType.Setter
public Builder rootBlockDevices(List rootBlockDevices) {
if (rootBlockDevices == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "rootBlockDevices");
}
this.rootBlockDevices = rootBlockDevices;
return this;
}
public Builder rootBlockDevices(GetInstanceRootBlockDevice... rootBlockDevices) {
return rootBlockDevices(List.of(rootBlockDevices));
}
@CustomType.Setter
public Builder secondaryPrivateIps(List secondaryPrivateIps) {
if (secondaryPrivateIps == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "secondaryPrivateIps");
}
this.secondaryPrivateIps = secondaryPrivateIps;
return this;
}
public Builder secondaryPrivateIps(String... secondaryPrivateIps) {
return secondaryPrivateIps(List.of(secondaryPrivateIps));
}
@CustomType.Setter
public Builder securityGroups(List securityGroups) {
if (securityGroups == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "securityGroups");
}
this.securityGroups = securityGroups;
return this;
}
public Builder securityGroups(String... securityGroups) {
return securityGroups(List.of(securityGroups));
}
@CustomType.Setter
public Builder sourceDestCheck(Boolean sourceDestCheck) {
if (sourceDestCheck == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "sourceDestCheck");
}
this.sourceDestCheck = sourceDestCheck;
return this;
}
@CustomType.Setter
public Builder subnetId(String subnetId) {
if (subnetId == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "subnetId");
}
this.subnetId = subnetId;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tenancy(String tenancy) {
if (tenancy == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "tenancy");
}
this.tenancy = tenancy;
return this;
}
@CustomType.Setter
public Builder userData(String userData) {
if (userData == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "userData");
}
this.userData = userData;
return this;
}
@CustomType.Setter
public Builder userDataBase64(String userDataBase64) {
if (userDataBase64 == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "userDataBase64");
}
this.userDataBase64 = userDataBase64;
return this;
}
@CustomType.Setter
public Builder vpcSecurityGroupIds(List vpcSecurityGroupIds) {
if (vpcSecurityGroupIds == null) {
throw new MissingRequiredPropertyException("GetInstanceResult", "vpcSecurityGroupIds");
}
this.vpcSecurityGroupIds = vpcSecurityGroupIds;
return this;
}
public Builder vpcSecurityGroupIds(String... vpcSecurityGroupIds) {
return vpcSecurityGroupIds(List.of(vpcSecurityGroupIds));
}
public GetInstanceResult build() {
final var _resultValue = new GetInstanceResult();
_resultValue.ami = ami;
_resultValue.arn = arn;
_resultValue.associatePublicIpAddress = associatePublicIpAddress;
_resultValue.availabilityZone = availabilityZone;
_resultValue.creditSpecifications = creditSpecifications;
_resultValue.disableApiStop = disableApiStop;
_resultValue.disableApiTermination = disableApiTermination;
_resultValue.ebsBlockDevices = ebsBlockDevices;
_resultValue.ebsOptimized = ebsOptimized;
_resultValue.enclaveOptions = enclaveOptions;
_resultValue.ephemeralBlockDevices = ephemeralBlockDevices;
_resultValue.filters = filters;
_resultValue.getPasswordData = getPasswordData;
_resultValue.getUserData = getUserData;
_resultValue.hostId = hostId;
_resultValue.hostResourceGroupArn = hostResourceGroupArn;
_resultValue.iamInstanceProfile = iamInstanceProfile;
_resultValue.id = id;
_resultValue.instanceId = instanceId;
_resultValue.instanceState = instanceState;
_resultValue.instanceTags = instanceTags;
_resultValue.instanceType = instanceType;
_resultValue.ipv6Addresses = ipv6Addresses;
_resultValue.keyName = keyName;
_resultValue.launchTime = launchTime;
_resultValue.maintenanceOptions = maintenanceOptions;
_resultValue.metadataOptions = metadataOptions;
_resultValue.monitoring = monitoring;
_resultValue.networkInterfaceId = networkInterfaceId;
_resultValue.outpostArn = outpostArn;
_resultValue.passwordData = passwordData;
_resultValue.placementGroup = placementGroup;
_resultValue.placementPartitionNumber = placementPartitionNumber;
_resultValue.privateDns = privateDns;
_resultValue.privateDnsNameOptions = privateDnsNameOptions;
_resultValue.privateIp = privateIp;
_resultValue.publicDns = publicDns;
_resultValue.publicIp = publicIp;
_resultValue.rootBlockDevices = rootBlockDevices;
_resultValue.secondaryPrivateIps = secondaryPrivateIps;
_resultValue.securityGroups = securityGroups;
_resultValue.sourceDestCheck = sourceDestCheck;
_resultValue.subnetId = subnetId;
_resultValue.tags = tags;
_resultValue.tenancy = tenancy;
_resultValue.userData = userData;
_resultValue.userDataBase64 = userDataBase64;
_resultValue.vpcSecurityGroupIds = vpcSecurityGroupIds;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy