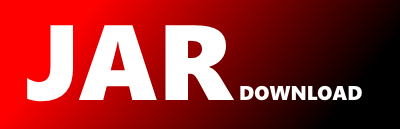
com.pulumi.aws.ecs.inputs.GetTaskExecutionPlainArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.ecs.inputs;
import com.pulumi.aws.ecs.inputs.GetTaskExecutionCapacityProviderStrategy;
import com.pulumi.aws.ecs.inputs.GetTaskExecutionNetworkConfiguration;
import com.pulumi.aws.ecs.inputs.GetTaskExecutionOverrides;
import com.pulumi.aws.ecs.inputs.GetTaskExecutionPlacementConstraint;
import com.pulumi.aws.ecs.inputs.GetTaskExecutionPlacementStrategy;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class GetTaskExecutionPlainArgs extends com.pulumi.resources.InvokeArgs {
public static final GetTaskExecutionPlainArgs Empty = new GetTaskExecutionPlainArgs();
/**
* Set of capacity provider strategies to use for the cluster. See below.
*
*/
@Import(name="capacityProviderStrategies")
private @Nullable List capacityProviderStrategies;
/**
* @return Set of capacity provider strategies to use for the cluster. See below.
*
*/
public Optional> capacityProviderStrategies() {
return Optional.ofNullable(this.capacityProviderStrategies);
}
/**
* An identifier that you provide to ensure the idempotency of the request. It must be unique and is case sensitive. Up to 64 characters are allowed. The valid characters are characters in the range of 33-126, inclusive. For more information, see [Ensuring idempotency](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/ECS_Idempotency.html).
*
*/
@Import(name="clientToken")
private @Nullable String clientToken;
/**
* @return An identifier that you provide to ensure the idempotency of the request. It must be unique and is case sensitive. Up to 64 characters are allowed. The valid characters are characters in the range of 33-126, inclusive. For more information, see [Ensuring idempotency](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/ECS_Idempotency.html).
*
*/
public Optional clientToken() {
return Optional.ofNullable(this.clientToken);
}
/**
* Short name or full Amazon Resource Name (ARN) of the cluster to run the task on.
*
*/
@Import(name="cluster", required=true)
private String cluster;
/**
* @return Short name or full Amazon Resource Name (ARN) of the cluster to run the task on.
*
*/
public String cluster() {
return this.cluster;
}
/**
* Number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks for each call.
*
*/
@Import(name="desiredCount")
private @Nullable Integer desiredCount;
/**
* @return Number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks for each call.
*
*/
public Optional desiredCount() {
return Optional.ofNullable(this.desiredCount);
}
/**
* Specifies whether to enable Amazon ECS managed tags for the tasks within the service.
*
*/
@Import(name="enableEcsManagedTags")
private @Nullable Boolean enableEcsManagedTags;
/**
* @return Specifies whether to enable Amazon ECS managed tags for the tasks within the service.
*
*/
public Optional enableEcsManagedTags() {
return Optional.ofNullable(this.enableEcsManagedTags);
}
/**
* Specifies whether to enable Amazon ECS Exec for the tasks within the service.
*
*/
@Import(name="enableExecuteCommand")
private @Nullable Boolean enableExecuteCommand;
/**
* @return Specifies whether to enable Amazon ECS Exec for the tasks within the service.
*
*/
public Optional enableExecuteCommand() {
return Optional.ofNullable(this.enableExecuteCommand);
}
/**
* Name of the task group to associate with the task. The default value is the family name of the task definition.
*
*/
@Import(name="group")
private @Nullable String group;
/**
* @return Name of the task group to associate with the task. The default value is the family name of the task definition.
*
*/
public Optional group() {
return Optional.ofNullable(this.group);
}
/**
* Launch type on which to run your service. Valid values are `EC2`, `FARGATE`, and `EXTERNAL`.
*
*/
@Import(name="launchType")
private @Nullable String launchType;
/**
* @return Launch type on which to run your service. Valid values are `EC2`, `FARGATE`, and `EXTERNAL`.
*
*/
public Optional launchType() {
return Optional.ofNullable(this.launchType);
}
/**
* Network configuration for the service. This parameter is required for task definitions that use the `awsvpc` network mode to receive their own Elastic Network Interface, and it is not supported for other network modes. See below.
*
*/
@Import(name="networkConfiguration")
private @Nullable GetTaskExecutionNetworkConfiguration networkConfiguration;
/**
* @return Network configuration for the service. This parameter is required for task definitions that use the `awsvpc` network mode to receive their own Elastic Network Interface, and it is not supported for other network modes. See below.
*
*/
public Optional networkConfiguration() {
return Optional.ofNullable(this.networkConfiguration);
}
/**
* A list of container overrides that specify the name of a container in the specified task definition and the overrides it should receive.
*
*/
@Import(name="overrides")
private @Nullable GetTaskExecutionOverrides overrides;
/**
* @return A list of container overrides that specify the name of a container in the specified task definition and the overrides it should receive.
*
*/
public Optional overrides() {
return Optional.ofNullable(this.overrides);
}
/**
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints for each task. See below.
*
*/
@Import(name="placementConstraints")
private @Nullable List placementConstraints;
/**
* @return An array of placement constraint objects to use for the task. You can specify up to 10 constraints for each task. See below.
*
*/
public Optional> placementConstraints() {
return Optional.ofNullable(this.placementConstraints);
}
/**
* The placement strategy objects to use for the task. You can specify a maximum of 5 strategy rules for each task. See below.
*
*/
@Import(name="placementStrategies")
private @Nullable List placementStrategies;
/**
* @return The placement strategy objects to use for the task. You can specify a maximum of 5 strategy rules for each task. See below.
*
*/
public Optional> placementStrategies() {
return Optional.ofNullable(this.placementStrategies);
}
/**
* The platform version the task uses. A platform version is only specified for tasks hosted on Fargate. If one isn't specified, the `LATEST` platform version is used.
*
*/
@Import(name="platformVersion")
private @Nullable String platformVersion;
/**
* @return The platform version the task uses. A platform version is only specified for tasks hosted on Fargate. If one isn't specified, the `LATEST` platform version is used.
*
*/
public Optional platformVersion() {
return Optional.ofNullable(this.platformVersion);
}
/**
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags aren't propagated. An error will be received if you specify the `SERVICE` option when running a task. Valid values are `TASK_DEFINITION` or `NONE`.
*
*/
@Import(name="propagateTags")
private @Nullable String propagateTags;
/**
* @return Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags aren't propagated. An error will be received if you specify the `SERVICE` option when running a task. Valid values are `TASK_DEFINITION` or `NONE`.
*
*/
public Optional propagateTags() {
return Optional.ofNullable(this.propagateTags);
}
/**
* The reference ID to use for the task.
*
*/
@Import(name="referenceId")
private @Nullable String referenceId;
/**
* @return The reference ID to use for the task.
*
*/
public Optional referenceId() {
return Optional.ofNullable(this.referenceId);
}
/**
* An optional tag specified when a task is started.
*
*/
@Import(name="startedBy")
private @Nullable String startedBy;
/**
* @return An optional tag specified when a task is started.
*
*/
public Optional startedBy() {
return Optional.ofNullable(this.startedBy);
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Import(name="tags")
private @Nullable Map tags;
/**
* @return Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy