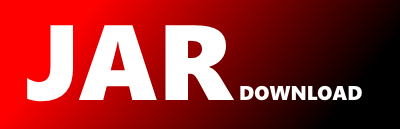
com.pulumi.aws.efs.inputs.ReplicationConfigurationDestinationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.efs.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ReplicationConfigurationDestinationArgs extends com.pulumi.resources.ResourceArgs {
public static final ReplicationConfigurationDestinationArgs Empty = new ReplicationConfigurationDestinationArgs();
/**
* The availability zone in which the replica should be created. If specified, the replica will be created with One Zone storage. If omitted, regional storage will be used.
*
*/
@Import(name="availabilityZoneName")
private @Nullable Output availabilityZoneName;
/**
* @return The availability zone in which the replica should be created. If specified, the replica will be created with One Zone storage. If omitted, regional storage will be used.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy