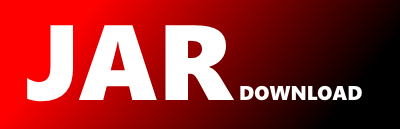
com.pulumi.aws.eks.PodIdentityAssociationArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class PodIdentityAssociationArgs extends com.pulumi.resources.ResourceArgs {
public static final PodIdentityAssociationArgs Empty = new PodIdentityAssociationArgs();
/**
* The name of the cluster to create the association in.
*
*/
@Import(name="clusterName", required=true)
private Output clusterName;
/**
* @return The name of the cluster to create the association in.
*
*/
public Output clusterName() {
return this.clusterName;
}
/**
* The name of the Kubernetes namespace inside the cluster to create the association in. The service account and the pods that use the service account must be in this namespace.
*
*/
@Import(name="namespace", required=true)
private Output namespace;
/**
* @return The name of the Kubernetes namespace inside the cluster to create the association in. The service account and the pods that use the service account must be in this namespace.
*
*/
public Output namespace() {
return this.namespace;
}
/**
* The Amazon Resource Name (ARN) of the IAM role to associate with the service account. The EKS Pod Identity agent manages credentials to assume this role for applications in the containers in the pods that use this service account.
*
*/
@Import(name="roleArn", required=true)
private Output roleArn;
/**
* @return The Amazon Resource Name (ARN) of the IAM role to associate with the service account. The EKS Pod Identity agent manages credentials to assume this role for applications in the containers in the pods that use this service account.
*
*/
public Output roleArn() {
return this.roleArn;
}
/**
* The name of the Kubernetes service account inside the cluster to associate the IAM credentials with.
*
* The following arguments are optional:
*
*/
@Import(name="serviceAccount", required=true)
private Output serviceAccount;
/**
* @return The name of the Kubernetes service account inside the cluster to associate the IAM credentials with.
*
* The following arguments are optional:
*
*/
public Output serviceAccount() {
return this.serviceAccount;
}
/**
* Key-value map of resource tags. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Import(name="tags")
private @Nullable Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy