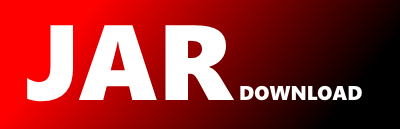
com.pulumi.aws.eks.outputs.GetAccessEntryResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class GetAccessEntryResult {
/**
* @return Amazon Resource Name (ARN) of the Access Entry.
*
*/
private String accessEntryArn;
private String clusterName;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was created.
*
*/
private String createdAt;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return List of string which can optionally specify the Kubernetes groups the user would belong to when creating an access entry.
*
*/
private List kubernetesGroups;
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was updated.
*
*/
private String modifiedAt;
private String principalArn;
private @Nullable Map tags;
/**
* @return (Optional) Key-value map of resource tags, including those inherited from the provider `default_tags` configuration block.
*
*/
private Map tagsAll;
/**
* @return Defaults to STANDARD which provides the standard workflow. EC2_LINUX, EC2_WINDOWS, FARGATE_LINUX types disallow users to input a username or groups, and prevent associations.
*
*/
private String type;
/**
* @return Defaults to principal ARN if user is principal else defaults to assume-role/session-name is role is used.
*
*/
private String userName;
private GetAccessEntryResult() {}
/**
* @return Amazon Resource Name (ARN) of the Access Entry.
*
*/
public String accessEntryArn() {
return this.accessEntryArn;
}
public String clusterName() {
return this.clusterName;
}
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was created.
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return List of string which can optionally specify the Kubernetes groups the user would belong to when creating an access entry.
*
*/
public List kubernetesGroups() {
return this.kubernetesGroups;
}
/**
* @return Date and time in [RFC3339 format](https://tools.ietf.org/html/rfc3339#section-5.8) that the EKS add-on was updated.
*
*/
public String modifiedAt() {
return this.modifiedAt;
}
public String principalArn() {
return this.principalArn;
}
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return (Optional) Key-value map of resource tags, including those inherited from the provider `default_tags` configuration block.
*
*/
public Map tagsAll() {
return this.tagsAll;
}
/**
* @return Defaults to STANDARD which provides the standard workflow. EC2_LINUX, EC2_WINDOWS, FARGATE_LINUX types disallow users to input a username or groups, and prevent associations.
*
*/
public String type() {
return this.type;
}
/**
* @return Defaults to principal ARN if user is principal else defaults to assume-role/session-name is role is used.
*
*/
public String userName() {
return this.userName;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAccessEntryResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String accessEntryArn;
private String clusterName;
private String createdAt;
private String id;
private List kubernetesGroups;
private String modifiedAt;
private String principalArn;
private @Nullable Map tags;
private Map tagsAll;
private String type;
private String userName;
public Builder() {}
public Builder(GetAccessEntryResult defaults) {
Objects.requireNonNull(defaults);
this.accessEntryArn = defaults.accessEntryArn;
this.clusterName = defaults.clusterName;
this.createdAt = defaults.createdAt;
this.id = defaults.id;
this.kubernetesGroups = defaults.kubernetesGroups;
this.modifiedAt = defaults.modifiedAt;
this.principalArn = defaults.principalArn;
this.tags = defaults.tags;
this.tagsAll = defaults.tagsAll;
this.type = defaults.type;
this.userName = defaults.userName;
}
@CustomType.Setter
public Builder accessEntryArn(String accessEntryArn) {
if (accessEntryArn == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "accessEntryArn");
}
this.accessEntryArn = accessEntryArn;
return this;
}
@CustomType.Setter
public Builder clusterName(String clusterName) {
if (clusterName == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "clusterName");
}
this.clusterName = clusterName;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kubernetesGroups(List kubernetesGroups) {
if (kubernetesGroups == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "kubernetesGroups");
}
this.kubernetesGroups = kubernetesGroups;
return this;
}
public Builder kubernetesGroups(String... kubernetesGroups) {
return kubernetesGroups(List.of(kubernetesGroups));
}
@CustomType.Setter
public Builder modifiedAt(String modifiedAt) {
if (modifiedAt == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "modifiedAt");
}
this.modifiedAt = modifiedAt;
return this;
}
@CustomType.Setter
public Builder principalArn(String principalArn) {
if (principalArn == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "principalArn");
}
this.principalArn = principalArn;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tagsAll(Map tagsAll) {
if (tagsAll == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "tagsAll");
}
this.tagsAll = tagsAll;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder userName(String userName) {
if (userName == null) {
throw new MissingRequiredPropertyException("GetAccessEntryResult", "userName");
}
this.userName = userName;
return this;
}
public GetAccessEntryResult build() {
final var _resultValue = new GetAccessEntryResult();
_resultValue.accessEntryArn = accessEntryArn;
_resultValue.clusterName = clusterName;
_resultValue.createdAt = createdAt;
_resultValue.id = id;
_resultValue.kubernetesGroups = kubernetesGroups;
_resultValue.modifiedAt = modifiedAt;
_resultValue.principalArn = principalArn;
_resultValue.tags = tags;
_resultValue.tagsAll = tagsAll;
_resultValue.type = type;
_resultValue.userName = userName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy