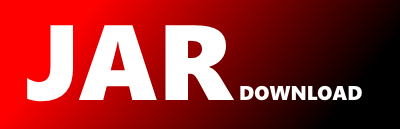
com.pulumi.aws.eks.outputs.GetClusterResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.eks.outputs;
import com.pulumi.aws.eks.outputs.GetClusterAccessConfig;
import com.pulumi.aws.eks.outputs.GetClusterCertificateAuthority;
import com.pulumi.aws.eks.outputs.GetClusterIdentity;
import com.pulumi.aws.eks.outputs.GetClusterKubernetesNetworkConfig;
import com.pulumi.aws.eks.outputs.GetClusterOutpostConfig;
import com.pulumi.aws.eks.outputs.GetClusterUpgradePolicy;
import com.pulumi.aws.eks.outputs.GetClusterVpcConfig;
import com.pulumi.aws.eks.outputs.GetClusterZonalShiftConfig;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetClusterResult {
/**
* @return Configuration block for access config.
*
*/
private List accessConfigs;
/**
* @return ARN of the cluster.
*
*/
private String arn;
/**
* @return Nested attribute containing `certificate-authority-data` for your cluster.
*
*/
private List certificateAuthorities;
/**
* @return The ID of your local Amazon EKS cluster on the AWS Outpost. This attribute isn't available for an AWS EKS cluster on AWS cloud.
*
*/
private String clusterId;
/**
* @return Unix epoch time stamp in seconds for when the cluster was created.
*
*/
private String createdAt;
/**
* @return The enabled control plane logs.
*
*/
private List enabledClusterLogTypes;
/**
* @return Endpoint for your Kubernetes API server.
*
*/
private String endpoint;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return Nested attribute containing identity provider information for your cluster. Only available on Kubernetes version 1.13 and 1.14 clusters created or upgraded on or after September 3, 2019. For an example using this information to enable IAM Roles for Service Accounts, see the `aws.eks.Cluster` resource documentation.
*
*/
private List identities;
/**
* @return Nested list containing Kubernetes Network Configuration.
*
*/
private List kubernetesNetworkConfigs;
private String name;
/**
* @return Contains Outpost Configuration.
*
*/
private List outpostConfigs;
/**
* @return Platform version for the cluster.
*
*/
private String platformVersion;
/**
* @return ARN of the IAM role that provides permissions for the Kubernetes control plane to make calls to AWS API operations on your behalf.
*
*/
private String roleArn;
/**
* @return Status of the EKS cluster. One of `CREATING`, `ACTIVE`, `DELETING`, `FAILED`.
*
*/
private String status;
/**
* @return Key-value map of resource tags.
*
*/
private Map tags;
/**
* @return (Optional) Configuration block for the support policy to use for the cluster.
*
*/
private List upgradePolicies;
/**
* @return Kubernetes server version for the cluster.
*
*/
private String version;
/**
* @return Nested list containing VPC configuration for the cluster.
*
*/
private GetClusterVpcConfig vpcConfig;
/**
* @return Contains Zonal Shift Configuration.
*
*/
private List zonalShiftConfigs;
private GetClusterResult() {}
/**
* @return Configuration block for access config.
*
*/
public List accessConfigs() {
return this.accessConfigs;
}
/**
* @return ARN of the cluster.
*
*/
public String arn() {
return this.arn;
}
/**
* @return Nested attribute containing `certificate-authority-data` for your cluster.
*
*/
public List certificateAuthorities() {
return this.certificateAuthorities;
}
/**
* @return The ID of your local Amazon EKS cluster on the AWS Outpost. This attribute isn't available for an AWS EKS cluster on AWS cloud.
*
*/
public String clusterId() {
return this.clusterId;
}
/**
* @return Unix epoch time stamp in seconds for when the cluster was created.
*
*/
public String createdAt() {
return this.createdAt;
}
/**
* @return The enabled control plane logs.
*
*/
public List enabledClusterLogTypes() {
return this.enabledClusterLogTypes;
}
/**
* @return Endpoint for your Kubernetes API server.
*
*/
public String endpoint() {
return this.endpoint;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return Nested attribute containing identity provider information for your cluster. Only available on Kubernetes version 1.13 and 1.14 clusters created or upgraded on or after September 3, 2019. For an example using this information to enable IAM Roles for Service Accounts, see the `aws.eks.Cluster` resource documentation.
*
*/
public List identities() {
return this.identities;
}
/**
* @return Nested list containing Kubernetes Network Configuration.
*
*/
public List kubernetesNetworkConfigs() {
return this.kubernetesNetworkConfigs;
}
public String name() {
return this.name;
}
/**
* @return Contains Outpost Configuration.
*
*/
public List outpostConfigs() {
return this.outpostConfigs;
}
/**
* @return Platform version for the cluster.
*
*/
public String platformVersion() {
return this.platformVersion;
}
/**
* @return ARN of the IAM role that provides permissions for the Kubernetes control plane to make calls to AWS API operations on your behalf.
*
*/
public String roleArn() {
return this.roleArn;
}
/**
* @return Status of the EKS cluster. One of `CREATING`, `ACTIVE`, `DELETING`, `FAILED`.
*
*/
public String status() {
return this.status;
}
/**
* @return Key-value map of resource tags.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return (Optional) Configuration block for the support policy to use for the cluster.
*
*/
public List upgradePolicies() {
return this.upgradePolicies;
}
/**
* @return Kubernetes server version for the cluster.
*
*/
public String version() {
return this.version;
}
/**
* @return Nested list containing VPC configuration for the cluster.
*
*/
public GetClusterVpcConfig vpcConfig() {
return this.vpcConfig;
}
/**
* @return Contains Zonal Shift Configuration.
*
*/
public List zonalShiftConfigs() {
return this.zonalShiftConfigs;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetClusterResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List accessConfigs;
private String arn;
private List certificateAuthorities;
private String clusterId;
private String createdAt;
private List enabledClusterLogTypes;
private String endpoint;
private String id;
private List identities;
private List kubernetesNetworkConfigs;
private String name;
private List outpostConfigs;
private String platformVersion;
private String roleArn;
private String status;
private Map tags;
private List upgradePolicies;
private String version;
private GetClusterVpcConfig vpcConfig;
private List zonalShiftConfigs;
public Builder() {}
public Builder(GetClusterResult defaults) {
Objects.requireNonNull(defaults);
this.accessConfigs = defaults.accessConfigs;
this.arn = defaults.arn;
this.certificateAuthorities = defaults.certificateAuthorities;
this.clusterId = defaults.clusterId;
this.createdAt = defaults.createdAt;
this.enabledClusterLogTypes = defaults.enabledClusterLogTypes;
this.endpoint = defaults.endpoint;
this.id = defaults.id;
this.identities = defaults.identities;
this.kubernetesNetworkConfigs = defaults.kubernetesNetworkConfigs;
this.name = defaults.name;
this.outpostConfigs = defaults.outpostConfigs;
this.platformVersion = defaults.platformVersion;
this.roleArn = defaults.roleArn;
this.status = defaults.status;
this.tags = defaults.tags;
this.upgradePolicies = defaults.upgradePolicies;
this.version = defaults.version;
this.vpcConfig = defaults.vpcConfig;
this.zonalShiftConfigs = defaults.zonalShiftConfigs;
}
@CustomType.Setter
public Builder accessConfigs(List accessConfigs) {
if (accessConfigs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "accessConfigs");
}
this.accessConfigs = accessConfigs;
return this;
}
public Builder accessConfigs(GetClusterAccessConfig... accessConfigs) {
return accessConfigs(List.of(accessConfigs));
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder certificateAuthorities(List certificateAuthorities) {
if (certificateAuthorities == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "certificateAuthorities");
}
this.certificateAuthorities = certificateAuthorities;
return this;
}
public Builder certificateAuthorities(GetClusterCertificateAuthority... certificateAuthorities) {
return certificateAuthorities(List.of(certificateAuthorities));
}
@CustomType.Setter
public Builder clusterId(String clusterId) {
if (clusterId == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "clusterId");
}
this.clusterId = clusterId;
return this;
}
@CustomType.Setter
public Builder createdAt(String createdAt) {
if (createdAt == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "createdAt");
}
this.createdAt = createdAt;
return this;
}
@CustomType.Setter
public Builder enabledClusterLogTypes(List enabledClusterLogTypes) {
if (enabledClusterLogTypes == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "enabledClusterLogTypes");
}
this.enabledClusterLogTypes = enabledClusterLogTypes;
return this;
}
public Builder enabledClusterLogTypes(String... enabledClusterLogTypes) {
return enabledClusterLogTypes(List.of(enabledClusterLogTypes));
}
@CustomType.Setter
public Builder endpoint(String endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder identities(List identities) {
if (identities == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "identities");
}
this.identities = identities;
return this;
}
public Builder identities(GetClusterIdentity... identities) {
return identities(List.of(identities));
}
@CustomType.Setter
public Builder kubernetesNetworkConfigs(List kubernetesNetworkConfigs) {
if (kubernetesNetworkConfigs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "kubernetesNetworkConfigs");
}
this.kubernetesNetworkConfigs = kubernetesNetworkConfigs;
return this;
}
public Builder kubernetesNetworkConfigs(GetClusterKubernetesNetworkConfig... kubernetesNetworkConfigs) {
return kubernetesNetworkConfigs(List.of(kubernetesNetworkConfigs));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder outpostConfigs(List outpostConfigs) {
if (outpostConfigs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "outpostConfigs");
}
this.outpostConfigs = outpostConfigs;
return this;
}
public Builder outpostConfigs(GetClusterOutpostConfig... outpostConfigs) {
return outpostConfigs(List.of(outpostConfigs));
}
@CustomType.Setter
public Builder platformVersion(String platformVersion) {
if (platformVersion == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "platformVersion");
}
this.platformVersion = platformVersion;
return this;
}
@CustomType.Setter
public Builder roleArn(String roleArn) {
if (roleArn == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "roleArn");
}
this.roleArn = roleArn;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder upgradePolicies(List upgradePolicies) {
if (upgradePolicies == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "upgradePolicies");
}
this.upgradePolicies = upgradePolicies;
return this;
}
public Builder upgradePolicies(GetClusterUpgradePolicy... upgradePolicies) {
return upgradePolicies(List.of(upgradePolicies));
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "version");
}
this.version = version;
return this;
}
@CustomType.Setter
public Builder vpcConfig(GetClusterVpcConfig vpcConfig) {
if (vpcConfig == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "vpcConfig");
}
this.vpcConfig = vpcConfig;
return this;
}
@CustomType.Setter
public Builder zonalShiftConfigs(List zonalShiftConfigs) {
if (zonalShiftConfigs == null) {
throw new MissingRequiredPropertyException("GetClusterResult", "zonalShiftConfigs");
}
this.zonalShiftConfigs = zonalShiftConfigs;
return this;
}
public Builder zonalShiftConfigs(GetClusterZonalShiftConfig... zonalShiftConfigs) {
return zonalShiftConfigs(List.of(zonalShiftConfigs));
}
public GetClusterResult build() {
final var _resultValue = new GetClusterResult();
_resultValue.accessConfigs = accessConfigs;
_resultValue.arn = arn;
_resultValue.certificateAuthorities = certificateAuthorities;
_resultValue.clusterId = clusterId;
_resultValue.createdAt = createdAt;
_resultValue.enabledClusterLogTypes = enabledClusterLogTypes;
_resultValue.endpoint = endpoint;
_resultValue.id = id;
_resultValue.identities = identities;
_resultValue.kubernetesNetworkConfigs = kubernetesNetworkConfigs;
_resultValue.name = name;
_resultValue.outpostConfigs = outpostConfigs;
_resultValue.platformVersion = platformVersion;
_resultValue.roleArn = roleArn;
_resultValue.status = status;
_resultValue.tags = tags;
_resultValue.upgradePolicies = upgradePolicies;
_resultValue.version = version;
_resultValue.vpcConfig = vpcConfig;
_resultValue.zonalShiftConfigs = zonalShiftConfigs;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy