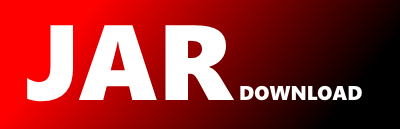
com.pulumi.aws.elasticache.ServerlessCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticache;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.elasticache.ServerlessCacheArgs;
import com.pulumi.aws.elasticache.inputs.ServerlessCacheState;
import com.pulumi.aws.elasticache.outputs.ServerlessCacheCacheUsageLimits;
import com.pulumi.aws.elasticache.outputs.ServerlessCacheEndpoint;
import com.pulumi.aws.elasticache.outputs.ServerlessCacheReaderEndpoint;
import com.pulumi.aws.elasticache.outputs.ServerlessCacheTimeouts;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Provides an ElastiCache Serverless Cache resource which manages memcached, redis or valkey.
*
* ## Example Usage
*
* ### Memcached Serverless
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticache.ServerlessCache;
* import com.pulumi.aws.elasticache.ServerlessCacheArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsDataStorageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ServerlessCache("example", ServerlessCacheArgs.builder()
* .engine("memcached")
* .name("example")
* .cacheUsageLimits(ServerlessCacheCacheUsageLimitsArgs.builder()
* .dataStorage(ServerlessCacheCacheUsageLimitsDataStorageArgs.builder()
* .maximum(10)
* .unit("GB")
* .build())
* .ecpuPerSeconds(ServerlessCacheCacheUsageLimitsEcpuPerSecondArgs.builder()
* .maximum(5000)
* .build())
* .build())
* .description("Test Server")
* .kmsKeyId(test.arn())
* .majorEngineVersion("1.6")
* .securityGroupIds(testAwsSecurityGroup.id())
* .subnetIds(testAwsSubnet.stream().map(element -> element.id()).collect(toList()))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Redis OSS Serverless
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticache.ServerlessCache;
* import com.pulumi.aws.elasticache.ServerlessCacheArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsDataStorageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ServerlessCache("example", ServerlessCacheArgs.builder()
* .engine("redis")
* .name("example")
* .cacheUsageLimits(ServerlessCacheCacheUsageLimitsArgs.builder()
* .dataStorage(ServerlessCacheCacheUsageLimitsDataStorageArgs.builder()
* .maximum(10)
* .unit("GB")
* .build())
* .ecpuPerSeconds(ServerlessCacheCacheUsageLimitsEcpuPerSecondArgs.builder()
* .maximum(5000)
* .build())
* .build())
* .dailySnapshotTime("09:00")
* .description("Test Server")
* .kmsKeyId(test.arn())
* .majorEngineVersion("7")
* .snapshotRetentionLimit(1)
* .securityGroupIds(testAwsSecurityGroup.id())
* .subnetIds(testAwsSubnet.stream().map(element -> element.id()).collect(toList()))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Valkey Serverless
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.elasticache.ServerlessCache;
* import com.pulumi.aws.elasticache.ServerlessCacheArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsArgs;
* import com.pulumi.aws.elasticache.inputs.ServerlessCacheCacheUsageLimitsDataStorageArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ServerlessCache("example", ServerlessCacheArgs.builder()
* .engine("valkey")
* .name("example")
* .cacheUsageLimits(ServerlessCacheCacheUsageLimitsArgs.builder()
* .dataStorage(ServerlessCacheCacheUsageLimitsDataStorageArgs.builder()
* .maximum(10)
* .unit("GB")
* .build())
* .ecpuPerSeconds(ServerlessCacheCacheUsageLimitsEcpuPerSecondArgs.builder()
* .maximum(5000)
* .build())
* .build())
* .dailySnapshotTime("09:00")
* .description("Test Server")
* .kmsKeyId(test.arn())
* .majorEngineVersion("7")
* .snapshotRetentionLimit(1)
* .securityGroupIds(testAwsSecurityGroup.id())
* .subnetIds(testAwsSubnet.stream().map(element -> element.id()).collect(toList()))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import ElastiCache Serverless Cache using the `name`. For example:
*
* ```sh
* $ pulumi import aws:elasticache/serverlessCache:ServerlessCache my_cluster my_cluster
* ```
*
*/
@ResourceType(type="aws:elasticache/serverlessCache:ServerlessCache")
public class ServerlessCache extends com.pulumi.resources.CustomResource {
/**
* The Amazon Resource Name (ARN) of the serverless cache.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return The Amazon Resource Name (ARN) of the serverless cache.
*
*/
public Output arn() {
return this.arn;
}
/**
* Sets the cache usage limits for storage and ElastiCache Processing Units for the cache. See `cache_usage_limits` Block for details.
*
*/
@Export(name="cacheUsageLimits", refs={ServerlessCacheCacheUsageLimits.class}, tree="[0]")
private Output* @Nullable */ ServerlessCacheCacheUsageLimits> cacheUsageLimits;
/**
* @return Sets the cache usage limits for storage and ElastiCache Processing Units for the cache. See `cache_usage_limits` Block for details.
*
*/
public Output> cacheUsageLimits() {
return Codegen.optional(this.cacheUsageLimits);
}
/**
* Timestamp of when the serverless cache was created.
*
*/
@Export(name="createTime", refs={String.class}, tree="[0]")
private Output createTime;
/**
* @return Timestamp of when the serverless cache was created.
*
*/
public Output createTime() {
return this.createTime;
}
/**
* The daily time that snapshots will be created from the new serverless cache. Only supported for engine types `"redis"` or `"valkey"`. Defaults to `0`.
*
*/
@Export(name="dailySnapshotTime", refs={String.class}, tree="[0]")
private Output dailySnapshotTime;
/**
* @return The daily time that snapshots will be created from the new serverless cache. Only supported for engine types `"redis"` or `"valkey"`. Defaults to `0`.
*
*/
public Output dailySnapshotTime() {
return this.dailySnapshotTime;
}
/**
* User-provided description for the serverless cache. The default is NULL.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output description;
/**
* @return User-provided description for the serverless cache. The default is NULL.
*
*/
public Output description() {
return this.description;
}
/**
* Represents the information required for client programs to connect to a cache node. See `endpoint` Block for details.
*
*/
@Export(name="endpoints", refs={List.class,ServerlessCacheEndpoint.class}, tree="[0,1]")
private Output> endpoints;
/**
* @return Represents the information required for client programs to connect to a cache node. See `endpoint` Block for details.
*
*/
public Output> endpoints() {
return this.endpoints;
}
/**
* Name of the cache engine to be used for this cache cluster. Valid values are `memcached`, `redis` or `valkey`.
*
*/
@Export(name="engine", refs={String.class}, tree="[0]")
private Output engine;
/**
* @return Name of the cache engine to be used for this cache cluster. Valid values are `memcached`, `redis` or `valkey`.
*
*/
public Output engine() {
return this.engine;
}
/**
* The name and version number of the engine the serverless cache is compatible with.
*
*/
@Export(name="fullEngineVersion", refs={String.class}, tree="[0]")
private Output fullEngineVersion;
/**
* @return The name and version number of the engine the serverless cache is compatible with.
*
*/
public Output fullEngineVersion() {
return this.fullEngineVersion;
}
/**
* ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key is used.
*
*/
@Export(name="kmsKeyId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> kmsKeyId;
/**
* @return ARN of the customer managed key for encrypting the data at rest. If no KMS key is provided, a default service key is used.
*
*/
public Output> kmsKeyId() {
return Codegen.optional(this.kmsKeyId);
}
/**
* The version of the cache engine that will be used to create the serverless cache.
* See [Describe Cache Engine Versions](https://docs.aws.amazon.com/cli/latest/reference/elasticache/describe-cache-engine-versions.html) in the AWS Documentation for supported versions.
*
*/
@Export(name="majorEngineVersion", refs={String.class}, tree="[0]")
private Output majorEngineVersion;
/**
* @return The version of the cache engine that will be used to create the serverless cache.
* See [Describe Cache Engine Versions](https://docs.aws.amazon.com/cli/latest/reference/elasticache/describe-cache-engine-versions.html) in the AWS Documentation for supported versions.
*
*/
public Output majorEngineVersion() {
return this.majorEngineVersion;
}
/**
* The Cluster name which serves as a unique identifier to the serverless cache
*
* The following arguments are optional:
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The Cluster name which serves as a unique identifier to the serverless cache
*
* The following arguments are optional:
*
*/
public Output name() {
return this.name;
}
/**
* Represents the information required for client programs to connect to a cache node. See `reader_endpoint` Block for details.
*
*/
@Export(name="readerEndpoints", refs={List.class,ServerlessCacheReaderEndpoint.class}, tree="[0,1]")
private Output> readerEndpoints;
/**
* @return Represents the information required for client programs to connect to a cache node. See `reader_endpoint` Block for details.
*
*/
public Output> readerEndpoints() {
return this.readerEndpoints;
}
/**
* A list of the one or more VPC security groups to be associated with the serverless cache. The security group will authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*/
@Export(name="securityGroupIds", refs={List.class,String.class}, tree="[0,1]")
private Output> securityGroupIds;
/**
* @return A list of the one or more VPC security groups to be associated with the serverless cache. The security group will authorize traffic access for the VPC end-point (private-link). If no other information is given this will be the VPC’s Default Security Group that is associated with the cluster VPC end-point.
*
*/
public Output> securityGroupIds() {
return this.securityGroupIds;
}
/**
* The list of ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis only.
*
*/
@Export(name="snapshotArnsToRestores", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> snapshotArnsToRestores;
/**
* @return The list of ARN(s) of the snapshot that the new serverless cache will be created from. Available for Redis only.
*
*/
public Output>> snapshotArnsToRestores() {
return Codegen.optional(this.snapshotArnsToRestores);
}
/**
* The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis only.
*
*/
@Export(name="snapshotRetentionLimit", refs={Integer.class}, tree="[0]")
private Output snapshotRetentionLimit;
/**
* @return The number of snapshots that will be retained for the serverless cache that is being created. As new snapshots beyond this limit are added, the oldest snapshots will be deleted on a rolling basis. Available for Redis only.
*
*/
public Output snapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
* The current status of the serverless cache. The allowed values are CREATING, AVAILABLE, DELETING, CREATE-FAILED and MODIFYING.
*
*/
@Export(name="status", refs={String.class}, tree="[0]")
private Output status;
/**
* @return The current status of the serverless cache. The allowed values are CREATING, AVAILABLE, DELETING, CREATE-FAILED and MODIFYING.
*
*/
public Output status() {
return this.status;
}
/**
* A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All the subnetIds must belong to the same VPC.
*
*/
@Export(name="subnetIds", refs={List.class,String.class}, tree="[0,1]")
private Output> subnetIds;
/**
* @return A list of the identifiers of the subnets where the VPC endpoint for the serverless cache will be deployed. All the subnetIds must belong to the same VPC.
*
*/
public Output> subnetIds() {
return this.subnetIds;
}
/**
* Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return Map of tags to assign to the resource. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy