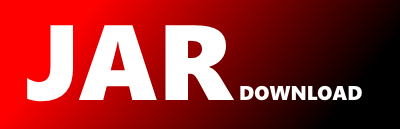
com.pulumi.aws.elasticache.outputs.GetServerlessCacheResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticache.outputs;
import com.pulumi.aws.elasticache.outputs.GetServerlessCacheCacheUsageLimits;
import com.pulumi.aws.elasticache.outputs.GetServerlessCacheEndpoint;
import com.pulumi.aws.elasticache.outputs.GetServerlessCacheReaderEndpoint;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class GetServerlessCacheResult {
/**
* @return The Amazon Resource Name (ARN) of the serverless cache.
*
*/
private String arn;
/**
* @return The cache usage limits for storage and ElastiCache Processing Units for the cache. See `cache_usage_limits` Block for details.
*
*/
private GetServerlessCacheCacheUsageLimits cacheUsageLimits;
/**
* @return Timestamp of when the serverless cache was created.
*
*/
private String createTime;
/**
* @return The daily time that snapshots will be created from the new serverless cache. Only available for engine types `"redis"` and `"valkey"`.
*
*/
private String dailySnapshotTime;
/**
* @return Description of the serverless cache.
*
*/
private String description;
/**
* @return Represents the information required for client programs to connect to the cache. See `endpoint` Block for details.
*
*/
private GetServerlessCacheEndpoint endpoint;
/**
* @return Name of the cache engine.
*
*/
private String engine;
/**
* @return The name and version number of the engine the serverless cache is compatible with.
*
*/
private String fullEngineVersion;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
/**
* @return ARN of the customer managed key for encrypting the data at rest.
*
*/
private String kmsKeyId;
/**
* @return The version number of the engine the serverless cache is compatible with.
*
*/
private String majorEngineVersion;
private String name;
/**
* @return Represents the information required for client programs to connect to a cache node. See `reader_endpoint` Block for details.
*
*/
private GetServerlessCacheReaderEndpoint readerEndpoint;
/**
* @return A list of the one or more VPC security groups associated with the serverless cache.
*
*/
private List securityGroupIds;
/**
* @return The number of snapshots that will be retained for the serverless cache. Available for Redis only.
*
*/
private Integer snapshotRetentionLimit;
/**
* @return The current status of the serverless cache.
*
*/
private String status;
/**
* @return A list of the identifiers of the subnets where the VPC endpoint for the serverless cache are deployed.
*
*/
private List subnetIds;
/**
* @return The identifier of the UserGroup associated with the serverless cache. Available for Redis only.
*
*/
private String userGroupId;
private GetServerlessCacheResult() {}
/**
* @return The Amazon Resource Name (ARN) of the serverless cache.
*
*/
public String arn() {
return this.arn;
}
/**
* @return The cache usage limits for storage and ElastiCache Processing Units for the cache. See `cache_usage_limits` Block for details.
*
*/
public GetServerlessCacheCacheUsageLimits cacheUsageLimits() {
return this.cacheUsageLimits;
}
/**
* @return Timestamp of when the serverless cache was created.
*
*/
public String createTime() {
return this.createTime;
}
/**
* @return The daily time that snapshots will be created from the new serverless cache. Only available for engine types `"redis"` and `"valkey"`.
*
*/
public String dailySnapshotTime() {
return this.dailySnapshotTime;
}
/**
* @return Description of the serverless cache.
*
*/
public String description() {
return this.description;
}
/**
* @return Represents the information required for client programs to connect to the cache. See `endpoint` Block for details.
*
*/
public GetServerlessCacheEndpoint endpoint() {
return this.endpoint;
}
/**
* @return Name of the cache engine.
*
*/
public String engine() {
return this.engine;
}
/**
* @return The name and version number of the engine the serverless cache is compatible with.
*
*/
public String fullEngineVersion() {
return this.fullEngineVersion;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
/**
* @return ARN of the customer managed key for encrypting the data at rest.
*
*/
public String kmsKeyId() {
return this.kmsKeyId;
}
/**
* @return The version number of the engine the serverless cache is compatible with.
*
*/
public String majorEngineVersion() {
return this.majorEngineVersion;
}
public String name() {
return this.name;
}
/**
* @return Represents the information required for client programs to connect to a cache node. See `reader_endpoint` Block for details.
*
*/
public GetServerlessCacheReaderEndpoint readerEndpoint() {
return this.readerEndpoint;
}
/**
* @return A list of the one or more VPC security groups associated with the serverless cache.
*
*/
public List securityGroupIds() {
return this.securityGroupIds;
}
/**
* @return The number of snapshots that will be retained for the serverless cache. Available for Redis only.
*
*/
public Integer snapshotRetentionLimit() {
return this.snapshotRetentionLimit;
}
/**
* @return The current status of the serverless cache.
*
*/
public String status() {
return this.status;
}
/**
* @return A list of the identifiers of the subnets where the VPC endpoint for the serverless cache are deployed.
*
*/
public List subnetIds() {
return this.subnetIds;
}
/**
* @return The identifier of the UserGroup associated with the serverless cache. Available for Redis only.
*
*/
public String userGroupId() {
return this.userGroupId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetServerlessCacheResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private GetServerlessCacheCacheUsageLimits cacheUsageLimits;
private String createTime;
private String dailySnapshotTime;
private String description;
private GetServerlessCacheEndpoint endpoint;
private String engine;
private String fullEngineVersion;
private String id;
private String kmsKeyId;
private String majorEngineVersion;
private String name;
private GetServerlessCacheReaderEndpoint readerEndpoint;
private List securityGroupIds;
private Integer snapshotRetentionLimit;
private String status;
private List subnetIds;
private String userGroupId;
public Builder() {}
public Builder(GetServerlessCacheResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.cacheUsageLimits = defaults.cacheUsageLimits;
this.createTime = defaults.createTime;
this.dailySnapshotTime = defaults.dailySnapshotTime;
this.description = defaults.description;
this.endpoint = defaults.endpoint;
this.engine = defaults.engine;
this.fullEngineVersion = defaults.fullEngineVersion;
this.id = defaults.id;
this.kmsKeyId = defaults.kmsKeyId;
this.majorEngineVersion = defaults.majorEngineVersion;
this.name = defaults.name;
this.readerEndpoint = defaults.readerEndpoint;
this.securityGroupIds = defaults.securityGroupIds;
this.snapshotRetentionLimit = defaults.snapshotRetentionLimit;
this.status = defaults.status;
this.subnetIds = defaults.subnetIds;
this.userGroupId = defaults.userGroupId;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder cacheUsageLimits(GetServerlessCacheCacheUsageLimits cacheUsageLimits) {
if (cacheUsageLimits == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "cacheUsageLimits");
}
this.cacheUsageLimits = cacheUsageLimits;
return this;
}
@CustomType.Setter
public Builder createTime(String createTime) {
if (createTime == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "createTime");
}
this.createTime = createTime;
return this;
}
@CustomType.Setter
public Builder dailySnapshotTime(String dailySnapshotTime) {
if (dailySnapshotTime == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "dailySnapshotTime");
}
this.dailySnapshotTime = dailySnapshotTime;
return this;
}
@CustomType.Setter
public Builder description(String description) {
if (description == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "description");
}
this.description = description;
return this;
}
@CustomType.Setter
public Builder endpoint(GetServerlessCacheEndpoint endpoint) {
if (endpoint == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "endpoint");
}
this.endpoint = endpoint;
return this;
}
@CustomType.Setter
public Builder engine(String engine) {
if (engine == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "engine");
}
this.engine = engine;
return this;
}
@CustomType.Setter
public Builder fullEngineVersion(String fullEngineVersion) {
if (fullEngineVersion == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "fullEngineVersion");
}
this.fullEngineVersion = fullEngineVersion;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kmsKeyId(String kmsKeyId) {
if (kmsKeyId == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "kmsKeyId");
}
this.kmsKeyId = kmsKeyId;
return this;
}
@CustomType.Setter
public Builder majorEngineVersion(String majorEngineVersion) {
if (majorEngineVersion == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "majorEngineVersion");
}
this.majorEngineVersion = majorEngineVersion;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder readerEndpoint(GetServerlessCacheReaderEndpoint readerEndpoint) {
if (readerEndpoint == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "readerEndpoint");
}
this.readerEndpoint = readerEndpoint;
return this;
}
@CustomType.Setter
public Builder securityGroupIds(List securityGroupIds) {
if (securityGroupIds == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "securityGroupIds");
}
this.securityGroupIds = securityGroupIds;
return this;
}
public Builder securityGroupIds(String... securityGroupIds) {
return securityGroupIds(List.of(securityGroupIds));
}
@CustomType.Setter
public Builder snapshotRetentionLimit(Integer snapshotRetentionLimit) {
if (snapshotRetentionLimit == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "snapshotRetentionLimit");
}
this.snapshotRetentionLimit = snapshotRetentionLimit;
return this;
}
@CustomType.Setter
public Builder status(String status) {
if (status == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "status");
}
this.status = status;
return this;
}
@CustomType.Setter
public Builder subnetIds(List subnetIds) {
if (subnetIds == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "subnetIds");
}
this.subnetIds = subnetIds;
return this;
}
public Builder subnetIds(String... subnetIds) {
return subnetIds(List.of(subnetIds));
}
@CustomType.Setter
public Builder userGroupId(String userGroupId) {
if (userGroupId == null) {
throw new MissingRequiredPropertyException("GetServerlessCacheResult", "userGroupId");
}
this.userGroupId = userGroupId;
return this;
}
public GetServerlessCacheResult build() {
final var _resultValue = new GetServerlessCacheResult();
_resultValue.arn = arn;
_resultValue.cacheUsageLimits = cacheUsageLimits;
_resultValue.createTime = createTime;
_resultValue.dailySnapshotTime = dailySnapshotTime;
_resultValue.description = description;
_resultValue.endpoint = endpoint;
_resultValue.engine = engine;
_resultValue.fullEngineVersion = fullEngineVersion;
_resultValue.id = id;
_resultValue.kmsKeyId = kmsKeyId;
_resultValue.majorEngineVersion = majorEngineVersion;
_resultValue.name = name;
_resultValue.readerEndpoint = readerEndpoint;
_resultValue.securityGroupIds = securityGroupIds;
_resultValue.snapshotRetentionLimit = snapshotRetentionLimit;
_resultValue.status = status;
_resultValue.subnetIds = subnetIds;
_resultValue.userGroupId = userGroupId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy