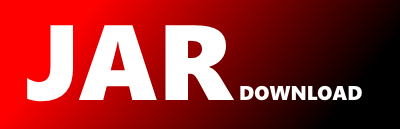
com.pulumi.aws.elasticsearch.inputs.DomainClusterConfigArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elasticsearch.inputs;
import com.pulumi.aws.elasticsearch.inputs.DomainClusterConfigColdStorageOptionsArgs;
import com.pulumi.aws.elasticsearch.inputs.DomainClusterConfigZoneAwarenessConfigArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DomainClusterConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final DomainClusterConfigArgs Empty = new DomainClusterConfigArgs();
/**
* Configuration block containing cold storage configuration. Detailed below.
*
*/
@Import(name="coldStorageOptions")
private @Nullable Output coldStorageOptions;
/**
* @return Configuration block containing cold storage configuration. Detailed below.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy