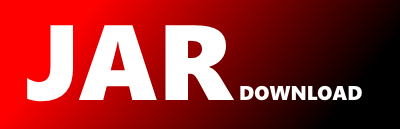
com.pulumi.aws.elastictranscoder.outputs.PresetVideo Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.elastictranscoder.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class PresetVideo {
/**
* @return The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
*
*/
private @Nullable String aspectRatio;
/**
* @return The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
*
*/
private @Nullable String bitRate;
/**
* @return The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
*
*/
private @Nullable String codec;
/**
* @return The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
*
*/
private @Nullable String displayAspectRatio;
/**
* @return Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
*
*/
private @Nullable String fixedGop;
/**
* @return The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
*
*/
private @Nullable String frameRate;
/**
* @return The maximum number of frames between key frames. Not applicable for containers of type gif.
*
*/
private @Nullable String keyframesMaxDist;
/**
* @return If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
*
*/
private @Nullable String maxFrameRate;
/**
* @return The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
*
*/
private @Nullable String maxHeight;
/**
* @return The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
*
*/
private @Nullable String maxWidth;
/**
* @return When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
*
*/
private @Nullable String paddingPolicy;
/**
* @return The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
*
*/
private @Nullable String resolution;
/**
* @return A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*
*/
private @Nullable String sizingPolicy;
private PresetVideo() {}
/**
* @return The display aspect ratio of the video in the output file. Valid values are: `auto`, `1:1`, `4:3`, `3:2`, `16:9`. (Note; to better control resolution and aspect ratio of output videos, we recommend that you use the values `max_width`, `max_height`, `sizing_policy`, `padding_policy`, and `display_aspect_ratio` instead of `resolution` and `aspect_ratio`.)
*
*/
public Optional aspectRatio() {
return Optional.ofNullable(this.aspectRatio);
}
/**
* @return The bit rate of the video stream in the output file, in kilobits/second. You can configure variable bit rate or constant bit rate encoding.
*
*/
public Optional bitRate() {
return Optional.ofNullable(this.bitRate);
}
/**
* @return The video codec for the output file. Valid values are `gif`, `H.264`, `mpeg2`, `vp8`, and `vp9`.
*
*/
public Optional codec() {
return Optional.ofNullable(this.codec);
}
/**
* @return The value that Elastic Transcoder adds to the metadata in the output file. If you set DisplayAspectRatio to auto, Elastic Transcoder chooses an aspect ratio that ensures square pixels. If you specify another option, Elastic Transcoder sets that value in the output file.
*
*/
public Optional displayAspectRatio() {
return Optional.ofNullable(this.displayAspectRatio);
}
/**
* @return Whether to use a fixed value for Video:FixedGOP. Not applicable for containers of type gif. Valid values are true and false. Also known as, Fixed Number of Frames Between Keyframes.
*
*/
public Optional fixedGop() {
return Optional.ofNullable(this.fixedGop);
}
/**
* @return The frames per second for the video stream in the output file. The following values are valid: `auto`, `10`, `15`, `23.97`, `24`, `25`, `29.97`, `30`, `50`, `60`.
*
*/
public Optional frameRate() {
return Optional.ofNullable(this.frameRate);
}
/**
* @return The maximum number of frames between key frames. Not applicable for containers of type gif.
*
*/
public Optional keyframesMaxDist() {
return Optional.ofNullable(this.keyframesMaxDist);
}
/**
* @return If you specify auto for FrameRate, Elastic Transcoder uses the frame rate of the input video for the frame rate of the output video, up to the maximum frame rate. If you do not specify a MaxFrameRate, Elastic Transcoder will use a default of 30.
*
*/
public Optional maxFrameRate() {
return Optional.ofNullable(this.maxFrameRate);
}
/**
* @return The maximum height of the output video in pixels. If you specify auto, Elastic Transcoder uses 1080 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 96 and 3072, inclusive.
*
*/
public Optional maxHeight() {
return Optional.ofNullable(this.maxHeight);
}
/**
* @return The maximum width of the output video in pixels. If you specify auto, Elastic Transcoder uses 1920 (Full HD) as the default value. If you specify a numeric value, enter an even integer between 128 and 4096, inclusive.
*
*/
public Optional maxWidth() {
return Optional.ofNullable(this.maxWidth);
}
/**
* @return When you set PaddingPolicy to Pad, Elastic Transcoder might add black bars to the top and bottom and/or left and right sides of the output video to make the total size of the output video match the values that you specified for `max_width` and `max_height`.
*
*/
public Optional paddingPolicy() {
return Optional.ofNullable(this.paddingPolicy);
}
/**
* @return The width and height of the video in the output file, in pixels. Valid values are `auto` and `widthxheight`. (see note for `aspect_ratio`)
*
*/
public Optional resolution() {
return Optional.ofNullable(this.resolution);
}
/**
* @return A value that controls scaling of the output video. Valid values are: `Fit`, `Fill`, `Stretch`, `Keep`, `ShrinkToFit`, `ShrinkToFill`.
*
*/
public Optional sizingPolicy() {
return Optional.ofNullable(this.sizingPolicy);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(PresetVideo defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String aspectRatio;
private @Nullable String bitRate;
private @Nullable String codec;
private @Nullable String displayAspectRatio;
private @Nullable String fixedGop;
private @Nullable String frameRate;
private @Nullable String keyframesMaxDist;
private @Nullable String maxFrameRate;
private @Nullable String maxHeight;
private @Nullable String maxWidth;
private @Nullable String paddingPolicy;
private @Nullable String resolution;
private @Nullable String sizingPolicy;
public Builder() {}
public Builder(PresetVideo defaults) {
Objects.requireNonNull(defaults);
this.aspectRatio = defaults.aspectRatio;
this.bitRate = defaults.bitRate;
this.codec = defaults.codec;
this.displayAspectRatio = defaults.displayAspectRatio;
this.fixedGop = defaults.fixedGop;
this.frameRate = defaults.frameRate;
this.keyframesMaxDist = defaults.keyframesMaxDist;
this.maxFrameRate = defaults.maxFrameRate;
this.maxHeight = defaults.maxHeight;
this.maxWidth = defaults.maxWidth;
this.paddingPolicy = defaults.paddingPolicy;
this.resolution = defaults.resolution;
this.sizingPolicy = defaults.sizingPolicy;
}
@CustomType.Setter
public Builder aspectRatio(@Nullable String aspectRatio) {
this.aspectRatio = aspectRatio;
return this;
}
@CustomType.Setter
public Builder bitRate(@Nullable String bitRate) {
this.bitRate = bitRate;
return this;
}
@CustomType.Setter
public Builder codec(@Nullable String codec) {
this.codec = codec;
return this;
}
@CustomType.Setter
public Builder displayAspectRatio(@Nullable String displayAspectRatio) {
this.displayAspectRatio = displayAspectRatio;
return this;
}
@CustomType.Setter
public Builder fixedGop(@Nullable String fixedGop) {
this.fixedGop = fixedGop;
return this;
}
@CustomType.Setter
public Builder frameRate(@Nullable String frameRate) {
this.frameRate = frameRate;
return this;
}
@CustomType.Setter
public Builder keyframesMaxDist(@Nullable String keyframesMaxDist) {
this.keyframesMaxDist = keyframesMaxDist;
return this;
}
@CustomType.Setter
public Builder maxFrameRate(@Nullable String maxFrameRate) {
this.maxFrameRate = maxFrameRate;
return this;
}
@CustomType.Setter
public Builder maxHeight(@Nullable String maxHeight) {
this.maxHeight = maxHeight;
return this;
}
@CustomType.Setter
public Builder maxWidth(@Nullable String maxWidth) {
this.maxWidth = maxWidth;
return this;
}
@CustomType.Setter
public Builder paddingPolicy(@Nullable String paddingPolicy) {
this.paddingPolicy = paddingPolicy;
return this;
}
@CustomType.Setter
public Builder resolution(@Nullable String resolution) {
this.resolution = resolution;
return this;
}
@CustomType.Setter
public Builder sizingPolicy(@Nullable String sizingPolicy) {
this.sizingPolicy = sizingPolicy;
return this;
}
public PresetVideo build() {
final var _resultValue = new PresetVideo();
_resultValue.aspectRatio = aspectRatio;
_resultValue.bitRate = bitRate;
_resultValue.codec = codec;
_resultValue.displayAspectRatio = displayAspectRatio;
_resultValue.fixedGop = fixedGop;
_resultValue.frameRate = frameRate;
_resultValue.keyframesMaxDist = keyframesMaxDist;
_resultValue.maxFrameRate = maxFrameRate;
_resultValue.maxHeight = maxHeight;
_resultValue.maxWidth = maxWidth;
_resultValue.paddingPolicy = paddingPolicy;
_resultValue.resolution = resolution;
_resultValue.sizingPolicy = sizingPolicy;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy