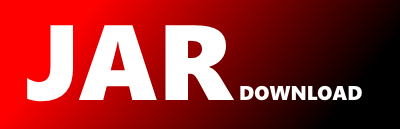
com.pulumi.aws.emr.outputs.ManagedScalingPolicyComputeLimit Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emr.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ManagedScalingPolicyComputeLimit {
/**
* @return The upper boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*/
private Integer maximumCapacityUnits;
/**
* @return The upper boundary of EC2 units for core node type in a cluster. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The core units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
*
*/
private @Nullable Integer maximumCoreCapacityUnits;
/**
* @return The upper boundary of On-Demand EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot instances.
*
*/
private @Nullable Integer maximumOndemandCapacityUnits;
/**
* @return The lower boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*/
private Integer minimumCapacityUnits;
/**
* @return The unit type used for specifying a managed scaling policy. Valid Values: `InstanceFleetUnits` | `Instances` | `VCPU`
*
*/
private String unitType;
private ManagedScalingPolicyComputeLimit() {}
/**
* @return The upper boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*/
public Integer maximumCapacityUnits() {
return this.maximumCapacityUnits;
}
/**
* @return The upper boundary of EC2 units for core node type in a cluster. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The core units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between core and task nodes.
*
*/
public Optional maximumCoreCapacityUnits() {
return Optional.ofNullable(this.maximumCoreCapacityUnits);
}
/**
* @return The upper boundary of On-Demand EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. The On-Demand units are not allowed to scale beyond this boundary. The parameter is used to split capacity allocation between On-Demand and Spot instances.
*
*/
public Optional maximumOndemandCapacityUnits() {
return Optional.ofNullable(this.maximumOndemandCapacityUnits);
}
/**
* @return The lower boundary of EC2 units. It is measured through VCPU cores or instances for instance groups and measured through units for instance fleets. Managed scaling activities are not allowed beyond this boundary. The limit only applies to the core and task nodes. The master node cannot be scaled after initial configuration.
*
*/
public Integer minimumCapacityUnits() {
return this.minimumCapacityUnits;
}
/**
* @return The unit type used for specifying a managed scaling policy. Valid Values: `InstanceFleetUnits` | `Instances` | `VCPU`
*
*/
public String unitType() {
return this.unitType;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ManagedScalingPolicyComputeLimit defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Integer maximumCapacityUnits;
private @Nullable Integer maximumCoreCapacityUnits;
private @Nullable Integer maximumOndemandCapacityUnits;
private Integer minimumCapacityUnits;
private String unitType;
public Builder() {}
public Builder(ManagedScalingPolicyComputeLimit defaults) {
Objects.requireNonNull(defaults);
this.maximumCapacityUnits = defaults.maximumCapacityUnits;
this.maximumCoreCapacityUnits = defaults.maximumCoreCapacityUnits;
this.maximumOndemandCapacityUnits = defaults.maximumOndemandCapacityUnits;
this.minimumCapacityUnits = defaults.minimumCapacityUnits;
this.unitType = defaults.unitType;
}
@CustomType.Setter
public Builder maximumCapacityUnits(Integer maximumCapacityUnits) {
if (maximumCapacityUnits == null) {
throw new MissingRequiredPropertyException("ManagedScalingPolicyComputeLimit", "maximumCapacityUnits");
}
this.maximumCapacityUnits = maximumCapacityUnits;
return this;
}
@CustomType.Setter
public Builder maximumCoreCapacityUnits(@Nullable Integer maximumCoreCapacityUnits) {
this.maximumCoreCapacityUnits = maximumCoreCapacityUnits;
return this;
}
@CustomType.Setter
public Builder maximumOndemandCapacityUnits(@Nullable Integer maximumOndemandCapacityUnits) {
this.maximumOndemandCapacityUnits = maximumOndemandCapacityUnits;
return this;
}
@CustomType.Setter
public Builder minimumCapacityUnits(Integer minimumCapacityUnits) {
if (minimumCapacityUnits == null) {
throw new MissingRequiredPropertyException("ManagedScalingPolicyComputeLimit", "minimumCapacityUnits");
}
this.minimumCapacityUnits = minimumCapacityUnits;
return this;
}
@CustomType.Setter
public Builder unitType(String unitType) {
if (unitType == null) {
throw new MissingRequiredPropertyException("ManagedScalingPolicyComputeLimit", "unitType");
}
this.unitType = unitType;
return this;
}
public ManagedScalingPolicyComputeLimit build() {
final var _resultValue = new ManagedScalingPolicyComputeLimit();
_resultValue.maximumCapacityUnits = maximumCapacityUnits;
_resultValue.maximumCoreCapacityUnits = maximumCoreCapacityUnits;
_resultValue.maximumOndemandCapacityUnits = maximumOndemandCapacityUnits;
_resultValue.minimumCapacityUnits = minimumCapacityUnits;
_resultValue.unitType = unitType;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy