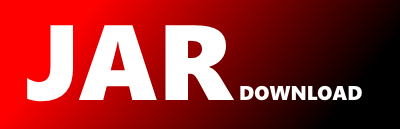
com.pulumi.aws.emrserverless.ApplicationArgs Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.emrserverless;
import com.pulumi.aws.emrserverless.inputs.ApplicationAutoStartConfigurationArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationAutoStopConfigurationArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationImageConfigurationArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationInitialCapacityArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationInteractiveConfigurationArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationMaximumCapacityArgs;
import com.pulumi.aws.emrserverless.inputs.ApplicationNetworkConfigurationArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ApplicationArgs extends com.pulumi.resources.ResourceArgs {
public static final ApplicationArgs Empty = new ApplicationArgs();
/**
* The CPU architecture of an application. Valid values are `ARM64` or `X86_64`. Default value is `X86_64`.
*
*/
@Import(name="architecture")
private @Nullable Output architecture;
/**
* @return The CPU architecture of an application. Valid values are `ARM64` or `X86_64`. Default value is `X86_64`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy