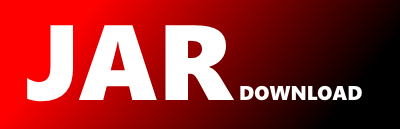
com.pulumi.aws.fsx.OntapStorageVirtualMachine Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.fsx;
import com.pulumi.aws.Utilities;
import com.pulumi.aws.fsx.OntapStorageVirtualMachineArgs;
import com.pulumi.aws.fsx.inputs.OntapStorageVirtualMachineState;
import com.pulumi.aws.fsx.outputs.OntapStorageVirtualMachineActiveDirectoryConfiguration;
import com.pulumi.aws.fsx.outputs.OntapStorageVirtualMachineEndpoint;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a FSx Storage Virtual Machine.
* See the [FSx ONTAP User Guide](https://docs.aws.amazon.com/fsx/latest/ONTAPGuide/managing-svms.html) for more information.
*
* ## Example Usage
*
* ### Basic Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.fsx.OntapStorageVirtualMachine;
* import com.pulumi.aws.fsx.OntapStorageVirtualMachineArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new OntapStorageVirtualMachine("test", OntapStorageVirtualMachineArgs.builder()
* .fileSystemId(testAwsFsxOntapFileSystem.id())
* .name("test")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ### Using a Self-Managed Microsoft Active Directory
*
* Additional information for using AWS Directory Service with ONTAP File Systems can be found in the [FSx ONTAP Guide](https://docs.aws.amazon.com/fsx/latest/ONTAPGuide/self-managed-AD.html).
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.aws.fsx.OntapStorageVirtualMachine;
* import com.pulumi.aws.fsx.OntapStorageVirtualMachineArgs;
* import com.pulumi.aws.fsx.inputs.OntapStorageVirtualMachineActiveDirectoryConfigurationArgs;
* import com.pulumi.aws.fsx.inputs.OntapStorageVirtualMachineActiveDirectoryConfigurationSelfManagedActiveDirectoryConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var test = new OntapStorageVirtualMachine("test", OntapStorageVirtualMachineArgs.builder()
* .fileSystemId(testAwsFsxOntapFileSystem.id())
* .name("mysvm")
* .activeDirectoryConfiguration(OntapStorageVirtualMachineActiveDirectoryConfigurationArgs.builder()
* .netbiosName("mysvm")
* .selfManagedActiveDirectoryConfiguration(OntapStorageVirtualMachineActiveDirectoryConfigurationSelfManagedActiveDirectoryConfigurationArgs.builder()
* .dnsIps(
* "10.0.0.111",
* "10.0.0.222")
* .domainName("corp.example.com")
* .password("avoid-plaintext-passwords")
* .username("Admin")
* .build())
* .build())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Using `pulumi import`, import FSx Storage Virtual Machine using the `id`. For example:
*
* ```sh
* $ pulumi import aws:fsx/ontapStorageVirtualMachine:OntapStorageVirtualMachine example svm-12345678abcdef123
* ```
* Certain resource arguments, like `svm_admin_password` and the `self_managed_active_directory` configuation block `password`, do not have a FSx API method for reading the information after creation. If these arguments are set in the Pulumi program on an imported resource, Pulumi will always show a difference. To workaround this behavior, either omit the argument from the Pulumi program or use `ignore_changes` to hide the difference. For example:
*
*/
@ResourceType(type="aws:fsx/ontapStorageVirtualMachine:OntapStorageVirtualMachine")
public class OntapStorageVirtualMachine extends com.pulumi.resources.CustomResource {
/**
* Configuration block that Amazon FSx uses to join the FSx ONTAP Storage Virtual Machine(SVM) to your Microsoft Active Directory (AD) directory. Detailed below.
*
*/
@Export(name="activeDirectoryConfiguration", refs={OntapStorageVirtualMachineActiveDirectoryConfiguration.class}, tree="[0]")
private Output* @Nullable */ OntapStorageVirtualMachineActiveDirectoryConfiguration> activeDirectoryConfiguration;
/**
* @return Configuration block that Amazon FSx uses to join the FSx ONTAP Storage Virtual Machine(SVM) to your Microsoft Active Directory (AD) directory. Detailed below.
*
*/
public Output> activeDirectoryConfiguration() {
return Codegen.optional(this.activeDirectoryConfiguration);
}
/**
* Amazon Resource Name of the storage virtual machine.
*
*/
@Export(name="arn", refs={String.class}, tree="[0]")
private Output arn;
/**
* @return Amazon Resource Name of the storage virtual machine.
*
*/
public Output arn() {
return this.arn;
}
/**
* The endpoints that are used to access data or to manage the storage virtual machine using the NetApp ONTAP CLI, REST API, or NetApp SnapMirror. See Endpoints below.
*
*/
@Export(name="endpoints", refs={List.class,OntapStorageVirtualMachineEndpoint.class}, tree="[0,1]")
private Output> endpoints;
/**
* @return The endpoints that are used to access data or to manage the storage virtual machine using the NetApp ONTAP CLI, REST API, or NetApp SnapMirror. See Endpoints below.
*
*/
public Output> endpoints() {
return this.endpoints;
}
/**
* The ID of the Amazon FSx ONTAP File System that this SVM will be created on.
*
*/
@Export(name="fileSystemId", refs={String.class}, tree="[0]")
private Output fileSystemId;
/**
* @return The ID of the Amazon FSx ONTAP File System that this SVM will be created on.
*
*/
public Output fileSystemId() {
return this.fileSystemId;
}
/**
* The name of the SVM. You can use a maximum of 47 alphanumeric characters, plus the underscore (_) special character.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the SVM. You can use a maximum of 47 alphanumeric characters, plus the underscore (_) special character.
*
*/
public Output name() {
return this.name;
}
/**
* Specifies the root volume security style, Valid values are `UNIX`, `NTFS`, and `MIXED`. All volumes created under this SVM will inherit the root security style unless the security style is specified on the volume. Default value is `UNIX`.
*
*/
@Export(name="rootVolumeSecurityStyle", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> rootVolumeSecurityStyle;
/**
* @return Specifies the root volume security style, Valid values are `UNIX`, `NTFS`, and `MIXED`. All volumes created under this SVM will inherit the root security style unless the security style is specified on the volume. Default value is `UNIX`.
*
*/
public Output> rootVolumeSecurityStyle() {
return Codegen.optional(this.rootVolumeSecurityStyle);
}
/**
* Describes the SVM's subtype, e.g. `DEFAULT`
*
*/
@Export(name="subtype", refs={String.class}, tree="[0]")
private Output subtype;
/**
* @return Describes the SVM's subtype, e.g. `DEFAULT`
*
*/
public Output subtype() {
return this.subtype;
}
/**
* Specifies the password to use when logging on to the SVM using a secure shell (SSH) connection to the SVM's management endpoint. Doing so enables you to manage the SVM using the NetApp ONTAP CLI or REST API. If you do not specify a password, you can still use the file system's fsxadmin user to manage the SVM.
*
*/
@Export(name="svmAdminPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> svmAdminPassword;
/**
* @return Specifies the password to use when logging on to the SVM using a secure shell (SSH) connection to the SVM's management endpoint. Doing so enables you to manage the SVM using the NetApp ONTAP CLI or REST API. If you do not specify a password, you can still use the file system's fsxadmin user to manage the SVM.
*
*/
public Output> svmAdminPassword() {
return Codegen.optional(this.svmAdminPassword);
}
/**
* A map of tags to assign to the storage virtual machine. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A map of tags to assign to the storage virtual machine. If configured with a provider `default_tags` configuration block present, tags with matching keys will overwrite those defined at the provider-level.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A map of tags assigned to the resource, including those inherited from the provider `default_tags` configuration block.
*
* @deprecated
* Please use `tags` instead.
*
*/
@Deprecated /* Please use `tags` instead. */
@Export(name="tagsAll", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy