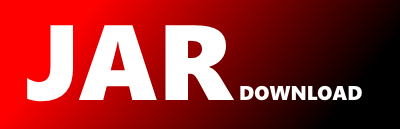
com.pulumi.aws.fsx.outputs.OpenZfsFileSystemRootVolumeConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.fsx.outputs;
import com.pulumi.aws.fsx.outputs.OpenZfsFileSystemRootVolumeConfigurationNfsExports;
import com.pulumi.aws.fsx.outputs.OpenZfsFileSystemRootVolumeConfigurationUserAndGroupQuota;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class OpenZfsFileSystemRootVolumeConfiguration {
/**
* @return A boolean flag indicating whether tags for the file system should be copied to snapshots. The default value is false.
*
*/
private @Nullable Boolean copyTagsToSnapshots;
/**
* @return Method used to compress the data on the volume. Valid values are `LZ4`, `NONE` or `ZSTD`. Child volumes that don't specify compression option will inherit from parent volume. This option on file system applies to the root volume.
*
*/
private @Nullable String dataCompressionType;
/**
* @return NFS export configuration for the root volume. Exactly 1 item. See `nfs_exports` Block for details.
*
*/
private @Nullable OpenZfsFileSystemRootVolumeConfigurationNfsExports nfsExports;
/**
* @return specifies whether the volume is read-only. Default is false.
*
*/
private @Nullable Boolean readOnly;
/**
* @return Specifies the record size of an OpenZFS root volume, in kibibytes (KiB). Valid values are `4`, `8`, `16`, `32`, `64`, `128`, `256`, `512`, or `1024` KiB. The default is `128` KiB.
*
*/
private @Nullable Integer recordSizeKib;
/**
* @return Specify how much storage users or groups can use on the volume. Maximum of 100 items. See `user_and_group_quotas` Block for details.
*
*/
private @Nullable List userAndGroupQuotas;
private OpenZfsFileSystemRootVolumeConfiguration() {}
/**
* @return A boolean flag indicating whether tags for the file system should be copied to snapshots. The default value is false.
*
*/
public Optional copyTagsToSnapshots() {
return Optional.ofNullable(this.copyTagsToSnapshots);
}
/**
* @return Method used to compress the data on the volume. Valid values are `LZ4`, `NONE` or `ZSTD`. Child volumes that don't specify compression option will inherit from parent volume. This option on file system applies to the root volume.
*
*/
public Optional dataCompressionType() {
return Optional.ofNullable(this.dataCompressionType);
}
/**
* @return NFS export configuration for the root volume. Exactly 1 item. See `nfs_exports` Block for details.
*
*/
public Optional nfsExports() {
return Optional.ofNullable(this.nfsExports);
}
/**
* @return specifies whether the volume is read-only. Default is false.
*
*/
public Optional readOnly() {
return Optional.ofNullable(this.readOnly);
}
/**
* @return Specifies the record size of an OpenZFS root volume, in kibibytes (KiB). Valid values are `4`, `8`, `16`, `32`, `64`, `128`, `256`, `512`, or `1024` KiB. The default is `128` KiB.
*
*/
public Optional recordSizeKib() {
return Optional.ofNullable(this.recordSizeKib);
}
/**
* @return Specify how much storage users or groups can use on the volume. Maximum of 100 items. See `user_and_group_quotas` Block for details.
*
*/
public List userAndGroupQuotas() {
return this.userAndGroupQuotas == null ? List.of() : this.userAndGroupQuotas;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(OpenZfsFileSystemRootVolumeConfiguration defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean copyTagsToSnapshots;
private @Nullable String dataCompressionType;
private @Nullable OpenZfsFileSystemRootVolumeConfigurationNfsExports nfsExports;
private @Nullable Boolean readOnly;
private @Nullable Integer recordSizeKib;
private @Nullable List userAndGroupQuotas;
public Builder() {}
public Builder(OpenZfsFileSystemRootVolumeConfiguration defaults) {
Objects.requireNonNull(defaults);
this.copyTagsToSnapshots = defaults.copyTagsToSnapshots;
this.dataCompressionType = defaults.dataCompressionType;
this.nfsExports = defaults.nfsExports;
this.readOnly = defaults.readOnly;
this.recordSizeKib = defaults.recordSizeKib;
this.userAndGroupQuotas = defaults.userAndGroupQuotas;
}
@CustomType.Setter
public Builder copyTagsToSnapshots(@Nullable Boolean copyTagsToSnapshots) {
this.copyTagsToSnapshots = copyTagsToSnapshots;
return this;
}
@CustomType.Setter
public Builder dataCompressionType(@Nullable String dataCompressionType) {
this.dataCompressionType = dataCompressionType;
return this;
}
@CustomType.Setter
public Builder nfsExports(@Nullable OpenZfsFileSystemRootVolumeConfigurationNfsExports nfsExports) {
this.nfsExports = nfsExports;
return this;
}
@CustomType.Setter
public Builder readOnly(@Nullable Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
@CustomType.Setter
public Builder recordSizeKib(@Nullable Integer recordSizeKib) {
this.recordSizeKib = recordSizeKib;
return this;
}
@CustomType.Setter
public Builder userAndGroupQuotas(@Nullable List userAndGroupQuotas) {
this.userAndGroupQuotas = userAndGroupQuotas;
return this;
}
public Builder userAndGroupQuotas(OpenZfsFileSystemRootVolumeConfigurationUserAndGroupQuota... userAndGroupQuotas) {
return userAndGroupQuotas(List.of(userAndGroupQuotas));
}
public OpenZfsFileSystemRootVolumeConfiguration build() {
final var _resultValue = new OpenZfsFileSystemRootVolumeConfiguration();
_resultValue.copyTagsToSnapshots = copyTagsToSnapshots;
_resultValue.dataCompressionType = dataCompressionType;
_resultValue.nfsExports = nfsExports;
_resultValue.readOnly = readOnly;
_resultValue.recordSizeKib = recordSizeKib;
_resultValue.userAndGroupQuotas = userAndGroupQuotas;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy