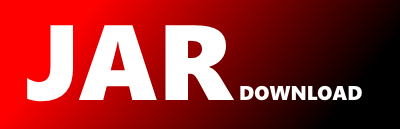
com.pulumi.aws.globalaccelerator.outputs.GetAcceleratorResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.globalaccelerator.outputs;
import com.pulumi.aws.globalaccelerator.outputs.GetAcceleratorAttribute;
import com.pulumi.aws.globalaccelerator.outputs.GetAcceleratorIpSet;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetAcceleratorResult {
private String arn;
private List attributes;
private String dnsName;
private String dualStackDnsName;
private Boolean enabled;
private String hostedZoneId;
private String id;
private String ipAddressType;
private List ipSets;
private String name;
private Map tags;
private GetAcceleratorResult() {}
public String arn() {
return this.arn;
}
public List attributes() {
return this.attributes;
}
public String dnsName() {
return this.dnsName;
}
public String dualStackDnsName() {
return this.dualStackDnsName;
}
public Boolean enabled() {
return this.enabled;
}
public String hostedZoneId() {
return this.hostedZoneId;
}
public String id() {
return this.id;
}
public String ipAddressType() {
return this.ipAddressType;
}
public List ipSets() {
return this.ipSets;
}
public String name() {
return this.name;
}
public Map tags() {
return this.tags;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetAcceleratorResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String arn;
private List attributes;
private String dnsName;
private String dualStackDnsName;
private Boolean enabled;
private String hostedZoneId;
private String id;
private String ipAddressType;
private List ipSets;
private String name;
private Map tags;
public Builder() {}
public Builder(GetAcceleratorResult defaults) {
Objects.requireNonNull(defaults);
this.arn = defaults.arn;
this.attributes = defaults.attributes;
this.dnsName = defaults.dnsName;
this.dualStackDnsName = defaults.dualStackDnsName;
this.enabled = defaults.enabled;
this.hostedZoneId = defaults.hostedZoneId;
this.id = defaults.id;
this.ipAddressType = defaults.ipAddressType;
this.ipSets = defaults.ipSets;
this.name = defaults.name;
this.tags = defaults.tags;
}
@CustomType.Setter
public Builder arn(String arn) {
if (arn == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "arn");
}
this.arn = arn;
return this;
}
@CustomType.Setter
public Builder attributes(List attributes) {
if (attributes == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "attributes");
}
this.attributes = attributes;
return this;
}
public Builder attributes(GetAcceleratorAttribute... attributes) {
return attributes(List.of(attributes));
}
@CustomType.Setter
public Builder dnsName(String dnsName) {
if (dnsName == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "dnsName");
}
this.dnsName = dnsName;
return this;
}
@CustomType.Setter
public Builder dualStackDnsName(String dualStackDnsName) {
if (dualStackDnsName == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "dualStackDnsName");
}
this.dualStackDnsName = dualStackDnsName;
return this;
}
@CustomType.Setter
public Builder enabled(Boolean enabled) {
if (enabled == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "enabled");
}
this.enabled = enabled;
return this;
}
@CustomType.Setter
public Builder hostedZoneId(String hostedZoneId) {
if (hostedZoneId == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "hostedZoneId");
}
this.hostedZoneId = hostedZoneId;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipAddressType(String ipAddressType) {
if (ipAddressType == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "ipAddressType");
}
this.ipAddressType = ipAddressType;
return this;
}
@CustomType.Setter
public Builder ipSets(List ipSets) {
if (ipSets == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "ipSets");
}
this.ipSets = ipSets;
return this;
}
public Builder ipSets(GetAcceleratorIpSet... ipSets) {
return ipSets(List.of(ipSets));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetAcceleratorResult", "tags");
}
this.tags = tags;
return this;
}
public GetAcceleratorResult build() {
final var _resultValue = new GetAcceleratorResult();
_resultValue.arn = arn;
_resultValue.attributes = attributes;
_resultValue.dnsName = dnsName;
_resultValue.dualStackDnsName = dualStackDnsName;
_resultValue.enabled = enabled;
_resultValue.hostedZoneId = hostedZoneId;
_resultValue.id = id;
_resultValue.ipAddressType = ipAddressType;
_resultValue.ipSets = ipSets;
_resultValue.name = name;
_resultValue.tags = tags;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy