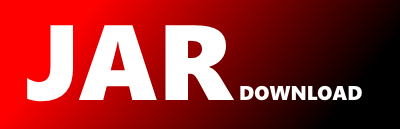
com.pulumi.aws.glue.outputs.GetScriptResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.glue.outputs;
import com.pulumi.aws.glue.outputs.GetScriptDagEdge;
import com.pulumi.aws.glue.outputs.GetScriptDagNode;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetScriptResult {
private List dagEdges;
private List dagNodes;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private @Nullable String language;
/**
* @return Python script generated from the DAG when the `language` argument is set to `PYTHON`.
*
*/
private String pythonScript;
/**
* @return Scala code generated from the DAG when the `language` argument is set to `SCALA`.
*
*/
private String scalaCode;
private GetScriptResult() {}
public List dagEdges() {
return this.dagEdges;
}
public List dagNodes() {
return this.dagNodes;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public Optional language() {
return Optional.ofNullable(this.language);
}
/**
* @return Python script generated from the DAG when the `language` argument is set to `PYTHON`.
*
*/
public String pythonScript() {
return this.pythonScript;
}
/**
* @return Scala code generated from the DAG when the `language` argument is set to `SCALA`.
*
*/
public String scalaCode() {
return this.scalaCode;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetScriptResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private List dagEdges;
private List dagNodes;
private String id;
private @Nullable String language;
private String pythonScript;
private String scalaCode;
public Builder() {}
public Builder(GetScriptResult defaults) {
Objects.requireNonNull(defaults);
this.dagEdges = defaults.dagEdges;
this.dagNodes = defaults.dagNodes;
this.id = defaults.id;
this.language = defaults.language;
this.pythonScript = defaults.pythonScript;
this.scalaCode = defaults.scalaCode;
}
@CustomType.Setter
public Builder dagEdges(List dagEdges) {
if (dagEdges == null) {
throw new MissingRequiredPropertyException("GetScriptResult", "dagEdges");
}
this.dagEdges = dagEdges;
return this;
}
public Builder dagEdges(GetScriptDagEdge... dagEdges) {
return dagEdges(List.of(dagEdges));
}
@CustomType.Setter
public Builder dagNodes(List dagNodes) {
if (dagNodes == null) {
throw new MissingRequiredPropertyException("GetScriptResult", "dagNodes");
}
this.dagNodes = dagNodes;
return this;
}
public Builder dagNodes(GetScriptDagNode... dagNodes) {
return dagNodes(List.of(dagNodes));
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetScriptResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder language(@Nullable String language) {
this.language = language;
return this;
}
@CustomType.Setter
public Builder pythonScript(String pythonScript) {
if (pythonScript == null) {
throw new MissingRequiredPropertyException("GetScriptResult", "pythonScript");
}
this.pythonScript = pythonScript;
return this;
}
@CustomType.Setter
public Builder scalaCode(String scalaCode) {
if (scalaCode == null) {
throw new MissingRequiredPropertyException("GetScriptResult", "scalaCode");
}
this.scalaCode = scalaCode;
return this;
}
public GetScriptResult build() {
final var _resultValue = new GetScriptResult();
_resultValue.dagEdges = dagEdges;
_resultValue.dagNodes = dagNodes;
_resultValue.id = id;
_resultValue.language = language;
_resultValue.pythonScript = pythonScript;
_resultValue.scalaCode = scalaCode;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy