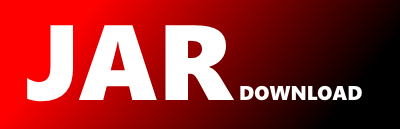
com.pulumi.aws.iam.ServiceSpecificCredentialArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws Show documentation
Show all versions of aws Show documentation
A Pulumi package for creating and managing Amazon Web Services (AWS) cloud resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.aws.iam;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ServiceSpecificCredentialArgs extends com.pulumi.resources.ResourceArgs {
public static final ServiceSpecificCredentialArgs Empty = new ServiceSpecificCredentialArgs();
/**
* The name of the AWS service that is to be associated with the credentials. The service you specify here is the only service that can be accessed using these credentials.
*
*/
@Import(name="serviceName", required=true)
private Output serviceName;
/**
* @return The name of the AWS service that is to be associated with the credentials. The service you specify here is the only service that can be accessed using these credentials.
*
*/
public Output serviceName() {
return this.serviceName;
}
/**
* The status to be assigned to the service-specific credential. Valid values are `Active` and `Inactive`. Default value is `Active`.
*
*/
@Import(name="status")
private @Nullable Output status;
/**
* @return The status to be assigned to the service-specific credential. Valid values are `Active` and `Inactive`. Default value is `Active`.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy